Routines to drive a chain of APA102 Leds.
Dependents: blink_led_threading
APA102.cpp
00001 #include "APA102.h" 00002 #include "mbed.h" 00003 00004 APA102::APA102(PinName mosi,PinName miso,PinName sclk,int rate) 00005 : _spi(mosi, miso, sclk) 00006 00007 { 00008 // Setup the spi for 8 bit data, high steady state clock, 00009 // second edge capture, with a 1MHz clock rate 00010 _spi.format(8,3); 00011 _spi.frequency(rate); 00012 } 00013 00014 void APA102::SetBuffer(unsigned int Buffer[],int Rows,int Cols, int Stride,int Offset, bool ZigZag,bool Wrap) 00015 { 00016 Buf = Buffer; 00017 NR = Rows; 00018 NC = Cols; 00019 NS = Stride; 00020 off = Offset; 00021 ZF = ZigZag; 00022 WF = Wrap; 00023 } 00024 00025 void APA102::Repaint() 00026 { 00027 int index; 00028 unsigned int val; 00029 00030 _spi.write(0X00); // Start 00031 _spi.write(0X00); 00032 _spi.write(0X00); 00033 _spi.write(0X00); 00034 00035 for(int r = 0;r<NR;r++) 00036 { 00037 for(int c = off;c<(NC+off);c++) 00038 { 00039 int cc = (WF)?(c%NS):((c<NS)?c:NS); 00040 if (ZF) 00041 if((r&0x01)>0) 00042 index = r*NS + NC+off-cc; 00043 else 00044 index = r*NS + cc; 00045 else 00046 index = r*NS + cc; 00047 00048 val = Buf[index]; 00049 _spi.write((val>>24)&0xFF); 00050 _spi.write((val>>16)&0xFF); 00051 _spi.write((val>>8)&0xFF); 00052 _spi.write(val&0xFF); 00053 } 00054 } 00055 _spi.write(0XFF); // Stop 00056 _spi.write(0XFF); 00057 _spi.write(0XFF); 00058 _spi.write(0XFF); 00059 00060 }
Generated on Sun Jul 17 2022 00:46:40 by
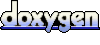