Library for 1.8 inch 160*180 TFT Display. Nice fonts in different formats.
Embed:
(wiki syntax)
Show/hide line numbers
ST7735.h
00001 #ifndef ST7735_H 00002 #define ST7735_H 00003 00004 #include "mbed.h" 00005 00006 // Comment out the #defines below with // to stop that font being loaded 00007 // If all fonts are loaded the total space required is ablout 17890 bytes 00008 00009 #define LOAD_FONT2 // Small font, needs ~3092 bytes in FLASH 00010 #define LOAD_FONT4 // Medium font, needs ~8126 bytes in FLASH 00011 #define LOAD_FONT6 // Large font, needs ~4404 bytes in FLASH 00012 #define LOAD_FONT7 // 7 segment font, needs ~3652 bytes in FLASH 00013 #define LOAD_FONT8 // Large font needs ~10kbytes, only 1234567890:. 00014 00015 #define pgm_read_byte(addr) (*(const unsigned char *)(addr)) 00016 #define pgm_read_word(addr) (*(const unsigned short *)(addr)) 00017 00018 #define swap(a, b) { int16_t t = a; a = b; b = t; } 00019 00020 #define INITR_GREENTAB 0x0 00021 #define INITR_REDTAB 0x1 00022 #define INITR_BLACKTAB 0x2 00023 00024 #define ST7735_TFTWIDTH 128 00025 #define ST7735_TFTHEIGHT 160 00026 00027 #define ST7735_NOP 0x00 00028 #define ST7735_SWRESET 0x01 00029 #define ST7735_RDDID 0x04 00030 #define ST7735_RDDST 0x09 00031 00032 #define ST7735_SLPIN 0x10 00033 #define ST7735_SLPOUT 0x11 00034 #define ST7735_PTLON 0x12 00035 #define ST7735_NORON 0x13 00036 00037 #define ST7735_INVOFF 0x20 00038 #define ST7735_INVON 0x21 00039 #define ST7735_DISPOFF 0x28 00040 #define ST7735_DISPON 0x29 00041 #define ST7735_CASET 0x2A 00042 #define ST7735_RASET 0x2B 00043 #define ST7735_RAMWR 0x2C 00044 #define ST7735_RAMRD 0x2E 00045 00046 #define ST7735_PTLAR 0x30 00047 #define ST7735_COLMOD 0x3A 00048 #define ST7735_MADCTL 0x36 00049 00050 #define ST7735_FRMCTR1 0xB1 00051 #define ST7735_FRMCTR2 0xB2 00052 #define ST7735_FRMCTR3 0xB3 00053 #define ST7735_INVCTR 0xB4 00054 #define ST7735_DISSET5 0xB6 00055 00056 #define ST7735_PWCTR1 0xC0 00057 #define ST7735_PWCTR2 0xC1 00058 #define ST7735_PWCTR3 0xC2 00059 #define ST7735_PWCTR4 0xC3 00060 #define ST7735_PWCTR5 0xC4 00061 #define ST7735_VMCTR1 0xC5 00062 00063 #define ST7735_RDID1 0xDA 00064 #define ST7735_RDID2 0xDB 00065 #define ST7735_RDID3 0xDC 00066 #define ST7735_RDID4 0xDD 00067 00068 #define ST7735_PWCTR6 0xFC 00069 00070 #define ST7735_GMCTRP1 0xE0 00071 #define ST7735_GMCTRN1 0xE1 00072 00073 // Color definitions 00074 #define ST7735_BLACK 0x0000 00075 #define ST7735_BLUE 0x001F 00076 #define ST7735_RED 0xF800 00077 #define ST7735_GREEN 0x07E0 00078 #define ST7735_CYAN 0x07FF 00079 #define ST7735_MAGENTA 0xF81F 00080 #define ST7735_YELLOW 0xFFE0 00081 #define ST7735_WHITE 0xFFFF 00082 #define ST7735_GREY 0x39C4 00083 #define ST7735_NAVY 0x000F 00084 #define ST7735_DARKGREEN 0x03E0 00085 #define ST7735_MAROON 0x7800 00086 #define ST7735_PURPLE 0x780F 00087 #define ST7735_OLIVE 0x7BE0 00088 #define ST7735_LIGHTGREY 0xC618 00089 #define ST7735_DARKGREY 0x7BEF 00090 #define ST7735_BROWN 0xFD20 00091 #define ST7735_PINK 0xF81F 00092 00093 #define MADCTL_MY 0x80 00094 #define MADCTL_MX 0x40 00095 #define MADCTL_MV 0x20 00096 #define MADCTL_ML 0x10 00097 #define MADCTL_RGB 0x00 00098 #define MADCTL_BGR 0x08 00099 #define MADCTL_MH 0x04 00100 00101 class ST7735 00102 { 00103 00104 public: 00105 /** Create a ST7735 object connected to the specified pins 00106 * 00107 * @param ce Pin connected to chip enable 00108 * @param dc Pin connected to data/command select 00109 * @param mosi Pin connected to data input 00110 * @param sclk Pin connected to serial clock 00111 * 00112 * Reset pin from LCD module is connected to reset pin microcontroller 00113 */ 00114 00115 ST7735(PinName cePin, PinName dcPin, PinName mosiPin, PinName sclkPin); 00116 00117 private: 00118 void writecommand(char c); 00119 void writedata(char c); 00120 void initSPI(); 00121 void commonInit(const uint8_t *cmdList); 00122 void commandList(const uint8_t *addr); 00123 void pushColor(uint16_t color); 00124 00125 public: 00126 uint8_t tabcolor; 00127 uint8_t colstart, rowstart; // some displays need this changed 00128 void initB(); 00129 void initR(uint8_t options); 00130 void setRotation(uint8_t m); 00131 void fillScreen(uint16_t color); 00132 void fillRect(int16_t x, int16_t y, int16_t w, int16_t h, uint16_t color); 00133 void setAddrWindow(uint8_t x0, uint8_t y0, uint8_t x1, uint8_t y1); 00134 void invertDisplay(bool i); 00135 void drawPixel(int16_t x, int16_t y, uint16_t color); 00136 void drawFastVLine(int16_t x, int16_t y, int16_t h, uint16_t color); 00137 void drawFastHLine(int16_t x, int16_t y, int16_t w, uint16_t color); 00138 uint16_t Color565(uint8_t r, uint8_t g, uint8_t b); 00139 void drawLine(int16_t x0, int16_t y0, int16_t x1, int16_t y1, uint16_t color); 00140 void drawRect(int16_t x, int16_t y, int16_t w, int16_t h, uint16_t color); 00141 void drawCircle(int16_t x0, int16_t y0, int16_t r, uint16_t color); 00142 void drawCircleHelper( int16_t x0, int16_t y0, int16_t r, uint8_t cornername, uint16_t color); 00143 void fillCircle(int16_t x0, int16_t y0, int16_t r, uint16_t color); 00144 void fillCircleHelper(int16_t x0, int16_t y0, int16_t r, uint8_t cornername, int16_t delta, uint16_t color); 00145 void drawTriangle(int16_t x0, int16_t y0, int16_t x1, int16_t y1, int16_t x2, int16_t y2, uint16_t color); 00146 void fillTriangle ( int16_t x0, int16_t y0, int16_t x1, int16_t y1, int16_t x2, int16_t y2, uint16_t color); 00147 void drawRoundRect(int16_t x, int16_t y, int16_t w, int16_t h, int16_t r, uint16_t color); 00148 void fillRoundRect(int16_t x, int16_t y, int16_t w, int16_t h, int16_t r, uint16_t color); 00149 void setCursor(int16_t x, int16_t y); 00150 void setTextSize(uint8_t s); 00151 void setTextColor(uint16_t c); 00152 void setTextColor(uint16_t c, uint16_t b); 00153 void setTextWrap(bool w); 00154 uint8_t getRotation(void); 00155 void drawBitmap(int16_t x, int16_t y, const uint8_t *bitmap, int16_t w, int16_t h, uint16_t color); 00156 int drawUnicode(unsigned int uniCode, int x, int y, int font); 00157 int drawNumber(long long_num,int poX, int poY, int font); 00158 int drawChar(char c, int x, int y, int font); 00159 int drawString(char *string, int poX, int poY, int font); 00160 int drawCentreString(char *string, int dX, int poY, int font); 00161 int drawRightString(char *string, int dX, int poY, int font); 00162 int drawFloat(float floatNumber, int decimal, int poX, int poY, int font); 00163 00164 00165 00166 private: 00167 SPI* spi; 00168 DigitalOut* ce; 00169 DigitalOut* rst; 00170 DigitalOut* dc; 00171 00172 protected: 00173 static const int16_t WIDTH = ST7735_TFTWIDTH; // This is the 'raw' display w/h - never changes 00174 static const int16_t HEIGHT = ST7735_TFTHEIGHT; 00175 int16_t _width, _height; // Display w/h as modified by current rotation 00176 uint16_t textcolor, textbgcolor; 00177 uint8_t textsize; 00178 uint8_t rotation; 00179 bool wrap; // If set, 'wrap' text at right edge of display 00180 int16_t cursor_x; 00181 int16_t cursor_y; 00182 }; 00183 00184 #endif
Generated on Wed Jul 13 2022 21:17:58 by
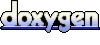