
Motor control on mbed
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 //Motor sensor 00004 InterruptIn HallA(D3); 00005 InterruptIn HallB(D4); 00006 InterruptIn HallC(D5); 00007 00008 void init_CN(void); 00009 void CN_interrupt(void); 00010 00011 int8_t stateA=0, stateB=0, stateC=0; 00012 int8_t state_1 = 0, state_1_old = 0; 00013 int vCount; 00014 00015 int main() { 00016 init_CN(); 00017 00018 while(1) 00019 { 00020 ; 00021 } 00022 } 00023 00024 void CN_interrupt(void) 00025 { 00026 //Motor 1 00027 stateA = HallA.read(); 00028 stateB = HallB.read(); 00029 stateC = HallC.read(); 00030 00031 ///code for state determination/// 00032 if (stateA == 1) 00033 { 00034 if (stateB == 1) 00035 if(stateC == 0) 00036 { 00037 state_1 = 2; 00038 } 00039 else 00040 { 00041 ; 00042 } 00043 else 00044 if(stateC == 1) 00045 { 00046 state_1 = 6; 00047 } 00048 else 00049 { 00050 state_1 = 1; 00051 } 00052 } 00053 else 00054 { 00055 if (stateB == 1) 00056 if(stateC == 1) 00057 { 00058 state_1 = 4; 00059 } 00060 else 00061 { 00062 state_1 = 3; 00063 } 00064 else 00065 if(stateC == 1) 00066 { 00067 state_1 = 5; 00068 } 00069 else 00070 { 00071 ; 00072 } 00073 } 00074 00075 //Forward: vCount +1 00076 //Inverse: vCount -1 00077 if ( (state_1 == (state_1_old + 1)) || (state_1 == 1 && state_1_old == 6) ) 00078 vCount++; 00079 else if ( (state_1 == (state_1_old - 1)) || (state_1 == 6 && state_1_old == 1)) 00080 vCount--; 00081 00082 state_1_old = state_1; 00083 } 00084 00085 void init_CN(void) 00086 { 00087 HallA.rise(&CN_interrupt); 00088 HallA.fall(&CN_interrupt); 00089 HallB.rise(&CN_interrupt); 00090 HallB.fall(&CN_interrupt); 00091 HallC.rise(&CN_interrupt); 00092 HallC.fall(&CN_interrupt); 00093 00094 stateA = HallA.read(); 00095 stateB = HallB.read(); 00096 stateC = HallC.read(); 00097 00098 }
Generated on Sun Jul 24 2022 04:24:34 by
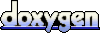