LT_SPI
Embed:
(wiki syntax)
Show/hide line numbers
LT_SPI.h
Go to the documentation of this file.
00001 //! @todo Review this document. 00002 /*! 00003 LT_SPI: Routines to communicate with ATmega328P's hardware SPI port. 00004 00005 REVISION HISTORY 00006 $Revision: 3659 $ 00007 $Date: 2015-07-01 10:19:20 -0700 (Wed, 01 Jul 2015) $ 00008 00009 Copyright (c) 2013, Linear Technology Corp.(LTC) 00010 All rights reserved. 00011 00012 Redistribution and use in source and binary forms, with or without 00013 modification, are permitted provided that the following conditions are met: 00014 00015 1. Redistributions of source code must retain the above copyright notice, this 00016 list of conditions and the following disclaimer. 00017 2. Redistributions in binary form must reproduce the above copyright notice, 00018 this list of conditions and the following disclaimer in the documentation 00019 and/or other materials provided with the distribution. 00020 00021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 00022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00024 DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR 00025 ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00026 (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00027 LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00028 ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00029 (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00030 SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00031 00032 The views and conclusions contained in the software and documentation are those 00033 of the authors and should not be interpreted as representing official policies, 00034 either expressed or implied, of Linear Technology Corp. 00035 00036 The Linear Technology Linduino is not affiliated with the official Arduino team. 00037 However, the Linduino is only possible because of the Arduino team's commitment 00038 to the open-source community. Please, visit http://www.arduino.cc and 00039 http://store.arduino.cc , and consider a purchase that will help fund their 00040 ongoing work. 00041 */ 00042 00043 /*! @file 00044 @ingroup LT_SPI 00045 Library Header File for LT_SPI: Routines to communicate with ATmega328P's hardware SPI port. 00046 */ 00047 00048 #ifndef LT_SPI_H 00049 #define LT_SPI_H 00050 00051 #include "mbed.h" 00052 00053 SPI spi(A6,A5,A4,A3); 00054 // Uncomment the following to use functions that implement LTC SPI routines 00055 00056 // //! @name SPI CLOCK DIVIDER CONSTANTS 00057 // //! @{ 00058 // #define SPI_CLOCK_DIV4 0x00 // 4 Mhz 00059 // #define SPI_CLOCK_DIV16 0x01 // 1 Mhz 00060 // #define SPI_CLOCK_DIV64 0x02 // 250 khz 00061 // #define SPI_CLOCK_DIV128 0x03 // 125 khz 00062 // #define SPI_CLOCK_DIV2 0x04 // 8 Mhz 00063 // #define SPI_CLOCK_DIV8 0x05 // 2 Mhz 00064 // #define SPI_CLOCK_DIV32 0x06 // 500 khz 00065 // //! @} 00066 // 00067 // //! @name SPI HARDWARE MODE CONSTANTS 00068 // //! @{ 00069 // #define SPI_MODE0 0x00 00070 // #define SPI_MODE1 0x04 00071 // // #define SPI_MODE2 0x08 00072 // #define SPI_MODE3 0x0C 00073 // //! @} 00074 // 00075 // //! @name SPI SET MASKS 00076 //! @{ 00077 // #define SPI_MODE_MASK 0x0C // CPOL = bit 3, CPHA = bit 2 on SPCR 00078 // #define SPI_CLOCK_MASK 0x03 // SPR1 = bit 1, SPR0 = bit 0 on SPCR 00079 // #define SPI_2XCLOCK_MASK 0x01 // SPI2X = bit 0 on SPSR 00080 // //! @} 00081 00082 //! Reads and sends a byte 00083 //! @return void 00084 void spi_transfer_byte(uint8_t cs_pin, //!< Chip select pin 00085 uint8_t tx, //!< Byte to be transmitted 00086 uint8_t *rx //!< Byte to be received 00087 ); 00088 00089 //! Reads and sends a word 00090 //! @return void 00091 void spi_transfer_word(uint8_t cs_pin, //!< Chip select pin 00092 uint16_t tx, //!< Byte to be transmitted 00093 uint16_t *rx //!< Byte to be received 00094 ); 00095 00096 //! Reads and sends a byte array 00097 //! @return void 00098 void spi_transfer_block(uint8_t cs_pin, //!< Chip select pin 00099 uint8_t *tx, //!< Byte array to be transmitted 00100 uint8_t *rx, //!< Byte array to be received 00101 uint8_t length //!< Length of array 00102 ); 00103 00104 //! Connect SPI pins to QuikEval connector through the Linduino MUX. This will disconnect I2C. 00105 //void quikeval_SPI_connect(); 00106 00107 //! Configure the SPI port for 4Mhz SCK. 00108 //! This function or spi_enable() must be called 00109 //! before using the other SPI routines. 00110 //void quikeval_SPI_init(); 00111 00112 //! Setup the processor for hardware SPI communication. 00113 //! Must be called before using the other SPI routines. 00114 //! Alternatively, call quikeval_SPI_connect(), which automatically 00115 //! calls this function. 00116 void spi_enable(void); //!< Configures SCK frequency. Use constant defined in header file. 00117 00118 00119 //! Disable the SPI hardware port 00120 //void spi_disable(); 00121 00122 //! Write a data byte using the SPI hardware 00123 //void spi_write(int8_t data //!< Byte to be written to SPI port 00124 // ); 00125 00126 //! Read and write a data byte using the SPI hardware 00127 //! @return the data byte read 00128 //int8_t spi_read(int8_t data //!< The data byte to be written 00129 // ); 00130 00131 #endif // LT_SPI_H
Generated on Sun Jul 17 2022 07:21:55 by
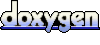