
by Rob Toulson and Tim Wilmshurst from textbook "Fast and Effective Embedded Systems Design: Applying the ARM mbed"
main.cpp
00001 /* Program Example 12.12 Using RPC variables for remote mbed control 00002 */ 00003 #include "mbed.h" 00004 #include "EthernetNetIf.h" 00005 #include "HTTPServer.h" 00006 #include "RPCVariable.h" 00007 #include "SerialRPCInterface.h" 00008 LocalFileSystem fs("webfs"); 00009 EthernetNetIf eth( 00010 IpAddr(192,168,0,101),//IP Address 00011 IpAddr(255,255,255,0),//Network Mask 00012 IpAddr(192,168,0,1), //Gateway 00013 IpAddr(192,168,0,1) //DNS 00014 ); 00015 HTTPServer svr; 00016 DigitalOut Led1(LED1); // define mbed object 00017 DigitalIn Button1(p21); // button 00018 int RemoteLEDStatus=0; 00019 RPCVariable<int> RPC_RemoteLEDStatus(&RemoteLEDStatus,"RemoteLEDStatus"); 00020 int RemoteLED1Button=0; 00021 RPCVariable<int> RPC_RemoteLED1Button(&RemoteLED1Button,"RemoteLED1Button"); 00022 int main() { 00023 Base::add_rpc_class<DigitalOut>(); // RPC base command 00024 eth.setup(); // Ethernet setup 00025 FSHandler::mount("/webfs", "/"); // Mount /webfs path root path 00026 svr.addHandler<FSHandler>("/"); //Default handler 00027 svr.addHandler<RPCHandler>("/rpc"); // Define RPC handler 00028 svr.bind(80); 00029 printf("Listening...\n"); 00030 while (true) { 00031 Net::poll(); 00032 if ((Button1==1)|(RemoteLED1Button==1)) { 00033 Led1=1; 00034 RemoteLEDStatus=1; 00035 } else { 00036 Led1=0; 00037 RemoteLEDStatus=0; 00038 } 00039 } 00040 } 00041
Generated on Sat Jul 30 2022 01:07:49 by
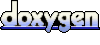