Aded CMSIS5 DSP and NN folder. Needs some work
Embed:
(wiki syntax)
Show/hide line numbers
mpu_armv7.h
00001 /****************************************************************************** 00002 * @file mpu_armv7.h 00003 * @brief CMSIS MPU API for Armv7-M MPU 00004 * @version V5.0.4 00005 * @date 10. January 2018 00006 ******************************************************************************/ 00007 /* 00008 * Copyright (c) 2017-2018 Arm Limited. All rights reserved. 00009 * 00010 * SPDX-License-Identifier: Apache-2.0 00011 * 00012 * Licensed under the Apache License, Version 2.0 (the License); you may 00013 * not use this file except in compliance with the License. 00014 * You may obtain a copy of the License at 00015 * 00016 * www.apache.org/licenses/LICENSE-2.0 00017 * 00018 * Unless required by applicable law or agreed to in writing, software 00019 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00020 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00021 * See the License for the specific language governing permissions and 00022 * limitations under the License. 00023 */ 00024 00025 #if defined ( __ICCARM__ ) 00026 #pragma system_include /* treat file as system include file for MISRA check */ 00027 #elif defined (__clang__) 00028 #pragma clang system_header /* treat file as system include file */ 00029 #endif 00030 00031 #ifndef ARM_MPU_ARMV7_H 00032 #define ARM_MPU_ARMV7_H 00033 00034 #define ARM_MPU_REGION_SIZE_32B ((uint8_t)0x04U) 00035 #define ARM_MPU_REGION_SIZE_64B ((uint8_t)0x05U) 00036 #define ARM_MPU_REGION_SIZE_128B ((uint8_t)0x06U) 00037 #define ARM_MPU_REGION_SIZE_256B ((uint8_t)0x07U) 00038 #define ARM_MPU_REGION_SIZE_512B ((uint8_t)0x08U) 00039 #define ARM_MPU_REGION_SIZE_1KB ((uint8_t)0x09U) 00040 #define ARM_MPU_REGION_SIZE_2KB ((uint8_t)0x0AU) 00041 #define ARM_MPU_REGION_SIZE_4KB ((uint8_t)0x0BU) 00042 #define ARM_MPU_REGION_SIZE_8KB ((uint8_t)0x0CU) 00043 #define ARM_MPU_REGION_SIZE_16KB ((uint8_t)0x0DU) 00044 #define ARM_MPU_REGION_SIZE_32KB ((uint8_t)0x0EU) 00045 #define ARM_MPU_REGION_SIZE_64KB ((uint8_t)0x0FU) 00046 #define ARM_MPU_REGION_SIZE_128KB ((uint8_t)0x10U) 00047 #define ARM_MPU_REGION_SIZE_256KB ((uint8_t)0x11U) 00048 #define ARM_MPU_REGION_SIZE_512KB ((uint8_t)0x12U) 00049 #define ARM_MPU_REGION_SIZE_1MB ((uint8_t)0x13U) 00050 #define ARM_MPU_REGION_SIZE_2MB ((uint8_t)0x14U) 00051 #define ARM_MPU_REGION_SIZE_4MB ((uint8_t)0x15U) 00052 #define ARM_MPU_REGION_SIZE_8MB ((uint8_t)0x16U) 00053 #define ARM_MPU_REGION_SIZE_16MB ((uint8_t)0x17U) 00054 #define ARM_MPU_REGION_SIZE_32MB ((uint8_t)0x18U) 00055 #define ARM_MPU_REGION_SIZE_64MB ((uint8_t)0x19U) 00056 #define ARM_MPU_REGION_SIZE_128MB ((uint8_t)0x1AU) 00057 #define ARM_MPU_REGION_SIZE_256MB ((uint8_t)0x1BU) 00058 #define ARM_MPU_REGION_SIZE_512MB ((uint8_t)0x1CU) 00059 #define ARM_MPU_REGION_SIZE_1GB ((uint8_t)0x1DU) 00060 #define ARM_MPU_REGION_SIZE_2GB ((uint8_t)0x1EU) 00061 #define ARM_MPU_REGION_SIZE_4GB ((uint8_t)0x1FU) 00062 00063 #define ARM_MPU_AP_NONE 0U 00064 #define ARM_MPU_AP_PRIV 1U 00065 #define ARM_MPU_AP_URO 2U 00066 #define ARM_MPU_AP_FULL 3U 00067 #define ARM_MPU_AP_PRO 5U 00068 #define ARM_MPU_AP_RO 6U 00069 00070 /** MPU Region Base Address Register Value 00071 * 00072 * \param Region The region to be configured, number 0 to 15. 00073 * \param BaseAddress The base address for the region. 00074 */ 00075 #define ARM_MPU_RBAR(Region, BaseAddress) \ 00076 (((BaseAddress) & MPU_RBAR_ADDR_Msk) | \ 00077 ((Region) & MPU_RBAR_REGION_Msk) | \ 00078 (MPU_RBAR_VALID_Msk)) 00079 00080 /** 00081 * MPU Region Attribute and Size Register Value 00082 * 00083 * \param DisableExec Instruction access disable bit, 1= disable instruction fetches. 00084 * \param AccessPermission Data access permissions, allows you to configure read/write access for User and Privileged mode. 00085 * \param TypeExtField Type extension field, allows you to configure memory access type, for example strongly ordered, peripheral. 00086 * \param IsShareable Region is shareable between multiple bus masters. 00087 * \param IsCacheable Region is cacheable, i.e. its value may be kept in cache. 00088 * \param IsBufferable Region is bufferable, i.e. using write-back caching. Cacheable but non-bufferable regions use write-through policy. 00089 * \param SubRegionDisable Sub-region disable field. 00090 * \param Size Region size of the region to be configured, for example 4K, 8K. 00091 */ 00092 #define ARM_MPU_RASR(DisableExec, AccessPermission, TypeExtField, IsShareable, IsCacheable, IsBufferable, SubRegionDisable, Size) \ 00093 ((((DisableExec ) << MPU_RASR_XN_Pos) & MPU_RASR_XN_Msk) | \ 00094 (((AccessPermission) << MPU_RASR_AP_Pos) & MPU_RASR_AP_Msk) | \ 00095 (((TypeExtField ) << MPU_RASR_TEX_Pos) & MPU_RASR_TEX_Msk) | \ 00096 (((IsShareable ) << MPU_RASR_S_Pos) & MPU_RASR_S_Msk) | \ 00097 (((IsCacheable ) << MPU_RASR_C_Pos) & MPU_RASR_C_Msk) | \ 00098 (((IsBufferable ) << MPU_RASR_B_Pos) & MPU_RASR_B_Msk) | \ 00099 (((SubRegionDisable) << MPU_RASR_SRD_Pos) & MPU_RASR_SRD_Msk) | \ 00100 (((Size ) << MPU_RASR_SIZE_Pos) & MPU_RASR_SIZE_Msk) | \ 00101 (MPU_RASR_ENABLE_Msk)) 00102 00103 00104 /** 00105 * Struct for a single MPU Region 00106 */ 00107 typedef struct { 00108 uint32_t RBAR; //!< The region base address register value (RBAR) 00109 uint32_t RASR; //!< The region attribute and size register value (RASR) \ref MPU_RASR 00110 } ARM_MPU_Region_t; 00111 00112 /** Enable the MPU. 00113 * \param MPU_Control Default access permissions for unconfigured regions. 00114 */ 00115 __STATIC_INLINE void ARM_MPU_Enable(uint32_t MPU_Control) 00116 { 00117 __DSB(); 00118 __ISB(); 00119 MPU->CTRL = MPU_Control | MPU_CTRL_ENABLE_Msk; 00120 #ifdef SCB_SHCSR_MEMFAULTENA_Msk 00121 SCB->SHCSR |= SCB_SHCSR_MEMFAULTENA_Msk; 00122 #endif 00123 } 00124 00125 /** Disable the MPU. 00126 */ 00127 __STATIC_INLINE void ARM_MPU_Disable(void) 00128 { 00129 __DSB(); 00130 __ISB(); 00131 #ifdef SCB_SHCSR_MEMFAULTENA_Msk 00132 SCB->SHCSR &= ~SCB_SHCSR_MEMFAULTENA_Msk; 00133 #endif 00134 MPU->CTRL &= ~MPU_CTRL_ENABLE_Msk; 00135 } 00136 00137 /** Clear and disable the given MPU region. 00138 * \param rnr Region number to be cleared. 00139 */ 00140 __STATIC_INLINE void ARM_MPU_ClrRegion(uint32_t rnr) 00141 { 00142 MPU->RNR = rnr; 00143 MPU->RASR = 0U; 00144 } 00145 00146 /** Configure an MPU region. 00147 * \param rbar Value for RBAR register. 00148 * \param rsar Value for RSAR register. 00149 */ 00150 __STATIC_INLINE void ARM_MPU_SetRegion(uint32_t rbar, uint32_t rasr) 00151 { 00152 MPU->RBAR = rbar; 00153 MPU->RASR = rasr; 00154 } 00155 00156 /** Configure the given MPU region. 00157 * \param rnr Region number to be configured. 00158 * \param rbar Value for RBAR register. 00159 * \param rsar Value for RSAR register. 00160 */ 00161 __STATIC_INLINE void ARM_MPU_SetRegionEx(uint32_t rnr, uint32_t rbar, uint32_t rasr) 00162 { 00163 MPU->RNR = rnr; 00164 MPU->RBAR = rbar; 00165 MPU->RASR = rasr; 00166 } 00167 00168 /** Memcopy with strictly ordered memory access, e.g. for register targets. 00169 * \param dst Destination data is copied to. 00170 * \param src Source data is copied from. 00171 * \param len Amount of data words to be copied. 00172 */ 00173 __STATIC_INLINE void orderedCpy(volatile uint32_t* dst, const uint32_t* __RESTRICT src, uint32_t len) 00174 { 00175 uint32_t i; 00176 for (i = 0U; i < len; ++i) 00177 { 00178 dst[i] = src[i]; 00179 } 00180 } 00181 00182 /** Load the given number of MPU regions from a table. 00183 * \param table Pointer to the MPU configuration table. 00184 * \param cnt Amount of regions to be configured. 00185 */ 00186 __STATIC_INLINE void ARM_MPU_Load(ARM_MPU_Region_t const* table, uint32_t cnt) 00187 { 00188 const uint32_t rowWordSize = sizeof(ARM_MPU_Region_t)/4U; 00189 while (cnt > MPU_TYPE_RALIASES) { 00190 orderedCpy(&(MPU->RBAR), &(table->RBAR), MPU_TYPE_RALIASES*rowWordSize); 00191 table += MPU_TYPE_RALIASES; 00192 cnt -= MPU_TYPE_RALIASES; 00193 } 00194 orderedCpy(&(MPU->RBAR), &(table->RBAR), cnt*rowWordSize); 00195 } 00196 00197 #endif 00198
Generated on Tue Jul 12 2022 16:47:29 by
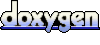