Aded CMSIS5 DSP and NN folder. Needs some work
Embed:
(wiki syntax)
Show/hide line numbers
core_cm0.h
Go to the documentation of this file.
00001 /**************************************************************************//** 00002 * @file core_cm0.h 00003 * @brief CMSIS Cortex-M0 Core Peripheral Access Layer Header File 00004 * @version V5.0.3 00005 * @date 10. January 2018 00006 ******************************************************************************/ 00007 /* 00008 * Copyright (c) 2009-2018 Arm Limited. All rights reserved. 00009 * 00010 * SPDX-License-Identifier: Apache-2.0 00011 * 00012 * Licensed under the Apache License, Version 2.0 (the License); you may 00013 * not use this file except in compliance with the License. 00014 * You may obtain a copy of the License at 00015 * 00016 * www.apache.org/licenses/LICENSE-2.0 00017 * 00018 * Unless required by applicable law or agreed to in writing, software 00019 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00020 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00021 * See the License for the specific language governing permissions and 00022 * limitations under the License. 00023 */ 00024 00025 #if defined ( __ICCARM__ ) 00026 #pragma system_include /* treat file as system include file for MISRA check */ 00027 #elif defined (__clang__) 00028 #pragma clang system_header /* treat file as system include file */ 00029 #endif 00030 00031 #ifndef __CORE_CM0_H_GENERIC 00032 #define __CORE_CM0_H_GENERIC 00033 00034 #include <stdint.h> 00035 00036 #ifdef __cplusplus 00037 extern "C" { 00038 #endif 00039 00040 /** 00041 \page CMSIS_MISRA_Exceptions MISRA-C:2004 Compliance Exceptions 00042 CMSIS violates the following MISRA-C:2004 rules: 00043 00044 \li Required Rule 8.5, object/function definition in header file.<br> 00045 Function definitions in header files are used to allow 'inlining'. 00046 00047 \li Required Rule 18.4, declaration of union type or object of union type: '{...}'.<br> 00048 Unions are used for effective representation of core registers. 00049 00050 \li Advisory Rule 19.7, Function-like macro defined.<br> 00051 Function-like macros are used to allow more efficient code. 00052 */ 00053 00054 00055 /******************************************************************************* 00056 * CMSIS definitions 00057 ******************************************************************************/ 00058 /** 00059 \ingroup Cortex_M0 00060 @{ 00061 */ 00062 00063 #include "cmsis_version.h" 00064 00065 /* CMSIS CM0 definitions */ 00066 #define __CM0_CMSIS_VERSION_MAIN (__CM_CMSIS_VERSION_MAIN) /*!< \deprecated [31:16] CMSIS HAL main version */ 00067 #define __CM0_CMSIS_VERSION_SUB (__CM_CMSIS_VERSION_SUB) /*!< \deprecated [15:0] CMSIS HAL sub version */ 00068 #define __CM0_CMSIS_VERSION ((__CM0_CMSIS_VERSION_MAIN << 16U) | \ 00069 __CM0_CMSIS_VERSION_SUB ) /*!< \deprecated CMSIS HAL version number */ 00070 00071 #define __CORTEX_M (0U) /*!< Cortex-M Core */ 00072 00073 /** __FPU_USED indicates whether an FPU is used or not. 00074 This core does not support an FPU at all 00075 */ 00076 #define __FPU_USED 0U 00077 00078 #if defined ( __CC_ARM ) 00079 #if defined __TARGET_FPU_VFP 00080 #error "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00081 #endif 00082 00083 #elif defined (__ARMCC_VERSION) && (__ARMCC_VERSION >= 6010050) 00084 #if defined __ARM_PCS_VFP 00085 #error "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00086 #endif 00087 00088 #elif defined ( __GNUC__ ) 00089 #if defined (__VFP_FP__) && !defined(__SOFTFP__) 00090 #error "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00091 #endif 00092 00093 #elif defined ( __ICCARM__ ) 00094 #if defined __ARMVFP__ 00095 #error "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00096 #endif 00097 00098 #elif defined ( __TI_ARM__ ) 00099 #if defined __TI_VFP_SUPPORT__ 00100 #error "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00101 #endif 00102 00103 #elif defined ( __TASKING__ ) 00104 #if defined __FPU_VFP__ 00105 #error "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00106 #endif 00107 00108 #elif defined ( __CSMC__ ) 00109 #if ( __CSMC__ & 0x400U) 00110 #error "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00111 #endif 00112 00113 #endif 00114 00115 #include "cmsis_compiler.h" /* CMSIS compiler specific defines */ 00116 00117 00118 #ifdef __cplusplus 00119 } 00120 #endif 00121 00122 #endif /* __CORE_CM0_H_GENERIC */ 00123 00124 #ifndef __CMSIS_GENERIC 00125 00126 #ifndef __CORE_CM0_H_DEPENDANT 00127 #define __CORE_CM0_H_DEPENDANT 00128 00129 #ifdef __cplusplus 00130 extern "C" { 00131 #endif 00132 00133 /* check device defines and use defaults */ 00134 #if defined __CHECK_DEVICE_DEFINES 00135 #ifndef __CM0_REV 00136 #define __CM0_REV 0x0000U 00137 #warning "__CM0_REV not defined in device header file; using default!" 00138 #endif 00139 00140 #ifndef __NVIC_PRIO_BITS 00141 #define __NVIC_PRIO_BITS 2U 00142 #warning "__NVIC_PRIO_BITS not defined in device header file; using default!" 00143 #endif 00144 00145 #ifndef __Vendor_SysTickConfig 00146 #define __Vendor_SysTickConfig 0U 00147 #warning "__Vendor_SysTickConfig not defined in device header file; using default!" 00148 #endif 00149 #endif 00150 00151 /* IO definitions (access restrictions to peripheral registers) */ 00152 /** 00153 \defgroup CMSIS_glob_defs CMSIS Global Defines 00154 00155 <strong>IO Type Qualifiers</strong> are used 00156 \li to specify the access to peripheral variables. 00157 \li for automatic generation of peripheral register debug information. 00158 */ 00159 #ifdef __cplusplus 00160 #define __I volatile /*!< Defines 'read only' permissions */ 00161 #else 00162 #define __I volatile const /*!< Defines 'read only' permissions */ 00163 #endif 00164 #define __O volatile /*!< Defines 'write only' permissions */ 00165 #define __IO volatile /*!< Defines 'read / write' permissions */ 00166 00167 /* following defines should be used for structure members */ 00168 #define __IM volatile const /*! Defines 'read only' structure member permissions */ 00169 #define __OM volatile /*! Defines 'write only' structure member permissions */ 00170 #define __IOM volatile /*! Defines 'read / write' structure member permissions */ 00171 00172 /*@} end of group Cortex_M0 */ 00173 00174 00175 00176 /******************************************************************************* 00177 * Register Abstraction 00178 Core Register contain: 00179 - Core Register 00180 - Core NVIC Register 00181 - Core SCB Register 00182 - Core SysTick Register 00183 ******************************************************************************/ 00184 /** 00185 \defgroup CMSIS_core_register Defines and Type Definitions 00186 \brief Type definitions and defines for Cortex-M processor based devices. 00187 */ 00188 00189 /** 00190 \ingroup CMSIS_core_register 00191 \defgroup CMSIS_CORE Status and Control Registers 00192 \brief Core Register type definitions. 00193 @{ 00194 */ 00195 00196 /** 00197 \brief Union type to access the Application Program Status Register (APSR). 00198 */ 00199 typedef union 00200 { 00201 struct 00202 { 00203 uint32_t _reserved0:28; /*!< bit: 0..27 Reserved */ 00204 uint32_t V:1; /*!< bit: 28 Overflow condition code flag */ 00205 uint32_t C:1; /*!< bit: 29 Carry condition code flag */ 00206 uint32_t Z:1; /*!< bit: 30 Zero condition code flag */ 00207 uint32_t N:1; /*!< bit: 31 Negative condition code flag */ 00208 } b; /*!< Structure used for bit access */ 00209 uint32_t w; /*!< Type used for word access */ 00210 } APSR_Type; 00211 00212 /* APSR Register Definitions */ 00213 #define APSR_N_Pos 31U /*!< APSR: N Position */ 00214 #define APSR_N_Msk (1UL << APSR_N_Pos) /*!< APSR: N Mask */ 00215 00216 #define APSR_Z_Pos 30U /*!< APSR: Z Position */ 00217 #define APSR_Z_Msk (1UL << APSR_Z_Pos) /*!< APSR: Z Mask */ 00218 00219 #define APSR_C_Pos 29U /*!< APSR: C Position */ 00220 #define APSR_C_Msk (1UL << APSR_C_Pos) /*!< APSR: C Mask */ 00221 00222 #define APSR_V_Pos 28U /*!< APSR: V Position */ 00223 #define APSR_V_Msk (1UL << APSR_V_Pos) /*!< APSR: V Mask */ 00224 00225 00226 /** 00227 \brief Union type to access the Interrupt Program Status Register (IPSR). 00228 */ 00229 typedef union 00230 { 00231 struct 00232 { 00233 uint32_t ISR:9; /*!< bit: 0.. 8 Exception number */ 00234 uint32_t _reserved0:23; /*!< bit: 9..31 Reserved */ 00235 } b; /*!< Structure used for bit access */ 00236 uint32_t w; /*!< Type used for word access */ 00237 } IPSR_Type; 00238 00239 /* IPSR Register Definitions */ 00240 #define IPSR_ISR_Pos 0U /*!< IPSR: ISR Position */ 00241 #define IPSR_ISR_Msk (0x1FFUL /*<< IPSR_ISR_Pos*/) /*!< IPSR: ISR Mask */ 00242 00243 00244 /** 00245 \brief Union type to access the Special-Purpose Program Status Registers (xPSR). 00246 */ 00247 typedef union 00248 { 00249 struct 00250 { 00251 uint32_t ISR:9; /*!< bit: 0.. 8 Exception number */ 00252 uint32_t _reserved0:15; /*!< bit: 9..23 Reserved */ 00253 uint32_t T:1; /*!< bit: 24 Thumb bit (read 0) */ 00254 uint32_t _reserved1:3; /*!< bit: 25..27 Reserved */ 00255 uint32_t V:1; /*!< bit: 28 Overflow condition code flag */ 00256 uint32_t C:1; /*!< bit: 29 Carry condition code flag */ 00257 uint32_t Z:1; /*!< bit: 30 Zero condition code flag */ 00258 uint32_t N:1; /*!< bit: 31 Negative condition code flag */ 00259 } b; /*!< Structure used for bit access */ 00260 uint32_t w; /*!< Type used for word access */ 00261 } xPSR_Type; 00262 00263 /* xPSR Register Definitions */ 00264 #define xPSR_N_Pos 31U /*!< xPSR: N Position */ 00265 #define xPSR_N_Msk (1UL << xPSR_N_Pos) /*!< xPSR: N Mask */ 00266 00267 #define xPSR_Z_Pos 30U /*!< xPSR: Z Position */ 00268 #define xPSR_Z_Msk (1UL << xPSR_Z_Pos) /*!< xPSR: Z Mask */ 00269 00270 #define xPSR_C_Pos 29U /*!< xPSR: C Position */ 00271 #define xPSR_C_Msk (1UL << xPSR_C_Pos) /*!< xPSR: C Mask */ 00272 00273 #define xPSR_V_Pos 28U /*!< xPSR: V Position */ 00274 #define xPSR_V_Msk (1UL << xPSR_V_Pos) /*!< xPSR: V Mask */ 00275 00276 #define xPSR_T_Pos 24U /*!< xPSR: T Position */ 00277 #define xPSR_T_Msk (1UL << xPSR_T_Pos) /*!< xPSR: T Mask */ 00278 00279 #define xPSR_ISR_Pos 0U /*!< xPSR: ISR Position */ 00280 #define xPSR_ISR_Msk (0x1FFUL /*<< xPSR_ISR_Pos*/) /*!< xPSR: ISR Mask */ 00281 00282 00283 /** 00284 \brief Union type to access the Control Registers (CONTROL). 00285 */ 00286 typedef union 00287 { 00288 struct 00289 { 00290 uint32_t _reserved0:1; /*!< bit: 0 Reserved */ 00291 uint32_t SPSEL:1; /*!< bit: 1 Stack to be used */ 00292 uint32_t _reserved1:30; /*!< bit: 2..31 Reserved */ 00293 } b; /*!< Structure used for bit access */ 00294 uint32_t w; /*!< Type used for word access */ 00295 } CONTROL_Type; 00296 00297 /* CONTROL Register Definitions */ 00298 #define CONTROL_SPSEL_Pos 1U /*!< CONTROL: SPSEL Position */ 00299 #define CONTROL_SPSEL_Msk (1UL << CONTROL_SPSEL_Pos) /*!< CONTROL: SPSEL Mask */ 00300 00301 /*@} end of group CMSIS_CORE */ 00302 00303 00304 /** 00305 \ingroup CMSIS_core_register 00306 \defgroup CMSIS_NVIC Nested Vectored Interrupt Controller (NVIC) 00307 \brief Type definitions for the NVIC Registers 00308 @{ 00309 */ 00310 00311 /** 00312 \brief Structure type to access the Nested Vectored Interrupt Controller (NVIC). 00313 */ 00314 typedef struct 00315 { 00316 __IOM uint32_t ISER[1U]; /*!< Offset: 0x000 (R/W) Interrupt Set Enable Register */ 00317 uint32_t RESERVED0[31U]; 00318 __IOM uint32_t ICER[1U]; /*!< Offset: 0x080 (R/W) Interrupt Clear Enable Register */ 00319 uint32_t RSERVED1[31U]; 00320 __IOM uint32_t ISPR[1U]; /*!< Offset: 0x100 (R/W) Interrupt Set Pending Register */ 00321 uint32_t RESERVED2[31U]; 00322 __IOM uint32_t ICPR[1U]; /*!< Offset: 0x180 (R/W) Interrupt Clear Pending Register */ 00323 uint32_t RESERVED3[31U]; 00324 uint32_t RESERVED4[64U]; 00325 __IOM uint32_t IP[8U]; /*!< Offset: 0x300 (R/W) Interrupt Priority Register */ 00326 } NVIC_Type; 00327 00328 /*@} end of group CMSIS_NVIC */ 00329 00330 00331 /** 00332 \ingroup CMSIS_core_register 00333 \defgroup CMSIS_SCB System Control Block (SCB) 00334 \brief Type definitions for the System Control Block Registers 00335 @{ 00336 */ 00337 00338 /** 00339 \brief Structure type to access the System Control Block (SCB). 00340 */ 00341 typedef struct 00342 { 00343 __IM uint32_t CPUID; /*!< Offset: 0x000 (R/ ) CPUID Base Register */ 00344 __IOM uint32_t ICSR; /*!< Offset: 0x004 (R/W) Interrupt Control and State Register */ 00345 uint32_t RESERVED0; 00346 __IOM uint32_t AIRCR; /*!< Offset: 0x00C (R/W) Application Interrupt and Reset Control Register */ 00347 __IOM uint32_t SCR; /*!< Offset: 0x010 (R/W) System Control Register */ 00348 __IOM uint32_t CCR; /*!< Offset: 0x014 (R/W) Configuration Control Register */ 00349 uint32_t RESERVED1; 00350 __IOM uint32_t SHP[2U]; /*!< Offset: 0x01C (R/W) System Handlers Priority Registers. [0] is RESERVED */ 00351 __IOM uint32_t SHCSR; /*!< Offset: 0x024 (R/W) System Handler Control and State Register */ 00352 } SCB_Type; 00353 00354 /* SCB CPUID Register Definitions */ 00355 #define SCB_CPUID_IMPLEMENTER_Pos 24U /*!< SCB CPUID: IMPLEMENTER Position */ 00356 #define SCB_CPUID_IMPLEMENTER_Msk (0xFFUL << SCB_CPUID_IMPLEMENTER_Pos) /*!< SCB CPUID: IMPLEMENTER Mask */ 00357 00358 #define SCB_CPUID_VARIANT_Pos 20U /*!< SCB CPUID: VARIANT Position */ 00359 #define SCB_CPUID_VARIANT_Msk (0xFUL << SCB_CPUID_VARIANT_Pos) /*!< SCB CPUID: VARIANT Mask */ 00360 00361 #define SCB_CPUID_ARCHITECTURE_Pos 16U /*!< SCB CPUID: ARCHITECTURE Position */ 00362 #define SCB_CPUID_ARCHITECTURE_Msk (0xFUL << SCB_CPUID_ARCHITECTURE_Pos) /*!< SCB CPUID: ARCHITECTURE Mask */ 00363 00364 #define SCB_CPUID_PARTNO_Pos 4U /*!< SCB CPUID: PARTNO Position */ 00365 #define SCB_CPUID_PARTNO_Msk (0xFFFUL << SCB_CPUID_PARTNO_Pos) /*!< SCB CPUID: PARTNO Mask */ 00366 00367 #define SCB_CPUID_REVISION_Pos 0U /*!< SCB CPUID: REVISION Position */ 00368 #define SCB_CPUID_REVISION_Msk (0xFUL /*<< SCB_CPUID_REVISION_Pos*/) /*!< SCB CPUID: REVISION Mask */ 00369 00370 /* SCB Interrupt Control State Register Definitions */ 00371 #define SCB_ICSR_NMIPENDSET_Pos 31U /*!< SCB ICSR: NMIPENDSET Position */ 00372 #define SCB_ICSR_NMIPENDSET_Msk (1UL << SCB_ICSR_NMIPENDSET_Pos) /*!< SCB ICSR: NMIPENDSET Mask */ 00373 00374 #define SCB_ICSR_PENDSVSET_Pos 28U /*!< SCB ICSR: PENDSVSET Position */ 00375 #define SCB_ICSR_PENDSVSET_Msk (1UL << SCB_ICSR_PENDSVSET_Pos) /*!< SCB ICSR: PENDSVSET Mask */ 00376 00377 #define SCB_ICSR_PENDSVCLR_Pos 27U /*!< SCB ICSR: PENDSVCLR Position */ 00378 #define SCB_ICSR_PENDSVCLR_Msk (1UL << SCB_ICSR_PENDSVCLR_Pos) /*!< SCB ICSR: PENDSVCLR Mask */ 00379 00380 #define SCB_ICSR_PENDSTSET_Pos 26U /*!< SCB ICSR: PENDSTSET Position */ 00381 #define SCB_ICSR_PENDSTSET_Msk (1UL << SCB_ICSR_PENDSTSET_Pos) /*!< SCB ICSR: PENDSTSET Mask */ 00382 00383 #define SCB_ICSR_PENDSTCLR_Pos 25U /*!< SCB ICSR: PENDSTCLR Position */ 00384 #define SCB_ICSR_PENDSTCLR_Msk (1UL << SCB_ICSR_PENDSTCLR_Pos) /*!< SCB ICSR: PENDSTCLR Mask */ 00385 00386 #define SCB_ICSR_ISRPREEMPT_Pos 23U /*!< SCB ICSR: ISRPREEMPT Position */ 00387 #define SCB_ICSR_ISRPREEMPT_Msk (1UL << SCB_ICSR_ISRPREEMPT_Pos) /*!< SCB ICSR: ISRPREEMPT Mask */ 00388 00389 #define SCB_ICSR_ISRPENDING_Pos 22U /*!< SCB ICSR: ISRPENDING Position */ 00390 #define SCB_ICSR_ISRPENDING_Msk (1UL << SCB_ICSR_ISRPENDING_Pos) /*!< SCB ICSR: ISRPENDING Mask */ 00391 00392 #define SCB_ICSR_VECTPENDING_Pos 12U /*!< SCB ICSR: VECTPENDING Position */ 00393 #define SCB_ICSR_VECTPENDING_Msk (0x1FFUL << SCB_ICSR_VECTPENDING_Pos) /*!< SCB ICSR: VECTPENDING Mask */ 00394 00395 #define SCB_ICSR_VECTACTIVE_Pos 0U /*!< SCB ICSR: VECTACTIVE Position */ 00396 #define SCB_ICSR_VECTACTIVE_Msk (0x1FFUL /*<< SCB_ICSR_VECTACTIVE_Pos*/) /*!< SCB ICSR: VECTACTIVE Mask */ 00397 00398 /* SCB Application Interrupt and Reset Control Register Definitions */ 00399 #define SCB_AIRCR_VECTKEY_Pos 16U /*!< SCB AIRCR: VECTKEY Position */ 00400 #define SCB_AIRCR_VECTKEY_Msk (0xFFFFUL << SCB_AIRCR_VECTKEY_Pos) /*!< SCB AIRCR: VECTKEY Mask */ 00401 00402 #define SCB_AIRCR_VECTKEYSTAT_Pos 16U /*!< SCB AIRCR: VECTKEYSTAT Position */ 00403 #define SCB_AIRCR_VECTKEYSTAT_Msk (0xFFFFUL << SCB_AIRCR_VECTKEYSTAT_Pos) /*!< SCB AIRCR: VECTKEYSTAT Mask */ 00404 00405 #define SCB_AIRCR_ENDIANESS_Pos 15U /*!< SCB AIRCR: ENDIANESS Position */ 00406 #define SCB_AIRCR_ENDIANESS_Msk (1UL << SCB_AIRCR_ENDIANESS_Pos) /*!< SCB AIRCR: ENDIANESS Mask */ 00407 00408 #define SCB_AIRCR_SYSRESETREQ_Pos 2U /*!< SCB AIRCR: SYSRESETREQ Position */ 00409 #define SCB_AIRCR_SYSRESETREQ_Msk (1UL << SCB_AIRCR_SYSRESETREQ_Pos) /*!< SCB AIRCR: SYSRESETREQ Mask */ 00410 00411 #define SCB_AIRCR_VECTCLRACTIVE_Pos 1U /*!< SCB AIRCR: VECTCLRACTIVE Position */ 00412 #define SCB_AIRCR_VECTCLRACTIVE_Msk (1UL << SCB_AIRCR_VECTCLRACTIVE_Pos) /*!< SCB AIRCR: VECTCLRACTIVE Mask */ 00413 00414 /* SCB System Control Register Definitions */ 00415 #define SCB_SCR_SEVONPEND_Pos 4U /*!< SCB SCR: SEVONPEND Position */ 00416 #define SCB_SCR_SEVONPEND_Msk (1UL << SCB_SCR_SEVONPEND_Pos) /*!< SCB SCR: SEVONPEND Mask */ 00417 00418 #define SCB_SCR_SLEEPDEEP_Pos 2U /*!< SCB SCR: SLEEPDEEP Position */ 00419 #define SCB_SCR_SLEEPDEEP_Msk (1UL << SCB_SCR_SLEEPDEEP_Pos) /*!< SCB SCR: SLEEPDEEP Mask */ 00420 00421 #define SCB_SCR_SLEEPONEXIT_Pos 1U /*!< SCB SCR: SLEEPONEXIT Position */ 00422 #define SCB_SCR_SLEEPONEXIT_Msk (1UL << SCB_SCR_SLEEPONEXIT_Pos) /*!< SCB SCR: SLEEPONEXIT Mask */ 00423 00424 /* SCB Configuration Control Register Definitions */ 00425 #define SCB_CCR_STKALIGN_Pos 9U /*!< SCB CCR: STKALIGN Position */ 00426 #define SCB_CCR_STKALIGN_Msk (1UL << SCB_CCR_STKALIGN_Pos) /*!< SCB CCR: STKALIGN Mask */ 00427 00428 #define SCB_CCR_UNALIGN_TRP_Pos 3U /*!< SCB CCR: UNALIGN_TRP Position */ 00429 #define SCB_CCR_UNALIGN_TRP_Msk (1UL << SCB_CCR_UNALIGN_TRP_Pos) /*!< SCB CCR: UNALIGN_TRP Mask */ 00430 00431 /* SCB System Handler Control and State Register Definitions */ 00432 #define SCB_SHCSR_SVCALLPENDED_Pos 15U /*!< SCB SHCSR: SVCALLPENDED Position */ 00433 #define SCB_SHCSR_SVCALLPENDED_Msk (1UL << SCB_SHCSR_SVCALLPENDED_Pos) /*!< SCB SHCSR: SVCALLPENDED Mask */ 00434 00435 /*@} end of group CMSIS_SCB */ 00436 00437 00438 /** 00439 \ingroup CMSIS_core_register 00440 \defgroup CMSIS_SysTick System Tick Timer (SysTick) 00441 \brief Type definitions for the System Timer Registers. 00442 @{ 00443 */ 00444 00445 /** 00446 \brief Structure type to access the System Timer (SysTick). 00447 */ 00448 typedef struct 00449 { 00450 __IOM uint32_t CTRL; /*!< Offset: 0x000 (R/W) SysTick Control and Status Register */ 00451 __IOM uint32_t LOAD; /*!< Offset: 0x004 (R/W) SysTick Reload Value Register */ 00452 __IOM uint32_t VAL; /*!< Offset: 0x008 (R/W) SysTick Current Value Register */ 00453 __IM uint32_t CALIB; /*!< Offset: 0x00C (R/ ) SysTick Calibration Register */ 00454 } SysTick_Type; 00455 00456 /* SysTick Control / Status Register Definitions */ 00457 #define SysTick_CTRL_COUNTFLAG_Pos 16U /*!< SysTick CTRL: COUNTFLAG Position */ 00458 #define SysTick_CTRL_COUNTFLAG_Msk (1UL << SysTick_CTRL_COUNTFLAG_Pos) /*!< SysTick CTRL: COUNTFLAG Mask */ 00459 00460 #define SysTick_CTRL_CLKSOURCE_Pos 2U /*!< SysTick CTRL: CLKSOURCE Position */ 00461 #define SysTick_CTRL_CLKSOURCE_Msk (1UL << SysTick_CTRL_CLKSOURCE_Pos) /*!< SysTick CTRL: CLKSOURCE Mask */ 00462 00463 #define SysTick_CTRL_TICKINT_Pos 1U /*!< SysTick CTRL: TICKINT Position */ 00464 #define SysTick_CTRL_TICKINT_Msk (1UL << SysTick_CTRL_TICKINT_Pos) /*!< SysTick CTRL: TICKINT Mask */ 00465 00466 #define SysTick_CTRL_ENABLE_Pos 0U /*!< SysTick CTRL: ENABLE Position */ 00467 #define SysTick_CTRL_ENABLE_Msk (1UL /*<< SysTick_CTRL_ENABLE_Pos*/) /*!< SysTick CTRL: ENABLE Mask */ 00468 00469 /* SysTick Reload Register Definitions */ 00470 #define SysTick_LOAD_RELOAD_Pos 0U /*!< SysTick LOAD: RELOAD Position */ 00471 #define SysTick_LOAD_RELOAD_Msk (0xFFFFFFUL /*<< SysTick_LOAD_RELOAD_Pos*/) /*!< SysTick LOAD: RELOAD Mask */ 00472 00473 /* SysTick Current Register Definitions */ 00474 #define SysTick_VAL_CURRENT_Pos 0U /*!< SysTick VAL: CURRENT Position */ 00475 #define SysTick_VAL_CURRENT_Msk (0xFFFFFFUL /*<< SysTick_VAL_CURRENT_Pos*/) /*!< SysTick VAL: CURRENT Mask */ 00476 00477 /* SysTick Calibration Register Definitions */ 00478 #define SysTick_CALIB_NOREF_Pos 31U /*!< SysTick CALIB: NOREF Position */ 00479 #define SysTick_CALIB_NOREF_Msk (1UL << SysTick_CALIB_NOREF_Pos) /*!< SysTick CALIB: NOREF Mask */ 00480 00481 #define SysTick_CALIB_SKEW_Pos 30U /*!< SysTick CALIB: SKEW Position */ 00482 #define SysTick_CALIB_SKEW_Msk (1UL << SysTick_CALIB_SKEW_Pos) /*!< SysTick CALIB: SKEW Mask */ 00483 00484 #define SysTick_CALIB_TENMS_Pos 0U /*!< SysTick CALIB: TENMS Position */ 00485 #define SysTick_CALIB_TENMS_Msk (0xFFFFFFUL /*<< SysTick_CALIB_TENMS_Pos*/) /*!< SysTick CALIB: TENMS Mask */ 00486 00487 /*@} end of group CMSIS_SysTick */ 00488 00489 00490 /** 00491 \ingroup CMSIS_core_register 00492 \defgroup CMSIS_CoreDebug Core Debug Registers (CoreDebug) 00493 \brief Cortex-M0 Core Debug Registers (DCB registers, SHCSR, and DFSR) are only accessible over DAP and not via processor. 00494 Therefore they are not covered by the Cortex-M0 header file. 00495 @{ 00496 */ 00497 /*@} end of group CMSIS_CoreDebug */ 00498 00499 00500 /** 00501 \ingroup CMSIS_core_register 00502 \defgroup CMSIS_core_bitfield Core register bit field macros 00503 \brief Macros for use with bit field definitions (xxx_Pos, xxx_Msk). 00504 @{ 00505 */ 00506 00507 /** 00508 \brief Mask and shift a bit field value for use in a register bit range. 00509 \param[in] field Name of the register bit field. 00510 \param[in] value Value of the bit field. This parameter is interpreted as an uint32_t type. 00511 \return Masked and shifted value. 00512 */ 00513 #define _VAL2FLD(field, value) (((uint32_t)(value) << field ## _Pos) & field ## _Msk) 00514 00515 /** 00516 \brief Mask and shift a register value to extract a bit filed value. 00517 \param[in] field Name of the register bit field. 00518 \param[in] value Value of register. This parameter is interpreted as an uint32_t type. 00519 \return Masked and shifted bit field value. 00520 */ 00521 #define _FLD2VAL(field, value) (((uint32_t)(value) & field ## _Msk) >> field ## _Pos) 00522 00523 /*@} end of group CMSIS_core_bitfield */ 00524 00525 00526 /** 00527 \ingroup CMSIS_core_register 00528 \defgroup CMSIS_core_base Core Definitions 00529 \brief Definitions for base addresses, unions, and structures. 00530 @{ 00531 */ 00532 00533 /* Memory mapping of Core Hardware */ 00534 #define SCS_BASE (0xE000E000UL) /*!< System Control Space Base Address */ 00535 #define SysTick_BASE (SCS_BASE + 0x0010UL) /*!< SysTick Base Address */ 00536 #define NVIC_BASE (SCS_BASE + 0x0100UL) /*!< NVIC Base Address */ 00537 #define SCB_BASE (SCS_BASE + 0x0D00UL) /*!< System Control Block Base Address */ 00538 00539 #define SCB ((SCB_Type *) SCB_BASE ) /*!< SCB configuration struct */ 00540 #define SysTick ((SysTick_Type *) SysTick_BASE ) /*!< SysTick configuration struct */ 00541 #define NVIC ((NVIC_Type *) NVIC_BASE ) /*!< NVIC configuration struct */ 00542 00543 00544 /*@} */ 00545 00546 00547 00548 /******************************************************************************* 00549 * Hardware Abstraction Layer 00550 Core Function Interface contains: 00551 - Core NVIC Functions 00552 - Core SysTick Functions 00553 - Core Register Access Functions 00554 ******************************************************************************/ 00555 /** 00556 \defgroup CMSIS_Core_FunctionInterface Functions and Instructions Reference 00557 */ 00558 00559 00560 00561 /* ########################## NVIC functions #################################### */ 00562 /** 00563 \ingroup CMSIS_Core_FunctionInterface 00564 \defgroup CMSIS_Core_NVICFunctions NVIC Functions 00565 \brief Functions that manage interrupts and exceptions via the NVIC. 00566 @{ 00567 */ 00568 00569 #ifdef CMSIS_NVIC_VIRTUAL 00570 #ifndef CMSIS_NVIC_VIRTUAL_HEADER_FILE 00571 #define CMSIS_NVIC_VIRTUAL_HEADER_FILE "cmsis_nvic_virtual.h" 00572 #endif 00573 #include CMSIS_NVIC_VIRTUAL_HEADER_FILE 00574 #else 00575 /*#define NVIC_SetPriorityGrouping __NVIC_SetPriorityGrouping not available for Cortex-M0 */ 00576 /*#define NVIC_GetPriorityGrouping __NVIC_GetPriorityGrouping not available for Cortex-M0 */ 00577 #define NVIC_EnableIRQ __NVIC_EnableIRQ 00578 #define NVIC_GetEnableIRQ __NVIC_GetEnableIRQ 00579 #define NVIC_DisableIRQ __NVIC_DisableIRQ 00580 #define NVIC_GetPendingIRQ __NVIC_GetPendingIRQ 00581 #define NVIC_SetPendingIRQ __NVIC_SetPendingIRQ 00582 #define NVIC_ClearPendingIRQ __NVIC_ClearPendingIRQ 00583 /*#define NVIC_GetActive __NVIC_GetActive not available for Cortex-M0 */ 00584 #define NVIC_SetPriority __NVIC_SetPriority 00585 #define NVIC_GetPriority __NVIC_GetPriority 00586 #define NVIC_SystemReset __NVIC_SystemReset 00587 #endif /* CMSIS_NVIC_VIRTUAL */ 00588 00589 #ifdef CMSIS_VECTAB_VIRTUAL 00590 #ifndef CMSIS_VECTAB_VIRTUAL_HEADER_FILE 00591 #define CMSIS_VECTAB_VIRTUAL_HEADER_FILE "cmsis_vectab_virtual.h" 00592 #endif 00593 #include CMSIS_VECTAB_VIRTUAL_HEADER_FILE 00594 #else 00595 #define NVIC_SetVector __NVIC_SetVector 00596 #define NVIC_GetVector __NVIC_GetVector 00597 #endif /* (CMSIS_VECTAB_VIRTUAL) */ 00598 00599 #define NVIC_USER_IRQ_OFFSET 16 00600 00601 00602 /* Interrupt Priorities are WORD accessible only under Armv6-M */ 00603 /* The following MACROS handle generation of the register offset and byte masks */ 00604 #define _BIT_SHIFT(IRQn) ( ((((uint32_t)(int32_t)(IRQn)) ) & 0x03UL) * 8UL) 00605 #define _SHP_IDX(IRQn) ( (((((uint32_t)(int32_t)(IRQn)) & 0x0FUL)-8UL) >> 2UL) ) 00606 #define _IP_IDX(IRQn) ( (((uint32_t)(int32_t)(IRQn)) >> 2UL) ) 00607 00608 00609 /** 00610 \brief Enable Interrupt 00611 \details Enables a device specific interrupt in the NVIC interrupt controller. 00612 \param [in] IRQn Device specific interrupt number. 00613 \note IRQn must not be negative. 00614 */ 00615 __STATIC_INLINE void __NVIC_EnableIRQ(IRQn_Type IRQn) 00616 { 00617 if ((int32_t)(IRQn) >= 0) 00618 { 00619 NVIC->ISER[0U] = (uint32_t)(1UL << (((uint32_t)IRQn) & 0x1FUL)); 00620 } 00621 } 00622 00623 00624 /** 00625 \brief Get Interrupt Enable status 00626 \details Returns a device specific interrupt enable status from the NVIC interrupt controller. 00627 \param [in] IRQn Device specific interrupt number. 00628 \return 0 Interrupt is not enabled. 00629 \return 1 Interrupt is enabled. 00630 \note IRQn must not be negative. 00631 */ 00632 __STATIC_INLINE uint32_t __NVIC_GetEnableIRQ(IRQn_Type IRQn) 00633 { 00634 if ((int32_t)(IRQn) >= 0) 00635 { 00636 return((uint32_t)(((NVIC->ISER[0U] & (1UL << (((uint32_t)IRQn) & 0x1FUL))) != 0UL) ? 1UL : 0UL)); 00637 } 00638 else 00639 { 00640 return(0U); 00641 } 00642 } 00643 00644 00645 /** 00646 \brief Disable Interrupt 00647 \details Disables a device specific interrupt in the NVIC interrupt controller. 00648 \param [in] IRQn Device specific interrupt number. 00649 \note IRQn must not be negative. 00650 */ 00651 __STATIC_INLINE void __NVIC_DisableIRQ(IRQn_Type IRQn) 00652 { 00653 if ((int32_t)(IRQn) >= 0) 00654 { 00655 NVIC->ICER[0U] = (uint32_t)(1UL << (((uint32_t)IRQn) & 0x1FUL)); 00656 __DSB(); 00657 __ISB(); 00658 } 00659 } 00660 00661 00662 /** 00663 \brief Get Pending Interrupt 00664 \details Reads the NVIC pending register and returns the pending bit for the specified device specific interrupt. 00665 \param [in] IRQn Device specific interrupt number. 00666 \return 0 Interrupt status is not pending. 00667 \return 1 Interrupt status is pending. 00668 \note IRQn must not be negative. 00669 */ 00670 __STATIC_INLINE uint32_t __NVIC_GetPendingIRQ(IRQn_Type IRQn) 00671 { 00672 if ((int32_t)(IRQn) >= 0) 00673 { 00674 return((uint32_t)(((NVIC->ISPR[0U] & (1UL << (((uint32_t)IRQn) & 0x1FUL))) != 0UL) ? 1UL : 0UL)); 00675 } 00676 else 00677 { 00678 return(0U); 00679 } 00680 } 00681 00682 00683 /** 00684 \brief Set Pending Interrupt 00685 \details Sets the pending bit of a device specific interrupt in the NVIC pending register. 00686 \param [in] IRQn Device specific interrupt number. 00687 \note IRQn must not be negative. 00688 */ 00689 __STATIC_INLINE void __NVIC_SetPendingIRQ(IRQn_Type IRQn) 00690 { 00691 if ((int32_t)(IRQn) >= 0) 00692 { 00693 NVIC->ISPR[0U] = (uint32_t)(1UL << (((uint32_t)IRQn) & 0x1FUL)); 00694 } 00695 } 00696 00697 00698 /** 00699 \brief Clear Pending Interrupt 00700 \details Clears the pending bit of a device specific interrupt in the NVIC pending register. 00701 \param [in] IRQn Device specific interrupt number. 00702 \note IRQn must not be negative. 00703 */ 00704 __STATIC_INLINE void __NVIC_ClearPendingIRQ(IRQn_Type IRQn) 00705 { 00706 if ((int32_t)(IRQn) >= 0) 00707 { 00708 NVIC->ICPR[0U] = (uint32_t)(1UL << (((uint32_t)IRQn) & 0x1FUL)); 00709 } 00710 } 00711 00712 00713 /** 00714 \brief Set Interrupt Priority 00715 \details Sets the priority of a device specific interrupt or a processor exception. 00716 The interrupt number can be positive to specify a device specific interrupt, 00717 or negative to specify a processor exception. 00718 \param [in] IRQn Interrupt number. 00719 \param [in] priority Priority to set. 00720 \note The priority cannot be set for every processor exception. 00721 */ 00722 __STATIC_INLINE void __NVIC_SetPriority(IRQn_Type IRQn, uint32_t priority) 00723 { 00724 if ((int32_t)(IRQn) >= 0) 00725 { 00726 NVIC->IP[_IP_IDX(IRQn)] = ((uint32_t)(NVIC->IP[_IP_IDX(IRQn)] & ~(0xFFUL << _BIT_SHIFT(IRQn))) | 00727 (((priority << (8U - __NVIC_PRIO_BITS)) & (uint32_t)0xFFUL) << _BIT_SHIFT(IRQn))); 00728 } 00729 else 00730 { 00731 SCB->SHP[_SHP_IDX(IRQn)] = ((uint32_t)(SCB->SHP[_SHP_IDX(IRQn)] & ~(0xFFUL << _BIT_SHIFT(IRQn))) | 00732 (((priority << (8U - __NVIC_PRIO_BITS)) & (uint32_t)0xFFUL) << _BIT_SHIFT(IRQn))); 00733 } 00734 } 00735 00736 00737 /** 00738 \brief Get Interrupt Priority 00739 \details Reads the priority of a device specific interrupt or a processor exception. 00740 The interrupt number can be positive to specify a device specific interrupt, 00741 or negative to specify a processor exception. 00742 \param [in] IRQn Interrupt number. 00743 \return Interrupt Priority. 00744 Value is aligned automatically to the implemented priority bits of the microcontroller. 00745 */ 00746 __STATIC_INLINE uint32_t __NVIC_GetPriority(IRQn_Type IRQn) 00747 { 00748 00749 if ((int32_t)(IRQn) >= 0) 00750 { 00751 return((uint32_t)(((NVIC->IP[ _IP_IDX(IRQn)] >> _BIT_SHIFT(IRQn) ) & (uint32_t)0xFFUL) >> (8U - __NVIC_PRIO_BITS))); 00752 } 00753 else 00754 { 00755 return((uint32_t)(((SCB->SHP[_SHP_IDX(IRQn)] >> _BIT_SHIFT(IRQn) ) & (uint32_t)0xFFUL) >> (8U - __NVIC_PRIO_BITS))); 00756 } 00757 } 00758 00759 00760 /** 00761 \brief Set Interrupt Vector 00762 \details Sets an interrupt vector in SRAM based interrupt vector table. 00763 The interrupt number can be positive to specify a device specific interrupt, 00764 or negative to specify a processor exception. 00765 Address 0 must be mapped to SRAM. 00766 \param [in] IRQn Interrupt number 00767 \param [in] vector Address of interrupt handler function 00768 */ 00769 __STATIC_INLINE void __NVIC_SetVector(IRQn_Type IRQn, uint32_t vector) 00770 { 00771 uint32_t *vectors = (uint32_t *)0x0U; 00772 vectors[(int32_t)IRQn + NVIC_USER_IRQ_OFFSET] = vector; 00773 } 00774 00775 00776 /** 00777 \brief Get Interrupt Vector 00778 \details Reads an interrupt vector from interrupt vector table. 00779 The interrupt number can be positive to specify a device specific interrupt, 00780 or negative to specify a processor exception. 00781 \param [in] IRQn Interrupt number. 00782 \return Address of interrupt handler function 00783 */ 00784 __STATIC_INLINE uint32_t __NVIC_GetVector(IRQn_Type IRQn) 00785 { 00786 uint32_t *vectors = (uint32_t *)0x0U; 00787 return vectors[(int32_t)IRQn + NVIC_USER_IRQ_OFFSET]; 00788 } 00789 00790 00791 /** 00792 \brief System Reset 00793 \details Initiates a system reset request to reset the MCU. 00794 */ 00795 __STATIC_INLINE void __NVIC_SystemReset(void) 00796 { 00797 __DSB(); /* Ensure all outstanding memory accesses included 00798 buffered write are completed before reset */ 00799 SCB->AIRCR = ((0x5FAUL << SCB_AIRCR_VECTKEY_Pos) | 00800 SCB_AIRCR_SYSRESETREQ_Msk); 00801 __DSB(); /* Ensure completion of memory access */ 00802 00803 for(;;) /* wait until reset */ 00804 { 00805 __NOP(); 00806 } 00807 } 00808 00809 /*@} end of CMSIS_Core_NVICFunctions */ 00810 00811 00812 /* ########################## FPU functions #################################### */ 00813 /** 00814 \ingroup CMSIS_Core_FunctionInterface 00815 \defgroup CMSIS_Core_FpuFunctions FPU Functions 00816 \brief Function that provides FPU type. 00817 @{ 00818 */ 00819 00820 /** 00821 \brief get FPU type 00822 \details returns the FPU type 00823 \returns 00824 - \b 0: No FPU 00825 - \b 1: Single precision FPU 00826 - \b 2: Double + Single precision FPU 00827 */ 00828 __STATIC_INLINE uint32_t SCB_GetFPUType(void) 00829 { 00830 return 0U; /* No FPU */ 00831 } 00832 00833 00834 /*@} end of CMSIS_Core_FpuFunctions */ 00835 00836 00837 00838 /* ################################## SysTick function ############################################ */ 00839 /** 00840 \ingroup CMSIS_Core_FunctionInterface 00841 \defgroup CMSIS_Core_SysTickFunctions SysTick Functions 00842 \brief Functions that configure the System. 00843 @{ 00844 */ 00845 00846 #if defined (__Vendor_SysTickConfig) && (__Vendor_SysTickConfig == 0U) 00847 00848 /** 00849 \brief System Tick Configuration 00850 \details Initializes the System Timer and its interrupt, and starts the System Tick Timer. 00851 Counter is in free running mode to generate periodic interrupts. 00852 \param [in] ticks Number of ticks between two interrupts. 00853 \return 0 Function succeeded. 00854 \return 1 Function failed. 00855 \note When the variable <b>__Vendor_SysTickConfig</b> is set to 1, then the 00856 function <b>SysTick_Config</b> is not included. In this case, the file <b><i>device</i>.h</b> 00857 must contain a vendor-specific implementation of this function. 00858 */ 00859 __STATIC_INLINE uint32_t SysTick_Config(uint32_t ticks) 00860 { 00861 if ((ticks - 1UL) > SysTick_LOAD_RELOAD_Msk) 00862 { 00863 return (1UL); /* Reload value impossible */ 00864 } 00865 00866 SysTick->LOAD = (uint32_t)(ticks - 1UL); /* set reload register */ 00867 NVIC_SetPriority (SysTick_IRQn, (1UL << __NVIC_PRIO_BITS) - 1UL); /* set Priority for Systick Interrupt */ 00868 SysTick->VAL = 0UL; /* Load the SysTick Counter Value */ 00869 SysTick->CTRL = SysTick_CTRL_CLKSOURCE_Msk | 00870 SysTick_CTRL_TICKINT_Msk | 00871 SysTick_CTRL_ENABLE_Msk; /* Enable SysTick IRQ and SysTick Timer */ 00872 return (0UL); /* Function successful */ 00873 } 00874 00875 #endif 00876 00877 /*@} end of CMSIS_Core_SysTickFunctions */ 00878 00879 00880 00881 00882 #ifdef __cplusplus 00883 } 00884 #endif 00885 00886 #endif /* __CORE_CM0_H_DEPENDANT */ 00887 00888 #endif /* __CMSIS_GENERIC */ 00889
Generated on Tue Jul 12 2022 16:47:29 by
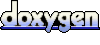