Aded CMSIS5 DSP and NN folder. Needs some work
Embed:
(wiki syntax)
Show/hide line numbers
cmsis_compiler.h
Go to the documentation of this file.
00001 /**************************************************************************//** 00002 * @file cmsis_compiler.h 00003 * @brief CMSIS compiler generic header file 00004 * @version V5.0.4 00005 * @date 10. January 2018 00006 ******************************************************************************/ 00007 /* 00008 * Copyright (c) 2009-2018 Arm Limited. All rights reserved. 00009 * 00010 * SPDX-License-Identifier: Apache-2.0 00011 * 00012 * Licensed under the Apache License, Version 2.0 (the License); you may 00013 * not use this file except in compliance with the License. 00014 * You may obtain a copy of the License at 00015 * 00016 * www.apache.org/licenses/LICENSE-2.0 00017 * 00018 * Unless required by applicable law or agreed to in writing, software 00019 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00020 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00021 * See the License for the specific language governing permissions and 00022 * limitations under the License. 00023 */ 00024 00025 #ifndef __CMSIS_COMPILER_H 00026 #define __CMSIS_COMPILER_H 00027 00028 #include <stdint.h> 00029 00030 /* 00031 * Arm Compiler 4/5 00032 */ 00033 #if defined ( __CC_ARM ) 00034 #include "cmsis_armcc.h" 00035 00036 00037 /* 00038 * Arm Compiler 6 (armclang) 00039 */ 00040 #elif defined (__ARMCC_VERSION) && (__ARMCC_VERSION >= 6010050) 00041 #include "cmsis_armclang.h" 00042 00043 00044 /* 00045 * GNU Compiler 00046 */ 00047 #elif defined ( __GNUC__ ) 00048 #include "cmsis_gcc.h" 00049 00050 00051 /* 00052 * IAR Compiler 00053 */ 00054 #elif defined ( __ICCARM__ ) 00055 #include <cmsis_iccarm.h> 00056 00057 00058 /* 00059 * TI Arm Compiler 00060 */ 00061 #elif defined ( __TI_ARM__ ) 00062 #include <cmsis_ccs.h> 00063 00064 #ifndef __ASM 00065 #define __ASM __asm 00066 #endif 00067 #ifndef __INLINE 00068 #define __INLINE inline 00069 #endif 00070 #ifndef __STATIC_INLINE 00071 #define __STATIC_INLINE static inline 00072 #endif 00073 #ifndef __STATIC_FORCEINLINE 00074 #define __STATIC_FORCEINLINE __STATIC_INLINE 00075 #endif 00076 #ifndef __NO_RETURN 00077 #define __NO_RETURN __attribute__((noreturn)) 00078 #endif 00079 #ifndef __USED 00080 #define __USED __attribute__((used)) 00081 #endif 00082 #ifndef __WEAK 00083 #define __WEAK __attribute__((weak)) 00084 #endif 00085 #ifndef __PACKED 00086 #define __PACKED __attribute__((packed)) 00087 #endif 00088 #ifndef __PACKED_STRUCT 00089 #define __PACKED_STRUCT struct __attribute__((packed)) 00090 #endif 00091 #ifndef __PACKED_UNION 00092 #define __PACKED_UNION union __attribute__((packed)) 00093 #endif 00094 #ifndef __UNALIGNED_UINT32 /* deprecated */ 00095 struct __attribute__((packed)) T_UINT32 { uint32_t v; }; 00096 #define __UNALIGNED_UINT32(x) (((struct T_UINT32 *)(x))->v) 00097 #endif 00098 #ifndef __UNALIGNED_UINT16_WRITE 00099 __PACKED_STRUCT T_UINT16_WRITE { uint16_t v; }; 00100 #define __UNALIGNED_UINT16_WRITE(addr, val) (void)((((struct T_UINT16_WRITE *)(void*)(addr))->v) = (val)) 00101 #endif 00102 #ifndef __UNALIGNED_UINT16_READ 00103 __PACKED_STRUCT T_UINT16_READ { uint16_t v; }; 00104 #define __UNALIGNED_UINT16_READ(addr) (((const struct T_UINT16_READ *)(const void *)(addr))->v) 00105 #endif 00106 #ifndef __UNALIGNED_UINT32_WRITE 00107 __PACKED_STRUCT T_UINT32_WRITE { uint32_t v; }; 00108 #define __UNALIGNED_UINT32_WRITE(addr, val) (void)((((struct T_UINT32_WRITE *)(void *)(addr))->v) = (val)) 00109 #endif 00110 #ifndef __UNALIGNED_UINT32_READ 00111 __PACKED_STRUCT T_UINT32_READ { uint32_t v; }; 00112 #define __UNALIGNED_UINT32_READ(addr) (((const struct T_UINT32_READ *)(const void *)(addr))->v) 00113 #endif 00114 #ifndef __ALIGNED 00115 #define __ALIGNED(x) __attribute__((aligned(x))) 00116 #endif 00117 #ifndef __RESTRICT 00118 #warning No compiler specific solution for __RESTRICT. __RESTRICT is ignored. 00119 #define __RESTRICT 00120 #endif 00121 00122 00123 /* 00124 * TASKING Compiler 00125 */ 00126 #elif defined ( __TASKING__ ) 00127 /* 00128 * The CMSIS functions have been implemented as intrinsics in the compiler. 00129 * Please use "carm -?i" to get an up to date list of all intrinsics, 00130 * Including the CMSIS ones. 00131 */ 00132 00133 #ifndef __ASM 00134 #define __ASM __asm 00135 #endif 00136 #ifndef __INLINE 00137 #define __INLINE inline 00138 #endif 00139 #ifndef __STATIC_INLINE 00140 #define __STATIC_INLINE static inline 00141 #endif 00142 #ifndef __STATIC_FORCEINLINE 00143 #define __STATIC_FORCEINLINE __STATIC_INLINE 00144 #endif 00145 #ifndef __NO_RETURN 00146 #define __NO_RETURN __attribute__((noreturn)) 00147 #endif 00148 #ifndef __USED 00149 #define __USED __attribute__((used)) 00150 #endif 00151 #ifndef __WEAK 00152 #define __WEAK __attribute__((weak)) 00153 #endif 00154 #ifndef __PACKED 00155 #define __PACKED __packed__ 00156 #endif 00157 #ifndef __PACKED_STRUCT 00158 #define __PACKED_STRUCT struct __packed__ 00159 #endif 00160 #ifndef __PACKED_UNION 00161 #define __PACKED_UNION union __packed__ 00162 #endif 00163 #ifndef __UNALIGNED_UINT32 /* deprecated */ 00164 struct __packed__ T_UINT32 { uint32_t v; }; 00165 #define __UNALIGNED_UINT32(x) (((struct T_UINT32 *)(x))->v) 00166 #endif 00167 #ifndef __UNALIGNED_UINT16_WRITE 00168 __PACKED_STRUCT T_UINT16_WRITE { uint16_t v; }; 00169 #define __UNALIGNED_UINT16_WRITE(addr, val) (void)((((struct T_UINT16_WRITE *)(void *)(addr))->v) = (val)) 00170 #endif 00171 #ifndef __UNALIGNED_UINT16_READ 00172 __PACKED_STRUCT T_UINT16_READ { uint16_t v; }; 00173 #define __UNALIGNED_UINT16_READ(addr) (((const struct T_UINT16_READ *)(const void *)(addr))->v) 00174 #endif 00175 #ifndef __UNALIGNED_UINT32_WRITE 00176 __PACKED_STRUCT T_UINT32_WRITE { uint32_t v; }; 00177 #define __UNALIGNED_UINT32_WRITE(addr, val) (void)((((struct T_UINT32_WRITE *)(void *)(addr))->v) = (val)) 00178 #endif 00179 #ifndef __UNALIGNED_UINT32_READ 00180 __PACKED_STRUCT T_UINT32_READ { uint32_t v; }; 00181 #define __UNALIGNED_UINT32_READ(addr) (((const struct T_UINT32_READ *)(const void *)(addr))->v) 00182 #endif 00183 #ifndef __ALIGNED 00184 #define __ALIGNED(x) __align(x) 00185 #endif 00186 #ifndef __RESTRICT 00187 #warning No compiler specific solution for __RESTRICT. __RESTRICT is ignored. 00188 #define __RESTRICT 00189 #endif 00190 00191 00192 /* 00193 * COSMIC Compiler 00194 */ 00195 #elif defined ( __CSMC__ ) 00196 #include <cmsis_csm.h> 00197 00198 #ifndef __ASM 00199 #define __ASM _asm 00200 #endif 00201 #ifndef __INLINE 00202 #define __INLINE inline 00203 #endif 00204 #ifndef __STATIC_INLINE 00205 #define __STATIC_INLINE static inline 00206 #endif 00207 #ifndef __STATIC_FORCEINLINE 00208 #define __STATIC_FORCEINLINE __STATIC_INLINE 00209 #endif 00210 #ifndef __NO_RETURN 00211 // NO RETURN is automatically detected hence no warning here 00212 #define __NO_RETURN 00213 #endif 00214 #ifndef __USED 00215 #warning No compiler specific solution for __USED. __USED is ignored. 00216 #define __USED 00217 #endif 00218 #ifndef __WEAK 00219 #define __WEAK __weak 00220 #endif 00221 #ifndef __PACKED 00222 #define __PACKED @packed 00223 #endif 00224 #ifndef __PACKED_STRUCT 00225 #define __PACKED_STRUCT @packed struct 00226 #endif 00227 #ifndef __PACKED_UNION 00228 #define __PACKED_UNION @packed union 00229 #endif 00230 #ifndef __UNALIGNED_UINT32 /* deprecated */ 00231 @packed struct T_UINT32 { uint32_t v; }; 00232 #define __UNALIGNED_UINT32(x) (((struct T_UINT32 *)(x))->v) 00233 #endif 00234 #ifndef __UNALIGNED_UINT16_WRITE 00235 __PACKED_STRUCT T_UINT16_WRITE { uint16_t v; }; 00236 #define __UNALIGNED_UINT16_WRITE(addr, val) (void)((((struct T_UINT16_WRITE *)(void *)(addr))->v) = (val)) 00237 #endif 00238 #ifndef __UNALIGNED_UINT16_READ 00239 __PACKED_STRUCT T_UINT16_READ { uint16_t v; }; 00240 #define __UNALIGNED_UINT16_READ(addr) (((const struct T_UINT16_READ *)(const void *)(addr))->v) 00241 #endif 00242 #ifndef __UNALIGNED_UINT32_WRITE 00243 __PACKED_STRUCT T_UINT32_WRITE { uint32_t v; }; 00244 #define __UNALIGNED_UINT32_WRITE(addr, val) (void)((((struct T_UINT32_WRITE *)(void *)(addr))->v) = (val)) 00245 #endif 00246 #ifndef __UNALIGNED_UINT32_READ 00247 __PACKED_STRUCT T_UINT32_READ { uint32_t v; }; 00248 #define __UNALIGNED_UINT32_READ(addr) (((const struct T_UINT32_READ *)(const void *)(addr))->v) 00249 #endif 00250 #ifndef __ALIGNED 00251 #warning No compiler specific solution for __ALIGNED. __ALIGNED is ignored. 00252 #define __ALIGNED(x) 00253 #endif 00254 #ifndef __RESTRICT 00255 #warning No compiler specific solution for __RESTRICT. __RESTRICT is ignored. 00256 #define __RESTRICT 00257 #endif 00258 00259 00260 #else 00261 #error Unknown compiler. 00262 #endif 00263 00264 00265 #endif /* __CMSIS_COMPILER_H */ 00266 00267
Generated on Tue Jul 12 2022 16:47:28 by
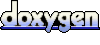