Aded CMSIS5 DSP and NN folder. Needs some work
Embed:
(wiki syntax)
Show/hide line numbers
cmsis_armcc.h
Go to the documentation of this file.
00001 /**************************************************************************//** 00002 * @file cmsis_armcc.h 00003 * @brief CMSIS compiler ARMCC (Arm Compiler 5) header file 00004 * @version V5.0.4 00005 * @date 10. January 2018 00006 ******************************************************************************/ 00007 /* 00008 * Copyright (c) 2009-2018 Arm Limited. All rights reserved. 00009 * 00010 * SPDX-License-Identifier: Apache-2.0 00011 * 00012 * Licensed under the Apache License, Version 2.0 (the License); you may 00013 * not use this file except in compliance with the License. 00014 * You may obtain a copy of the License at 00015 * 00016 * www.apache.org/licenses/LICENSE-2.0 00017 * 00018 * Unless required by applicable law or agreed to in writing, software 00019 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00020 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00021 * See the License for the specific language governing permissions and 00022 * limitations under the License. 00023 */ 00024 00025 #ifndef __CMSIS_ARMCC_H 00026 #define __CMSIS_ARMCC_H 00027 00028 00029 #if defined(__ARMCC_VERSION) && (__ARMCC_VERSION < 400677) 00030 #error "Please use Arm Compiler Toolchain V4.0.677 or later!" 00031 #endif 00032 00033 /* CMSIS compiler control architecture macros */ 00034 #if ((defined (__TARGET_ARCH_6_M ) && (__TARGET_ARCH_6_M == 1)) || \ 00035 (defined (__TARGET_ARCH_6S_M ) && (__TARGET_ARCH_6S_M == 1)) ) 00036 #define __ARM_ARCH_6M__ 1 00037 #endif 00038 00039 #if (defined (__TARGET_ARCH_7_M ) && (__TARGET_ARCH_7_M == 1)) 00040 #define __ARM_ARCH_7M__ 1 00041 #endif 00042 00043 #if (defined (__TARGET_ARCH_7E_M) && (__TARGET_ARCH_7E_M == 1)) 00044 #define __ARM_ARCH_7EM__ 1 00045 #endif 00046 00047 /* __ARM_ARCH_8M_BASE__ not applicable */ 00048 /* __ARM_ARCH_8M_MAIN__ not applicable */ 00049 00050 00051 /* CMSIS compiler specific defines */ 00052 #ifndef __ASM 00053 #define __ASM __asm 00054 #endif 00055 #ifndef __INLINE 00056 #define __INLINE __inline 00057 #endif 00058 #ifndef __STATIC_INLINE 00059 #define __STATIC_INLINE static __inline 00060 #endif 00061 #ifndef __STATIC_FORCEINLINE 00062 #define __STATIC_FORCEINLINE static __forceinline 00063 #endif 00064 #ifndef __NO_RETURN 00065 #define __NO_RETURN __declspec(noreturn) 00066 #endif 00067 #ifndef __USED 00068 #define __USED __attribute__((used)) 00069 #endif 00070 #ifndef __WEAK 00071 #define __WEAK __attribute__((weak)) 00072 #endif 00073 #ifndef __PACKED 00074 #define __PACKED __attribute__((packed)) 00075 #endif 00076 #ifndef __PACKED_STRUCT 00077 #define __PACKED_STRUCT __packed struct 00078 #endif 00079 #ifndef __PACKED_UNION 00080 #define __PACKED_UNION __packed union 00081 #endif 00082 #ifndef __UNALIGNED_UINT32 /* deprecated */ 00083 #define __UNALIGNED_UINT32(x) (*((__packed uint32_t *)(x))) 00084 #endif 00085 #ifndef __UNALIGNED_UINT16_WRITE 00086 #define __UNALIGNED_UINT16_WRITE(addr, val) ((*((__packed uint16_t *)(addr))) = (val)) 00087 #endif 00088 #ifndef __UNALIGNED_UINT16_READ 00089 #define __UNALIGNED_UINT16_READ(addr) (*((const __packed uint16_t *)(addr))) 00090 #endif 00091 #ifndef __UNALIGNED_UINT32_WRITE 00092 #define __UNALIGNED_UINT32_WRITE(addr, val) ((*((__packed uint32_t *)(addr))) = (val)) 00093 #endif 00094 #ifndef __UNALIGNED_UINT32_READ 00095 #define __UNALIGNED_UINT32_READ(addr) (*((const __packed uint32_t *)(addr))) 00096 #endif 00097 #ifndef __ALIGNED 00098 #define __ALIGNED(x) __attribute__((aligned(x))) 00099 #endif 00100 #ifndef __RESTRICT 00101 #define __RESTRICT __restrict 00102 #endif 00103 00104 /* ########################### Core Function Access ########################### */ 00105 /** \ingroup CMSIS_Core_FunctionInterface 00106 \defgroup CMSIS_Core_RegAccFunctions CMSIS Core Register Access Functions 00107 @{ 00108 */ 00109 00110 /** 00111 \brief Enable IRQ Interrupts 00112 \details Enables IRQ interrupts by clearing the I-bit in the CPSR. 00113 Can only be executed in Privileged modes. 00114 */ 00115 /* intrinsic void __enable_irq(); */ 00116 00117 00118 /** 00119 \brief Disable IRQ Interrupts 00120 \details Disables IRQ interrupts by setting the I-bit in the CPSR. 00121 Can only be executed in Privileged modes. 00122 */ 00123 /* intrinsic void __disable_irq(); */ 00124 00125 /** 00126 \brief Get Control Register 00127 \details Returns the content of the Control Register. 00128 \return Control Register value 00129 */ 00130 __STATIC_INLINE uint32_t __get_CONTROL(void) 00131 { 00132 register uint32_t __regControl __ASM("control"); 00133 return(__regControl); 00134 } 00135 00136 00137 /** 00138 \brief Set Control Register 00139 \details Writes the given value to the Control Register. 00140 \param [in] control Control Register value to set 00141 */ 00142 __STATIC_INLINE void __set_CONTROL(uint32_t control) 00143 { 00144 register uint32_t __regControl __ASM("control"); 00145 __regControl = control; 00146 } 00147 00148 00149 /** 00150 \brief Get IPSR Register 00151 \details Returns the content of the IPSR Register. 00152 \return IPSR Register value 00153 */ 00154 __STATIC_INLINE uint32_t __get_IPSR(void) 00155 { 00156 register uint32_t __regIPSR __ASM("ipsr"); 00157 return(__regIPSR); 00158 } 00159 00160 00161 /** 00162 \brief Get APSR Register 00163 \details Returns the content of the APSR Register. 00164 \return APSR Register value 00165 */ 00166 __STATIC_INLINE uint32_t __get_APSR(void) 00167 { 00168 register uint32_t __regAPSR __ASM("apsr"); 00169 return(__regAPSR); 00170 } 00171 00172 00173 /** 00174 \brief Get xPSR Register 00175 \details Returns the content of the xPSR Register. 00176 \return xPSR Register value 00177 */ 00178 __STATIC_INLINE uint32_t __get_xPSR(void) 00179 { 00180 register uint32_t __regXPSR __ASM("xpsr"); 00181 return(__regXPSR); 00182 } 00183 00184 00185 /** 00186 \brief Get Process Stack Pointer 00187 \details Returns the current value of the Process Stack Pointer (PSP). 00188 \return PSP Register value 00189 */ 00190 __STATIC_INLINE uint32_t __get_PSP(void) 00191 { 00192 register uint32_t __regProcessStackPointer __ASM("psp"); 00193 return(__regProcessStackPointer); 00194 } 00195 00196 00197 /** 00198 \brief Set Process Stack Pointer 00199 \details Assigns the given value to the Process Stack Pointer (PSP). 00200 \param [in] topOfProcStack Process Stack Pointer value to set 00201 */ 00202 __STATIC_INLINE void __set_PSP(uint32_t topOfProcStack) 00203 { 00204 register uint32_t __regProcessStackPointer __ASM("psp"); 00205 __regProcessStackPointer = topOfProcStack; 00206 } 00207 00208 00209 /** 00210 \brief Get Main Stack Pointer 00211 \details Returns the current value of the Main Stack Pointer (MSP). 00212 \return MSP Register value 00213 */ 00214 __STATIC_INLINE uint32_t __get_MSP(void) 00215 { 00216 register uint32_t __regMainStackPointer __ASM("msp"); 00217 return(__regMainStackPointer); 00218 } 00219 00220 00221 /** 00222 \brief Set Main Stack Pointer 00223 \details Assigns the given value to the Main Stack Pointer (MSP). 00224 \param [in] topOfMainStack Main Stack Pointer value to set 00225 */ 00226 __STATIC_INLINE void __set_MSP(uint32_t topOfMainStack) 00227 { 00228 register uint32_t __regMainStackPointer __ASM("msp"); 00229 __regMainStackPointer = topOfMainStack; 00230 } 00231 00232 00233 /** 00234 \brief Get Priority Mask 00235 \details Returns the current state of the priority mask bit from the Priority Mask Register. 00236 \return Priority Mask value 00237 */ 00238 __STATIC_INLINE uint32_t __get_PRIMASK(void) 00239 { 00240 register uint32_t __regPriMask __ASM("primask"); 00241 return(__regPriMask); 00242 } 00243 00244 00245 /** 00246 \brief Set Priority Mask 00247 \details Assigns the given value to the Priority Mask Register. 00248 \param [in] priMask Priority Mask 00249 */ 00250 __STATIC_INLINE void __set_PRIMASK(uint32_t priMask) 00251 { 00252 register uint32_t __regPriMask __ASM("primask"); 00253 __regPriMask = (priMask); 00254 } 00255 00256 00257 #if ((defined (__ARM_ARCH_7M__ ) && (__ARM_ARCH_7M__ == 1)) || \ 00258 (defined (__ARM_ARCH_7EM__) && (__ARM_ARCH_7EM__ == 1)) ) 00259 00260 /** 00261 \brief Enable FIQ 00262 \details Enables FIQ interrupts by clearing the F-bit in the CPSR. 00263 Can only be executed in Privileged modes. 00264 */ 00265 #define __enable_fault_irq __enable_fiq 00266 00267 00268 /** 00269 \brief Disable FIQ 00270 \details Disables FIQ interrupts by setting the F-bit in the CPSR. 00271 Can only be executed in Privileged modes. 00272 */ 00273 #define __disable_fault_irq __disable_fiq 00274 00275 00276 /** 00277 \brief Get Base Priority 00278 \details Returns the current value of the Base Priority register. 00279 \return Base Priority register value 00280 */ 00281 __STATIC_INLINE uint32_t __get_BASEPRI(void) 00282 { 00283 register uint32_t __regBasePri __ASM("basepri"); 00284 return(__regBasePri); 00285 } 00286 00287 00288 /** 00289 \brief Set Base Priority 00290 \details Assigns the given value to the Base Priority register. 00291 \param [in] basePri Base Priority value to set 00292 */ 00293 __STATIC_INLINE void __set_BASEPRI(uint32_t basePri) 00294 { 00295 register uint32_t __regBasePri __ASM("basepri"); 00296 __regBasePri = (basePri & 0xFFU); 00297 } 00298 00299 00300 /** 00301 \brief Set Base Priority with condition 00302 \details Assigns the given value to the Base Priority register only if BASEPRI masking is disabled, 00303 or the new value increases the BASEPRI priority level. 00304 \param [in] basePri Base Priority value to set 00305 */ 00306 __STATIC_INLINE void __set_BASEPRI_MAX(uint32_t basePri) 00307 { 00308 register uint32_t __regBasePriMax __ASM("basepri_max"); 00309 __regBasePriMax = (basePri & 0xFFU); 00310 } 00311 00312 00313 /** 00314 \brief Get Fault Mask 00315 \details Returns the current value of the Fault Mask register. 00316 \return Fault Mask register value 00317 */ 00318 __STATIC_INLINE uint32_t __get_FAULTMASK(void) 00319 { 00320 register uint32_t __regFaultMask __ASM("faultmask"); 00321 return(__regFaultMask); 00322 } 00323 00324 00325 /** 00326 \brief Set Fault Mask 00327 \details Assigns the given value to the Fault Mask register. 00328 \param [in] faultMask Fault Mask value to set 00329 */ 00330 __STATIC_INLINE void __set_FAULTMASK(uint32_t faultMask) 00331 { 00332 register uint32_t __regFaultMask __ASM("faultmask"); 00333 __regFaultMask = (faultMask & (uint32_t)1U); 00334 } 00335 00336 #endif /* ((defined (__ARM_ARCH_7M__ ) && (__ARM_ARCH_7M__ == 1)) || \ 00337 (defined (__ARM_ARCH_7EM__) && (__ARM_ARCH_7EM__ == 1)) ) */ 00338 00339 00340 #if ((defined (__ARM_ARCH_7EM__) && (__ARM_ARCH_7EM__ == 1)) ) 00341 00342 /** 00343 \brief Get FPSCR 00344 \details Returns the current value of the Floating Point Status/Control register. 00345 \return Floating Point Status/Control register value 00346 */ 00347 __STATIC_INLINE uint32_t __get_FPSCR(void) 00348 { 00349 #if ((defined (__FPU_PRESENT) && (__FPU_PRESENT == 1U)) && \ 00350 (defined (__FPU_USED ) && (__FPU_USED == 1U)) ) 00351 register uint32_t __regfpscr __ASM("fpscr"); 00352 return(__regfpscr); 00353 #else 00354 return(0U); 00355 #endif 00356 } 00357 00358 00359 /** 00360 \brief Set FPSCR 00361 \details Assigns the given value to the Floating Point Status/Control register. 00362 \param [in] fpscr Floating Point Status/Control value to set 00363 */ 00364 __STATIC_INLINE void __set_FPSCR(uint32_t fpscr) 00365 { 00366 #if ((defined (__FPU_PRESENT) && (__FPU_PRESENT == 1U)) && \ 00367 (defined (__FPU_USED ) && (__FPU_USED == 1U)) ) 00368 register uint32_t __regfpscr __ASM("fpscr"); 00369 __regfpscr = (fpscr); 00370 #else 00371 (void)fpscr; 00372 #endif 00373 } 00374 00375 #endif /* ((defined (__ARM_ARCH_7EM__) && (__ARM_ARCH_7EM__ == 1)) ) */ 00376 00377 00378 00379 /*@} end of CMSIS_Core_RegAccFunctions */ 00380 00381 00382 /* ########################## Core Instruction Access ######################### */ 00383 /** \defgroup CMSIS_Core_InstructionInterface CMSIS Core Instruction Interface 00384 Access to dedicated instructions 00385 @{ 00386 */ 00387 00388 /** 00389 \brief No Operation 00390 \details No Operation does nothing. This instruction can be used for code alignment purposes. 00391 */ 00392 #define __NOP __nop 00393 00394 00395 /** 00396 \brief Wait For Interrupt 00397 \details Wait For Interrupt is a hint instruction that suspends execution until one of a number of events occurs. 00398 */ 00399 #define __WFI __wfi 00400 00401 00402 /** 00403 \brief Wait For Event 00404 \details Wait For Event is a hint instruction that permits the processor to enter 00405 a low-power state until one of a number of events occurs. 00406 */ 00407 #define __WFE __wfe 00408 00409 00410 /** 00411 \brief Send Event 00412 \details Send Event is a hint instruction. It causes an event to be signaled to the CPU. 00413 */ 00414 #define __SEV __sev 00415 00416 00417 /** 00418 \brief Instruction Synchronization Barrier 00419 \details Instruction Synchronization Barrier flushes the pipeline in the processor, 00420 so that all instructions following the ISB are fetched from cache or memory, 00421 after the instruction has been completed. 00422 */ 00423 #define __ISB() do {\ 00424 __schedule_barrier();\ 00425 __isb(0xF);\ 00426 __schedule_barrier();\ 00427 } while (0U) 00428 00429 /** 00430 \brief Data Synchronization Barrier 00431 \details Acts as a special kind of Data Memory Barrier. 00432 It completes when all explicit memory accesses before this instruction complete. 00433 */ 00434 #define __DSB() do {\ 00435 __schedule_barrier();\ 00436 __dsb(0xF);\ 00437 __schedule_barrier();\ 00438 } while (0U) 00439 00440 /** 00441 \brief Data Memory Barrier 00442 \details Ensures the apparent order of the explicit memory operations before 00443 and after the instruction, without ensuring their completion. 00444 */ 00445 #define __DMB() do {\ 00446 __schedule_barrier();\ 00447 __dmb(0xF);\ 00448 __schedule_barrier();\ 00449 } while (0U) 00450 00451 00452 /** 00453 \brief Reverse byte order (32 bit) 00454 \details Reverses the byte order in unsigned integer value. For example, 0x12345678 becomes 0x78563412. 00455 \param [in] value Value to reverse 00456 \return Reversed value 00457 */ 00458 #define __REV __rev 00459 00460 00461 /** 00462 \brief Reverse byte order (16 bit) 00463 \details Reverses the byte order within each halfword of a word. For example, 0x12345678 becomes 0x34127856. 00464 \param [in] value Value to reverse 00465 \return Reversed value 00466 */ 00467 #ifndef __NO_EMBEDDED_ASM 00468 __attribute__((section(".rev16_text"))) __STATIC_INLINE __ASM uint32_t __REV16(uint32_t value) 00469 { 00470 rev16 r0, r0 00471 bx lr 00472 } 00473 #endif 00474 00475 00476 /** 00477 \brief Reverse byte order (16 bit) 00478 \details Reverses the byte order in a 16-bit value and returns the signed 16-bit result. For example, 0x0080 becomes 0x8000. 00479 \param [in] value Value to reverse 00480 \return Reversed value 00481 */ 00482 #ifndef __NO_EMBEDDED_ASM 00483 __attribute__((section(".revsh_text"))) __STATIC_INLINE __ASM int16_t __REVSH(int16_t value) 00484 { 00485 revsh r0, r0 00486 bx lr 00487 } 00488 #endif 00489 00490 00491 /** 00492 \brief Rotate Right in unsigned value (32 bit) 00493 \details Rotate Right (immediate) provides the value of the contents of a register rotated by a variable number of bits. 00494 \param [in] op1 Value to rotate 00495 \param [in] op2 Number of Bits to rotate 00496 \return Rotated value 00497 */ 00498 #define __ROR __ror 00499 00500 00501 /** 00502 \brief Breakpoint 00503 \details Causes the processor to enter Debug state. 00504 Debug tools can use this to investigate system state when the instruction at a particular address is reached. 00505 \param [in] value is ignored by the processor. 00506 If required, a debugger can use it to store additional information about the breakpoint. 00507 */ 00508 #define __BKPT(value) __breakpoint(value) 00509 00510 00511 /** 00512 \brief Reverse bit order of value 00513 \details Reverses the bit order of the given value. 00514 \param [in] value Value to reverse 00515 \return Reversed value 00516 */ 00517 #if ((defined (__ARM_ARCH_7M__ ) && (__ARM_ARCH_7M__ == 1)) || \ 00518 (defined (__ARM_ARCH_7EM__) && (__ARM_ARCH_7EM__ == 1)) ) 00519 #define __RBIT __rbit 00520 #else 00521 __attribute__((always_inline)) __STATIC_INLINE uint32_t __RBIT(uint32_t value) 00522 { 00523 uint32_t result; 00524 uint32_t s = (4U /*sizeof(v)*/ * 8U) - 1U; /* extra shift needed at end */ 00525 00526 result = value; /* r will be reversed bits of v; first get LSB of v */ 00527 for (value >>= 1U; value != 0U; value >>= 1U) 00528 { 00529 result <<= 1U; 00530 result |= value & 1U; 00531 s--; 00532 } 00533 result <<= s; /* shift when v's highest bits are zero */ 00534 return result; 00535 } 00536 #endif 00537 00538 00539 /** 00540 \brief Count leading zeros 00541 \details Counts the number of leading zeros of a data value. 00542 \param [in] value Value to count the leading zeros 00543 \return number of leading zeros in value 00544 */ 00545 #define __CLZ __clz 00546 00547 00548 #if ((defined (__ARM_ARCH_7M__ ) && (__ARM_ARCH_7M__ == 1)) || \ 00549 (defined (__ARM_ARCH_7EM__) && (__ARM_ARCH_7EM__ == 1)) ) 00550 00551 /** 00552 \brief LDR Exclusive (8 bit) 00553 \details Executes a exclusive LDR instruction for 8 bit value. 00554 \param [in] ptr Pointer to data 00555 \return value of type uint8_t at (*ptr) 00556 */ 00557 #if defined(__ARMCC_VERSION) && (__ARMCC_VERSION < 5060020) 00558 #define __LDREXB(ptr) ((uint8_t ) __ldrex(ptr)) 00559 #else 00560 #define __LDREXB(ptr) _Pragma("push") _Pragma("diag_suppress 3731") ((uint8_t ) __ldrex(ptr)) _Pragma("pop") 00561 #endif 00562 00563 00564 /** 00565 \brief LDR Exclusive (16 bit) 00566 \details Executes a exclusive LDR instruction for 16 bit values. 00567 \param [in] ptr Pointer to data 00568 \return value of type uint16_t at (*ptr) 00569 */ 00570 #if defined(__ARMCC_VERSION) && (__ARMCC_VERSION < 5060020) 00571 #define __LDREXH(ptr) ((uint16_t) __ldrex(ptr)) 00572 #else 00573 #define __LDREXH(ptr) _Pragma("push") _Pragma("diag_suppress 3731") ((uint16_t) __ldrex(ptr)) _Pragma("pop") 00574 #endif 00575 00576 00577 /** 00578 \brief LDR Exclusive (32 bit) 00579 \details Executes a exclusive LDR instruction for 32 bit values. 00580 \param [in] ptr Pointer to data 00581 \return value of type uint32_t at (*ptr) 00582 */ 00583 #if defined(__ARMCC_VERSION) && (__ARMCC_VERSION < 5060020) 00584 #define __LDREXW(ptr) ((uint32_t ) __ldrex(ptr)) 00585 #else 00586 #define __LDREXW(ptr) _Pragma("push") _Pragma("diag_suppress 3731") ((uint32_t ) __ldrex(ptr)) _Pragma("pop") 00587 #endif 00588 00589 00590 /** 00591 \brief STR Exclusive (8 bit) 00592 \details Executes a exclusive STR instruction for 8 bit values. 00593 \param [in] value Value to store 00594 \param [in] ptr Pointer to location 00595 \return 0 Function succeeded 00596 \return 1 Function failed 00597 */ 00598 #if defined(__ARMCC_VERSION) && (__ARMCC_VERSION < 5060020) 00599 #define __STREXB(value, ptr) __strex(value, ptr) 00600 #else 00601 #define __STREXB(value, ptr) _Pragma("push") _Pragma("diag_suppress 3731") __strex(value, ptr) _Pragma("pop") 00602 #endif 00603 00604 00605 /** 00606 \brief STR Exclusive (16 bit) 00607 \details Executes a exclusive STR instruction for 16 bit values. 00608 \param [in] value Value to store 00609 \param [in] ptr Pointer to location 00610 \return 0 Function succeeded 00611 \return 1 Function failed 00612 */ 00613 #if defined(__ARMCC_VERSION) && (__ARMCC_VERSION < 5060020) 00614 #define __STREXH(value, ptr) __strex(value, ptr) 00615 #else 00616 #define __STREXH(value, ptr) _Pragma("push") _Pragma("diag_suppress 3731") __strex(value, ptr) _Pragma("pop") 00617 #endif 00618 00619 00620 /** 00621 \brief STR Exclusive (32 bit) 00622 \details Executes a exclusive STR instruction for 32 bit values. 00623 \param [in] value Value to store 00624 \param [in] ptr Pointer to location 00625 \return 0 Function succeeded 00626 \return 1 Function failed 00627 */ 00628 #if defined(__ARMCC_VERSION) && (__ARMCC_VERSION < 5060020) 00629 #define __STREXW(value, ptr) __strex(value, ptr) 00630 #else 00631 #define __STREXW(value, ptr) _Pragma("push") _Pragma("diag_suppress 3731") __strex(value, ptr) _Pragma("pop") 00632 #endif 00633 00634 00635 /** 00636 \brief Remove the exclusive lock 00637 \details Removes the exclusive lock which is created by LDREX. 00638 */ 00639 #define __CLREX __clrex 00640 00641 00642 /** 00643 \brief Signed Saturate 00644 \details Saturates a signed value. 00645 \param [in] value Value to be saturated 00646 \param [in] sat Bit position to saturate to (1..32) 00647 \return Saturated value 00648 */ 00649 #define __SSAT __ssat 00650 00651 00652 /** 00653 \brief Unsigned Saturate 00654 \details Saturates an unsigned value. 00655 \param [in] value Value to be saturated 00656 \param [in] sat Bit position to saturate to (0..31) 00657 \return Saturated value 00658 */ 00659 #define __USAT __usat 00660 00661 00662 /** 00663 \brief Rotate Right with Extend (32 bit) 00664 \details Moves each bit of a bitstring right by one bit. 00665 The carry input is shifted in at the left end of the bitstring. 00666 \param [in] value Value to rotate 00667 \return Rotated value 00668 */ 00669 #ifndef __NO_EMBEDDED_ASM 00670 __attribute__((section(".rrx_text"))) __STATIC_INLINE __ASM uint32_t __RRX(uint32_t value) 00671 { 00672 rrx r0, r0 00673 bx lr 00674 } 00675 #endif 00676 00677 00678 /** 00679 \brief LDRT Unprivileged (8 bit) 00680 \details Executes a Unprivileged LDRT instruction for 8 bit value. 00681 \param [in] ptr Pointer to data 00682 \return value of type uint8_t at (*ptr) 00683 */ 00684 #define __LDRBT(ptr) ((uint8_t ) __ldrt(ptr)) 00685 00686 00687 /** 00688 \brief LDRT Unprivileged (16 bit) 00689 \details Executes a Unprivileged LDRT instruction for 16 bit values. 00690 \param [in] ptr Pointer to data 00691 \return value of type uint16_t at (*ptr) 00692 */ 00693 #define __LDRHT(ptr) ((uint16_t) __ldrt(ptr)) 00694 00695 00696 /** 00697 \brief LDRT Unprivileged (32 bit) 00698 \details Executes a Unprivileged LDRT instruction for 32 bit values. 00699 \param [in] ptr Pointer to data 00700 \return value of type uint32_t at (*ptr) 00701 */ 00702 #define __LDRT(ptr) ((uint32_t ) __ldrt(ptr)) 00703 00704 00705 /** 00706 \brief STRT Unprivileged (8 bit) 00707 \details Executes a Unprivileged STRT instruction for 8 bit values. 00708 \param [in] value Value to store 00709 \param [in] ptr Pointer to location 00710 */ 00711 #define __STRBT(value, ptr) __strt(value, ptr) 00712 00713 00714 /** 00715 \brief STRT Unprivileged (16 bit) 00716 \details Executes a Unprivileged STRT instruction for 16 bit values. 00717 \param [in] value Value to store 00718 \param [in] ptr Pointer to location 00719 */ 00720 #define __STRHT(value, ptr) __strt(value, ptr) 00721 00722 00723 /** 00724 \brief STRT Unprivileged (32 bit) 00725 \details Executes a Unprivileged STRT instruction for 32 bit values. 00726 \param [in] value Value to store 00727 \param [in] ptr Pointer to location 00728 */ 00729 #define __STRT(value, ptr) __strt(value, ptr) 00730 00731 #else /* ((defined (__ARM_ARCH_7M__ ) && (__ARM_ARCH_7M__ == 1)) || \ 00732 (defined (__ARM_ARCH_7EM__) && (__ARM_ARCH_7EM__ == 1)) ) */ 00733 00734 /** 00735 \brief Signed Saturate 00736 \details Saturates a signed value. 00737 \param [in] value Value to be saturated 00738 \param [in] sat Bit position to saturate to (1..32) 00739 \return Saturated value 00740 */ 00741 __attribute__((always_inline)) __STATIC_INLINE int32_t __SSAT(int32_t val, uint32_t sat) 00742 { 00743 if ((sat >= 1U) && (sat <= 32U)) 00744 { 00745 const int32_t max = (int32_t)((1U << (sat - 1U)) - 1U); 00746 const int32_t min = -1 - max ; 00747 if (val > max) 00748 { 00749 return max; 00750 } 00751 else if (val < min) 00752 { 00753 return min; 00754 } 00755 } 00756 return val; 00757 } 00758 00759 /** 00760 \brief Unsigned Saturate 00761 \details Saturates an unsigned value. 00762 \param [in] value Value to be saturated 00763 \param [in] sat Bit position to saturate to (0..31) 00764 \return Saturated value 00765 */ 00766 __attribute__((always_inline)) __STATIC_INLINE uint32_t __USAT(int32_t val, uint32_t sat) 00767 { 00768 if (sat <= 31U) 00769 { 00770 const uint32_t max = ((1U << sat) - 1U); 00771 if (val > (int32_t)max) 00772 { 00773 return max; 00774 } 00775 else if (val < 0) 00776 { 00777 return 0U; 00778 } 00779 } 00780 return (uint32_t)val; 00781 } 00782 00783 #endif /* ((defined (__ARM_ARCH_7M__ ) && (__ARM_ARCH_7M__ == 1)) || \ 00784 (defined (__ARM_ARCH_7EM__) && (__ARM_ARCH_7EM__ == 1)) ) */ 00785 00786 /*@}*/ /* end of group CMSIS_Core_InstructionInterface */ 00787 00788 00789 /* ################### Compiler specific Intrinsics ########################### */ 00790 /** \defgroup CMSIS_SIMD_intrinsics CMSIS SIMD Intrinsics 00791 Access to dedicated SIMD instructions 00792 @{ 00793 */ 00794 00795 #if ((defined (__ARM_ARCH_7EM__) && (__ARM_ARCH_7EM__ == 1)) ) 00796 00797 #define __SADD8 __sadd8 00798 #define __QADD8 __qadd8 00799 #define __SHADD8 __shadd8 00800 #define __UADD8 __uadd8 00801 #define __UQADD8 __uqadd8 00802 #define __UHADD8 __uhadd8 00803 #define __SSUB8 __ssub8 00804 #define __QSUB8 __qsub8 00805 #define __SHSUB8 __shsub8 00806 #define __USUB8 __usub8 00807 #define __UQSUB8 __uqsub8 00808 #define __UHSUB8 __uhsub8 00809 #define __SADD16 __sadd16 00810 #define __QADD16 __qadd16 00811 #define __SHADD16 __shadd16 00812 #define __UADD16 __uadd16 00813 #define __UQADD16 __uqadd16 00814 #define __UHADD16 __uhadd16 00815 #define __SSUB16 __ssub16 00816 #define __QSUB16 __qsub16 00817 #define __SHSUB16 __shsub16 00818 #define __USUB16 __usub16 00819 #define __UQSUB16 __uqsub16 00820 #define __UHSUB16 __uhsub16 00821 #define __SASX __sasx 00822 #define __QASX __qasx 00823 #define __SHASX __shasx 00824 #define __UASX __uasx 00825 #define __UQASX __uqasx 00826 #define __UHASX __uhasx 00827 #define __SSAX __ssax 00828 #define __QSAX __qsax 00829 #define __SHSAX __shsax 00830 #define __USAX __usax 00831 #define __UQSAX __uqsax 00832 #define __UHSAX __uhsax 00833 #define __USAD8 __usad8 00834 #define __USADA8 __usada8 00835 #define __SSAT16 __ssat16 00836 #define __USAT16 __usat16 00837 #define __UXTB16 __uxtb16 00838 #define __UXTAB16 __uxtab16 00839 #define __SXTB16 __sxtb16 00840 #define __SXTAB16 __sxtab16 00841 #define __SMUAD __smuad 00842 #define __SMUADX __smuadx 00843 #define __SMLAD __smlad 00844 #define __SMLADX __smladx 00845 #define __SMLALD __smlald 00846 #define __SMLALDX __smlaldx 00847 #define __SMUSD __smusd 00848 #define __SMUSDX __smusdx 00849 #define __SMLSD __smlsd 00850 #define __SMLSDX __smlsdx 00851 #define __SMLSLD __smlsld 00852 #define __SMLSLDX __smlsldx 00853 #define __SEL __sel 00854 #define __QADD __qadd 00855 #define __QSUB __qsub 00856 00857 #define __PKHBT(ARG1,ARG2,ARG3) ( ((((uint32_t)(ARG1)) ) & 0x0000FFFFUL) | \ 00858 ((((uint32_t)(ARG2)) << (ARG3)) & 0xFFFF0000UL) ) 00859 00860 #define __PKHTB(ARG1,ARG2,ARG3) ( ((((uint32_t)(ARG1)) ) & 0xFFFF0000UL) | \ 00861 ((((uint32_t)(ARG2)) >> (ARG3)) & 0x0000FFFFUL) ) 00862 00863 #define __SMMLA(ARG1,ARG2,ARG3) ( (int32_t)((((int64_t)(ARG1) * (ARG2)) + \ 00864 ((int64_t)(ARG3) << 32U) ) >> 32U)) 00865 00866 #endif /* ((defined (__ARM_ARCH_7EM__) && (__ARM_ARCH_7EM__ == 1)) ) */ 00867 /*@} end of group CMSIS_SIMD_intrinsics */ 00868 00869 00870 #endif /* __CMSIS_ARMCC_H */ 00871
Generated on Tue Jul 12 2022 16:47:28 by
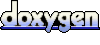