Aded CMSIS5 DSP and NN folder. Needs some work
Embed:
(wiki syntax)
Show/hide line numbers
arm_sub_q15.c
00001 /* ---------------------------------------------------------------------- 00002 * Project: CMSIS DSP Library 00003 * Title: arm_sub_q15.c 00004 * Description: Q15 vector subtraction 00005 * 00006 * $Date: 27. January 2017 00007 * $Revision: V.1.5.1 00008 * 00009 * Target Processor: Cortex-M cores 00010 * -------------------------------------------------------------------- */ 00011 /* 00012 * Copyright (C) 2010-2017 ARM Limited or its affiliates. All rights reserved. 00013 * 00014 * SPDX-License-Identifier: Apache-2.0 00015 * 00016 * Licensed under the Apache License, Version 2.0 (the License); you may 00017 * not use this file except in compliance with the License. 00018 * You may obtain a copy of the License at 00019 * 00020 * www.apache.org/licenses/LICENSE-2.0 00021 * 00022 * Unless required by applicable law or agreed to in writing, software 00023 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00024 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00025 * See the License for the specific language governing permissions and 00026 * limitations under the License. 00027 */ 00028 00029 #include "arm_math.h" 00030 00031 /** 00032 * @ingroup groupMath 00033 */ 00034 00035 /** 00036 * @addtogroup BasicSub 00037 * @{ 00038 */ 00039 00040 /** 00041 * @brief Q15 vector subtraction. 00042 * @param[in] *pSrcA points to the first input vector 00043 * @param[in] *pSrcB points to the second input vector 00044 * @param[out] *pDst points to the output vector 00045 * @param[in] blockSize number of samples in each vector 00046 * @return none. 00047 * 00048 * <b>Scaling and Overflow Behavior:</b> 00049 * \par 00050 * The function uses saturating arithmetic. 00051 * Results outside of the allowable Q15 range [0x8000 0x7FFF] will be saturated. 00052 */ 00053 00054 void arm_sub_q15( 00055 q15_t * pSrcA, 00056 q15_t * pSrcB, 00057 q15_t * pDst, 00058 uint32_t blockSize) 00059 { 00060 uint32_t blkCnt; /* loop counter */ 00061 00062 00063 #if defined (ARM_MATH_DSP) 00064 00065 /* Run the below code for Cortex-M4 and Cortex-M3 */ 00066 q31_t inA1, inA2; 00067 q31_t inB1, inB2; 00068 00069 /*loop Unrolling */ 00070 blkCnt = blockSize >> 2U; 00071 00072 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00073 ** a second loop below computes the remaining 1 to 3 samples. */ 00074 while (blkCnt > 0U) 00075 { 00076 /* C = A - B */ 00077 /* Subtract and then store the results in the destination buffer two samples at a time. */ 00078 inA1 = *__SIMD32(pSrcA)++; 00079 inA2 = *__SIMD32(pSrcA)++; 00080 inB1 = *__SIMD32(pSrcB)++; 00081 inB2 = *__SIMD32(pSrcB)++; 00082 00083 *__SIMD32(pDst)++ = __QSUB16(inA1, inB1); 00084 *__SIMD32(pDst)++ = __QSUB16(inA2, inB2); 00085 00086 /* Decrement the loop counter */ 00087 blkCnt--; 00088 } 00089 00090 /* If the blockSize is not a multiple of 4, compute any remaining output samples here. 00091 ** No loop unrolling is used. */ 00092 blkCnt = blockSize % 0x4U; 00093 00094 while (blkCnt > 0U) 00095 { 00096 /* C = A - B */ 00097 /* Subtract and then store the result in the destination buffer. */ 00098 *pDst++ = (q15_t) __QSUB16(*pSrcA++, *pSrcB++); 00099 00100 /* Decrement the loop counter */ 00101 blkCnt--; 00102 } 00103 00104 #else 00105 00106 /* Run the below code for Cortex-M0 */ 00107 00108 /* Initialize blkCnt with number of samples */ 00109 blkCnt = blockSize; 00110 00111 while (blkCnt > 0U) 00112 { 00113 /* C = A - B */ 00114 /* Subtract and then store the result in the destination buffer. */ 00115 *pDst++ = (q15_t) __SSAT(((q31_t) * pSrcA++ - *pSrcB++), 16); 00116 00117 /* Decrement the loop counter */ 00118 blkCnt--; 00119 } 00120 00121 #endif /* #if defined (ARM_MATH_DSP) */ 00122 00123 00124 } 00125 00126 /** 00127 * @} end of BasicSub group 00128 */ 00129
Generated on Tue Jul 12 2022 16:47:28 by
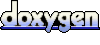