Aded CMSIS5 DSP and NN folder. Needs some work
Embed:
(wiki syntax)
Show/hide line numbers
arm_shift_q15.c
00001 /* ---------------------------------------------------------------------- 00002 * Project: CMSIS DSP Library 00003 * Title: arm_shift_q15.c 00004 * Description: Shifts the elements of a Q15 vector by a specified number of bits 00005 * 00006 * $Date: 27. January 2017 00007 * $Revision: V.1.5.1 00008 * 00009 * Target Processor: Cortex-M cores 00010 * -------------------------------------------------------------------- */ 00011 /* 00012 * Copyright (C) 2010-2017 ARM Limited or its affiliates. All rights reserved. 00013 * 00014 * SPDX-License-Identifier: Apache-2.0 00015 * 00016 * Licensed under the Apache License, Version 2.0 (the License); you may 00017 * not use this file except in compliance with the License. 00018 * You may obtain a copy of the License at 00019 * 00020 * www.apache.org/licenses/LICENSE-2.0 00021 * 00022 * Unless required by applicable law or agreed to in writing, software 00023 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00024 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00025 * See the License for the specific language governing permissions and 00026 * limitations under the License. 00027 */ 00028 00029 #include "arm_math.h" 00030 00031 /** 00032 * @ingroup groupMath 00033 */ 00034 00035 /** 00036 * @addtogroup shift 00037 * @{ 00038 */ 00039 00040 /** 00041 * @brief Shifts the elements of a Q15 vector a specified number of bits. 00042 * @param[in] *pSrc points to the input vector 00043 * @param[in] shiftBits number of bits to shift. A positive value shifts left; a negative value shifts right. 00044 * @param[out] *pDst points to the output vector 00045 * @param[in] blockSize number of samples in the vector 00046 * @return none. 00047 * 00048 * <b>Scaling and Overflow Behavior:</b> 00049 * \par 00050 * The function uses saturating arithmetic. 00051 * Results outside of the allowable Q15 range [0x8000 0x7FFF] will be saturated. 00052 */ 00053 00054 void arm_shift_q15( 00055 q15_t * pSrc, 00056 int8_t shiftBits, 00057 q15_t * pDst, 00058 uint32_t blockSize) 00059 { 00060 uint32_t blkCnt; /* loop counter */ 00061 uint8_t sign; /* Sign of shiftBits */ 00062 00063 #if defined (ARM_MATH_DSP) 00064 00065 /* Run the below code for Cortex-M4 and Cortex-M3 */ 00066 00067 q15_t in1, in2; /* Temporary variables */ 00068 00069 00070 /*loop Unrolling */ 00071 blkCnt = blockSize >> 2U; 00072 00073 /* Getting the sign of shiftBits */ 00074 sign = (shiftBits & 0x80); 00075 00076 /* If the shift value is positive then do right shift else left shift */ 00077 if (sign == 0U) 00078 { 00079 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00080 ** a second loop below computes the remaining 1 to 3 samples. */ 00081 while (blkCnt > 0U) 00082 { 00083 /* Read 2 inputs */ 00084 in1 = *pSrc++; 00085 in2 = *pSrc++; 00086 /* C = A << shiftBits */ 00087 /* Shift the inputs and then store the results in the destination buffer. */ 00088 #ifndef ARM_MATH_BIG_ENDIAN 00089 00090 *__SIMD32(pDst)++ = __PKHBT(__SSAT((in1 << shiftBits), 16), 00091 __SSAT((in2 << shiftBits), 16), 16); 00092 00093 #else 00094 00095 *__SIMD32(pDst)++ = __PKHBT(__SSAT((in2 << shiftBits), 16), 00096 __SSAT((in1 << shiftBits), 16), 16); 00097 00098 #endif /* #ifndef ARM_MATH_BIG_ENDIAN */ 00099 00100 in1 = *pSrc++; 00101 in2 = *pSrc++; 00102 00103 #ifndef ARM_MATH_BIG_ENDIAN 00104 00105 *__SIMD32(pDst)++ = __PKHBT(__SSAT((in1 << shiftBits), 16), 00106 __SSAT((in2 << shiftBits), 16), 16); 00107 00108 #else 00109 00110 *__SIMD32(pDst)++ = __PKHBT(__SSAT((in2 << shiftBits), 16), 00111 __SSAT((in1 << shiftBits), 16), 16); 00112 00113 #endif /* #ifndef ARM_MATH_BIG_ENDIAN */ 00114 00115 /* Decrement the loop counter */ 00116 blkCnt--; 00117 } 00118 00119 /* If the blockSize is not a multiple of 4, compute any remaining output samples here. 00120 ** No loop unrolling is used. */ 00121 blkCnt = blockSize % 0x4U; 00122 00123 while (blkCnt > 0U) 00124 { 00125 /* C = A << shiftBits */ 00126 /* Shift and then store the results in the destination buffer. */ 00127 *pDst++ = __SSAT((*pSrc++ << shiftBits), 16); 00128 00129 /* Decrement the loop counter */ 00130 blkCnt--; 00131 } 00132 } 00133 else 00134 { 00135 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00136 ** a second loop below computes the remaining 1 to 3 samples. */ 00137 while (blkCnt > 0U) 00138 { 00139 /* Read 2 inputs */ 00140 in1 = *pSrc++; 00141 in2 = *pSrc++; 00142 00143 /* C = A >> shiftBits */ 00144 /* Shift the inputs and then store the results in the destination buffer. */ 00145 #ifndef ARM_MATH_BIG_ENDIAN 00146 00147 *__SIMD32(pDst)++ = __PKHBT((in1 >> -shiftBits), 00148 (in2 >> -shiftBits), 16); 00149 00150 #else 00151 00152 *__SIMD32(pDst)++ = __PKHBT((in2 >> -shiftBits), 00153 (in1 >> -shiftBits), 16); 00154 00155 #endif /* #ifndef ARM_MATH_BIG_ENDIAN */ 00156 00157 in1 = *pSrc++; 00158 in2 = *pSrc++; 00159 00160 #ifndef ARM_MATH_BIG_ENDIAN 00161 00162 *__SIMD32(pDst)++ = __PKHBT((in1 >> -shiftBits), 00163 (in2 >> -shiftBits), 16); 00164 00165 #else 00166 00167 *__SIMD32(pDst)++ = __PKHBT((in2 >> -shiftBits), 00168 (in1 >> -shiftBits), 16); 00169 00170 #endif /* #ifndef ARM_MATH_BIG_ENDIAN */ 00171 00172 /* Decrement the loop counter */ 00173 blkCnt--; 00174 } 00175 00176 /* If the blockSize is not a multiple of 4, compute any remaining output samples here. 00177 ** No loop unrolling is used. */ 00178 blkCnt = blockSize % 0x4U; 00179 00180 while (blkCnt > 0U) 00181 { 00182 /* C = A >> shiftBits */ 00183 /* Shift the inputs and then store the results in the destination buffer. */ 00184 *pDst++ = (*pSrc++ >> -shiftBits); 00185 00186 /* Decrement the loop counter */ 00187 blkCnt--; 00188 } 00189 } 00190 00191 #else 00192 00193 /* Run the below code for Cortex-M0 */ 00194 00195 /* Getting the sign of shiftBits */ 00196 sign = (shiftBits & 0x80); 00197 00198 /* If the shift value is positive then do right shift else left shift */ 00199 if (sign == 0U) 00200 { 00201 /* Initialize blkCnt with number of samples */ 00202 blkCnt = blockSize; 00203 00204 while (blkCnt > 0U) 00205 { 00206 /* C = A << shiftBits */ 00207 /* Shift and then store the results in the destination buffer. */ 00208 *pDst++ = __SSAT(((q31_t) * pSrc++ << shiftBits), 16); 00209 00210 /* Decrement the loop counter */ 00211 blkCnt--; 00212 } 00213 } 00214 else 00215 { 00216 /* Initialize blkCnt with number of samples */ 00217 blkCnt = blockSize; 00218 00219 while (blkCnt > 0U) 00220 { 00221 /* C = A >> shiftBits */ 00222 /* Shift the inputs and then store the results in the destination buffer. */ 00223 *pDst++ = (*pSrc++ >> -shiftBits); 00224 00225 /* Decrement the loop counter */ 00226 blkCnt--; 00227 } 00228 } 00229 00230 #endif /* #if defined (ARM_MATH_DSP) */ 00231 00232 } 00233 00234 /** 00235 * @} end of shift group 00236 */ 00237
Generated on Tue Jul 12 2022 16:47:28 by
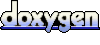