Aded CMSIS5 DSP and NN folder. Needs some work
Embed:
(wiki syntax)
Show/hide line numbers
arm_scale_f32.c
00001 /* ---------------------------------------------------------------------- 00002 * Project: CMSIS DSP Library 00003 * Title: arm_scale_f32.c 00004 * Description: Multiplies a floating-point vector by a scalar 00005 * 00006 * $Date: 27. January 2017 00007 * $Revision: V.1.5.1 00008 * 00009 * Target Processor: Cortex-M cores 00010 * -------------------------------------------------------------------- */ 00011 /* 00012 * Copyright (C) 2010-2017 ARM Limited or its affiliates. All rights reserved. 00013 * 00014 * SPDX-License-Identifier: Apache-2.0 00015 * 00016 * Licensed under the Apache License, Version 2.0 (the License); you may 00017 * not use this file except in compliance with the License. 00018 * You may obtain a copy of the License at 00019 * 00020 * www.apache.org/licenses/LICENSE-2.0 00021 * 00022 * Unless required by applicable law or agreed to in writing, software 00023 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00024 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00025 * See the License for the specific language governing permissions and 00026 * limitations under the License. 00027 */ 00028 00029 #include "arm_math.h" 00030 00031 /** 00032 * @ingroup groupMath 00033 */ 00034 00035 /** 00036 * @defgroup scale Vector Scale 00037 * 00038 * Multiply a vector by a scalar value. For floating-point data, the algorithm used is: 00039 * 00040 * <pre> 00041 * pDst[n] = pSrc[n] * scale, 0 <= n < blockSize. 00042 * </pre> 00043 * 00044 * In the fixed-point Q7, Q15, and Q31 functions, <code>scale</code> is represented by 00045 * a fractional multiplication <code>scaleFract</code> and an arithmetic shift <code>shift</code>. 00046 * The shift allows the gain of the scaling operation to exceed 1.0. 00047 * The algorithm used with fixed-point data is: 00048 * 00049 * <pre> 00050 * pDst[n] = (pSrc[n] * scaleFract) << shift, 0 <= n < blockSize. 00051 * </pre> 00052 * 00053 * The overall scale factor applied to the fixed-point data is 00054 * <pre> 00055 * scale = scaleFract * 2^shift. 00056 * </pre> 00057 * 00058 * The functions support in-place computation allowing the source and destination 00059 * pointers to reference the same memory buffer. 00060 */ 00061 00062 /** 00063 * @addtogroup scale 00064 * @{ 00065 */ 00066 00067 /** 00068 * @brief Multiplies a floating-point vector by a scalar. 00069 * @param[in] *pSrc points to the input vector 00070 * @param[in] scale scale factor to be applied 00071 * @param[out] *pDst points to the output vector 00072 * @param[in] blockSize number of samples in the vector 00073 * @return none. 00074 */ 00075 00076 00077 void arm_scale_f32( 00078 float32_t * pSrc, 00079 float32_t scale, 00080 float32_t * pDst, 00081 uint32_t blockSize) 00082 { 00083 uint32_t blkCnt; /* loop counter */ 00084 #if defined (ARM_MATH_DSP) 00085 00086 /* Run the below code for Cortex-M4 and Cortex-M3 */ 00087 float32_t in1, in2, in3, in4; /* temporary variabels */ 00088 00089 /*loop Unrolling */ 00090 blkCnt = blockSize >> 2U; 00091 00092 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00093 ** a second loop below computes the remaining 1 to 3 samples. */ 00094 while (blkCnt > 0U) 00095 { 00096 /* C = A * scale */ 00097 /* Scale the input and then store the results in the destination buffer. */ 00098 /* read input samples from source */ 00099 in1 = *pSrc; 00100 in2 = *(pSrc + 1); 00101 00102 /* multiply with scaling factor */ 00103 in1 = in1 * scale; 00104 00105 /* read input sample from source */ 00106 in3 = *(pSrc + 2); 00107 00108 /* multiply with scaling factor */ 00109 in2 = in2 * scale; 00110 00111 /* read input sample from source */ 00112 in4 = *(pSrc + 3); 00113 00114 /* multiply with scaling factor */ 00115 in3 = in3 * scale; 00116 in4 = in4 * scale; 00117 /* store the result to destination */ 00118 *pDst = in1; 00119 *(pDst + 1) = in2; 00120 *(pDst + 2) = in3; 00121 *(pDst + 3) = in4; 00122 00123 /* update pointers to process next samples */ 00124 pSrc += 4U; 00125 pDst += 4U; 00126 00127 /* Decrement the loop counter */ 00128 blkCnt--; 00129 } 00130 00131 /* If the blockSize is not a multiple of 4, compute any remaining output samples here. 00132 ** No loop unrolling is used. */ 00133 blkCnt = blockSize % 0x4U; 00134 00135 #else 00136 00137 /* Run the below code for Cortex-M0 */ 00138 00139 /* Initialize blkCnt with number of samples */ 00140 blkCnt = blockSize; 00141 00142 #endif /* #if defined (ARM_MATH_DSP) */ 00143 00144 while (blkCnt > 0U) 00145 { 00146 /* C = A * scale */ 00147 /* Scale the input and then store the result in the destination buffer. */ 00148 *pDst++ = (*pSrc++) * scale; 00149 00150 /* Decrement the loop counter */ 00151 blkCnt--; 00152 } 00153 } 00154 00155 /** 00156 * @} end of scale group 00157 */ 00158
Generated on Tue Jul 12 2022 16:47:28 by
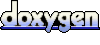