Aded CMSIS5 DSP and NN folder. Needs some work
Embed:
(wiki syntax)
Show/hide line numbers
arm_relu_q7.c
00001 /* 00002 * Copyright (C) 2010-2018 Arm Limited or its affiliates. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: Apache-2.0 00005 * 00006 * Licensed under the Apache License, Version 2.0 (the License); you may 00007 * not use this file except in compliance with the License. 00008 * You may obtain a copy of the License at 00009 * 00010 * www.apache.org/licenses/LICENSE-2.0 00011 * 00012 * Unless required by applicable law or agreed to in writing, software 00013 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00014 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 * See the License for the specific language governing permissions and 00016 * limitations under the License. 00017 */ 00018 00019 /* ---------------------------------------------------------------------- 00020 * Project: CMSIS NN Library 00021 * Title: arm_relu_q7.c 00022 * Description: Q7 version of ReLU 00023 * 00024 * $Date: 17. January 2018 00025 * $Revision: V.1.0.0 00026 * 00027 * Target Processor: Cortex-M cores 00028 * 00029 * -------------------------------------------------------------------- */ 00030 00031 #include "arm_math.h" 00032 #include "arm_nnfunctions.h" 00033 00034 /** 00035 * @ingroup groupNN 00036 */ 00037 00038 /** 00039 * @addtogroup Acti 00040 * @{ 00041 */ 00042 00043 /** 00044 * @brief Q7 RELU function 00045 * @param[in,out] data pointer to input 00046 * @param[in] size number of elements 00047 * @return none. 00048 * 00049 * @details 00050 * 00051 * Optimized relu with QSUB instructions. 00052 * 00053 */ 00054 00055 void arm_relu_q7(q7_t * data, uint16_t size) 00056 { 00057 00058 #if defined (ARM_MATH_DSP) 00059 /* Run the following code for Cortex-M4 and Cortex-M7 */ 00060 00061 uint16_t i = size >> 2; 00062 q7_t *pIn = data; 00063 q7_t *pOut = data; 00064 q31_t in; 00065 q31_t buf; 00066 q31_t mask; 00067 00068 while (i) 00069 { 00070 in = *__SIMD32(pIn)++; 00071 00072 /* extract the first bit */ 00073 buf = __ROR(in & 0x80808080, 7); 00074 00075 /* if MSB=1, mask will be 0xFF, 0x0 otherwise */ 00076 mask = __QSUB8(0x00000000, buf); 00077 00078 *__SIMD32(pOut)++ = in & (~mask); 00079 i--; 00080 } 00081 00082 i = size & 0x3; 00083 while (i) 00084 { 00085 if (*pIn < 0) 00086 { 00087 *pIn = 0; 00088 } 00089 pIn++; 00090 i--; 00091 } 00092 00093 #else 00094 /* Run the following code as reference implementation for Cortex-M0 and Cortex-M3 */ 00095 00096 uint16_t i; 00097 00098 for (i = 0; i < size; i++) 00099 { 00100 if (data[i] < 0) 00101 data[i] = 0; 00102 } 00103 00104 #endif /* ARM_MATH_DSP */ 00105 00106 } 00107 00108 /** 00109 * @} end of Acti group 00110 */ 00111
Generated on Tue Jul 12 2022 16:47:28 by
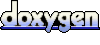