Aded CMSIS5 DSP and NN folder. Needs some work
Embed:
(wiki syntax)
Show/hide line numbers
arm_q7_to_q15.c
00001 /* ---------------------------------------------------------------------- 00002 * Project: CMSIS DSP Library 00003 * Title: arm_q7_to_q15.c 00004 * Description: Converts the elements of the Q7 vector to Q15 vector 00005 * 00006 * $Date: 27. January 2017 00007 * $Revision: V.1.5.1 00008 * 00009 * Target Processor: Cortex-M cores 00010 * -------------------------------------------------------------------- */ 00011 /* 00012 * Copyright (C) 2010-2017 ARM Limited or its affiliates. All rights reserved. 00013 * 00014 * SPDX-License-Identifier: Apache-2.0 00015 * 00016 * Licensed under the Apache License, Version 2.0 (the License); you may 00017 * not use this file except in compliance with the License. 00018 * You may obtain a copy of the License at 00019 * 00020 * www.apache.org/licenses/LICENSE-2.0 00021 * 00022 * Unless required by applicable law or agreed to in writing, software 00023 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00024 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00025 * See the License for the specific language governing permissions and 00026 * limitations under the License. 00027 */ 00028 00029 #include "arm_math.h" 00030 00031 /** 00032 * @ingroup groupSupport 00033 */ 00034 00035 /** 00036 * @addtogroup q7_to_x 00037 * @{ 00038 */ 00039 00040 00041 00042 00043 /** 00044 * @brief Converts the elements of the Q7 vector to Q15 vector. 00045 * @param[in] *pSrc points to the Q7 input vector 00046 * @param[out] *pDst points to the Q15 output vector 00047 * @param[in] blockSize length of the input vector 00048 * @return none. 00049 * 00050 * \par Description: 00051 * 00052 * The equation used for the conversion process is: 00053 * 00054 * <pre> 00055 * pDst[n] = (q15_t) pSrc[n] << 8; 0 <= n < blockSize. 00056 * </pre> 00057 * 00058 */ 00059 00060 00061 void arm_q7_to_q15( 00062 q7_t * pSrc, 00063 q15_t * pDst, 00064 uint32_t blockSize) 00065 { 00066 q7_t *pIn = pSrc; /* Src pointer */ 00067 uint32_t blkCnt; /* loop counter */ 00068 00069 #if defined (ARM_MATH_DSP) 00070 q31_t in; 00071 q31_t in1, in2; 00072 q31_t out1, out2; 00073 00074 /* Run the below code for Cortex-M4 and Cortex-M3 */ 00075 00076 /*loop Unrolling */ 00077 blkCnt = blockSize >> 2U; 00078 00079 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00080 ** a second loop below computes the remaining 1 to 3 samples. */ 00081 while (blkCnt > 0U) 00082 { 00083 /* C = (q15_t) A << 8 */ 00084 /* convert from q7 to q15 and then store the results in the destination buffer */ 00085 in = *__SIMD32(pIn)++; 00086 00087 /* rotatate in by 8 and extend two q7_t values to q15_t values */ 00088 in1 = __SXTB16(__ROR(in, 8)); 00089 00090 /* extend remainig two q7_t values to q15_t values */ 00091 in2 = __SXTB16(in); 00092 00093 in1 = in1 << 8U; 00094 in2 = in2 << 8U; 00095 00096 in1 = in1 & 0xFF00FF00; 00097 in2 = in2 & 0xFF00FF00; 00098 00099 #ifndef ARM_MATH_BIG_ENDIAN 00100 00101 out2 = __PKHTB(in1, in2, 16); 00102 out1 = __PKHBT(in2, in1, 16); 00103 00104 #else 00105 00106 out1 = __PKHTB(in1, in2, 16); 00107 out2 = __PKHBT(in2, in1, 16); 00108 00109 #endif 00110 00111 *__SIMD32(pDst)++ = out1; 00112 *__SIMD32(pDst)++ = out2; 00113 00114 /* Decrement the loop counter */ 00115 blkCnt--; 00116 } 00117 00118 /* If the blockSize is not a multiple of 4, compute any remaining output samples here. 00119 ** No loop unrolling is used. */ 00120 blkCnt = blockSize % 0x4U; 00121 00122 #else 00123 00124 /* Run the below code for Cortex-M0 */ 00125 00126 /* Loop over blockSize number of values */ 00127 blkCnt = blockSize; 00128 00129 #endif /* #if defined (ARM_MATH_DSP) */ 00130 00131 while (blkCnt > 0U) 00132 { 00133 /* C = (q15_t) A << 8 */ 00134 /* convert from q7 to q15 and then store the results in the destination buffer */ 00135 *pDst++ = (q15_t) * pIn++ << 8; 00136 00137 /* Decrement the loop counter */ 00138 blkCnt--; 00139 } 00140 00141 } 00142 00143 /** 00144 * @} end of q7_to_x group 00145 */ 00146
Generated on Tue Jul 12 2022 16:47:27 by
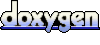