Aded CMSIS5 DSP and NN folder. Needs some work
Embed:
(wiki syntax)
Show/hide line numbers
arm_nn_activations_q15.c
00001 /* 00002 * Copyright (C) 2010-2018 Arm Limited or its affiliates. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: Apache-2.0 00005 * 00006 * Licensed under the Apache License, Version 2.0 (the License); you may 00007 * not use this file except in compliance with the License. 00008 * You may obtain a copy of the License at 00009 * 00010 * www.apache.org/licenses/LICENSE-2.0 00011 * 00012 * Unless required by applicable law or agreed to in writing, software 00013 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00014 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 * See the License for the specific language governing permissions and 00016 * limitations under the License. 00017 */ 00018 00019 /* ---------------------------------------------------------------------- 00020 * Project: CMSIS NN Library 00021 * Title: arm_nn_activations_q15.c 00022 * Description: Q15 neural network activation function using direct table look-up 00023 * 00024 * $Date: 17. January 2018 00025 * $Revision: V.1.0.0 00026 * 00027 * Target Processor: Cortex-M cores 00028 * 00029 * -------------------------------------------------------------------- */ 00030 00031 #include "arm_math.h" 00032 #include "arm_common_tables.h" 00033 #include "arm_nnfunctions.h" 00034 00035 /** 00036 * @ingroup groupNN 00037 */ 00038 00039 /** 00040 * @addtogroup Acti 00041 * @{ 00042 */ 00043 00044 /** 00045 * @brief Q15 neural network activation function using direct table look-up 00046 * @param[in,out] data pointer to input 00047 * @param[in] size number of elements 00048 * @param[in] int_width bit-width of the integer part, assume to be smaller than 3 00049 * @param[in] type type of activation functions 00050 * @return none. 00051 * 00052 * @details 00053 * 00054 * This is the direct table look-up approach. 00055 * 00056 * Assume here the integer part of the fixed-point is <= 3. 00057 * More than 3 just not making much sense, makes no difference with 00058 * saturation followed by any of these activation functions. 00059 */ 00060 00061 void arm_nn_activations_direct_q15(q15_t * data, uint16_t size, uint16_t int_width, arm_nn_activation_type type) 00062 { 00063 uint16_t i = size; 00064 q15_t *pIn = data; 00065 q15_t *pOut = data; 00066 uint16_t shift_size = 8 + 3 - int_width; 00067 uint32_t bit_mask = 0x7FF >> int_width; 00068 uint32_t full_frac = bit_mask + 1; 00069 const q15_t *lookup_table; 00070 00071 switch (type) 00072 { 00073 case ARM_SIGMOID: 00074 lookup_table = sigmoidTable_q15; 00075 break; 00076 case ARM_TANH: 00077 default: 00078 lookup_table = tanhTable_q15; 00079 break; 00080 } 00081 00082 while (i) 00083 { 00084 q15_t out; 00085 q15_t in = *pIn++; 00086 q15_t frac = (uint32_t) in & bit_mask; 00087 q15_t value = lookup_table[__USAT(in >> shift_size, 8)]; 00088 q15_t value2 = lookup_table[__USAT(1 + (in >> shift_size), 8)]; 00089 00090 /* doing the interpolation here for better accuracy */ 00091 out = ((q31_t) (full_frac - frac) * value + (q31_t) value2 * frac) >> shift_size; 00092 00093 *pOut++ = out; 00094 i--; 00095 } 00096 00097 } 00098 00099 /** 00100 * @} end of Acti group 00101 */ 00102
Generated on Tue Jul 12 2022 16:47:27 by
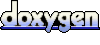