Aded CMSIS5 DSP and NN folder. Needs some work
Embed:
(wiki syntax)
Show/hide line numbers
arm_max_f32.c
00001 /* ---------------------------------------------------------------------- 00002 * Project: CMSIS DSP Library 00003 * Title: arm_max_f32.c 00004 * Description: Maximum value of a floating-point vector 00005 * 00006 * $Date: 27. January 2017 00007 * $Revision: V.1.5.1 00008 * 00009 * Target Processor: Cortex-M cores 00010 * -------------------------------------------------------------------- */ 00011 /* 00012 * Copyright (C) 2010-2017 ARM Limited or its affiliates. All rights reserved. 00013 * 00014 * SPDX-License-Identifier: Apache-2.0 00015 * 00016 * Licensed under the Apache License, Version 2.0 (the License); you may 00017 * not use this file except in compliance with the License. 00018 * You may obtain a copy of the License at 00019 * 00020 * www.apache.org/licenses/LICENSE-2.0 00021 * 00022 * Unless required by applicable law or agreed to in writing, software 00023 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00024 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00025 * See the License for the specific language governing permissions and 00026 * limitations under the License. 00027 */ 00028 00029 #include "arm_math.h" 00030 00031 /** 00032 * @ingroup groupStats 00033 */ 00034 00035 /** 00036 * @defgroup Max Maximum 00037 * 00038 * Computes the maximum value of an array of data. 00039 * The function returns both the maximum value and its position within the array. 00040 * There are separate functions for floating-point, Q31, Q15, and Q7 data types. 00041 */ 00042 00043 /** 00044 * @addtogroup Max 00045 * @{ 00046 */ 00047 00048 00049 /** 00050 * @brief Maximum value of a floating-point vector. 00051 * @param[in] *pSrc points to the input vector 00052 * @param[in] blockSize length of the input vector 00053 * @param[out] *pResult maximum value returned here 00054 * @param[out] *pIndex index of maximum value returned here 00055 * @return none. 00056 */ 00057 00058 void arm_max_f32( 00059 float32_t * pSrc, 00060 uint32_t blockSize, 00061 float32_t * pResult, 00062 uint32_t * pIndex) 00063 { 00064 #if defined (ARM_MATH_DSP) 00065 /* Run the below code for Cortex-M4 and Cortex-M3 */ 00066 00067 float32_t maxVal1, maxVal2, out; /* Temporary variables to store the output value. */ 00068 uint32_t blkCnt, outIndex, count; /* loop counter */ 00069 00070 /* Initialise the count value. */ 00071 count = 0U; 00072 /* Initialise the index value to zero. */ 00073 outIndex = 0U; 00074 /* Load first input value that act as reference value for comparision */ 00075 out = *pSrc++; 00076 00077 /* Loop unrolling */ 00078 blkCnt = (blockSize - 1U) >> 2U; 00079 00080 while (blkCnt > 0U) 00081 { 00082 /* Initialize maxVal to the next consecutive values one by one */ 00083 maxVal1 = *pSrc++; 00084 maxVal2 = *pSrc++; 00085 00086 /* compare for the maximum value */ 00087 if (out < maxVal1) 00088 { 00089 /* Update the maximum value and its index */ 00090 out = maxVal1; 00091 outIndex = count + 1U; 00092 } 00093 00094 /* compare for the maximum value */ 00095 if (out < maxVal2) 00096 { 00097 /* Update the maximum value and its index */ 00098 out = maxVal2; 00099 outIndex = count + 2U; 00100 } 00101 00102 /* Initialize maxVal to the next consecutive values one by one */ 00103 maxVal1 = *pSrc++; 00104 maxVal2 = *pSrc++; 00105 00106 /* compare for the maximum value */ 00107 if (out < maxVal1) 00108 { 00109 /* Update the maximum value and its index */ 00110 out = maxVal1; 00111 outIndex = count + 3U; 00112 } 00113 00114 /* compare for the maximum value */ 00115 if (out < maxVal2) 00116 { 00117 /* Update the maximum value and its index */ 00118 out = maxVal2; 00119 outIndex = count + 4U; 00120 } 00121 00122 count += 4U; 00123 00124 /* Decrement the loop counter */ 00125 blkCnt--; 00126 } 00127 00128 /* if (blockSize - 1U) is not multiple of 4 */ 00129 blkCnt = (blockSize - 1U) % 4U; 00130 00131 #else 00132 /* Run the below code for Cortex-M0 */ 00133 00134 float32_t maxVal1, out; /* Temporary variables to store the output value. */ 00135 uint32_t blkCnt, outIndex; /* loop counter */ 00136 00137 /* Initialise the index value to zero. */ 00138 outIndex = 0U; 00139 /* Load first input value that act as reference value for comparision */ 00140 out = *pSrc++; 00141 00142 blkCnt = (blockSize - 1U); 00143 00144 #endif /* #if defined (ARM_MATH_DSP) */ 00145 00146 while (blkCnt > 0U) 00147 { 00148 /* Initialize maxVal to the next consecutive values one by one */ 00149 maxVal1 = *pSrc++; 00150 00151 /* compare for the maximum value */ 00152 if (out < maxVal1) 00153 { 00154 /* Update the maximum value and it's index */ 00155 out = maxVal1; 00156 outIndex = blockSize - blkCnt; 00157 } 00158 00159 /* Decrement the loop counter */ 00160 blkCnt--; 00161 } 00162 00163 /* Store the maximum value and it's index into destination pointers */ 00164 *pResult = out; 00165 *pIndex = outIndex; 00166 } 00167 00168 /** 00169 * @} end of Max group 00170 */ 00171
Generated on Tue Jul 12 2022 16:47:27 by
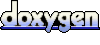