Aded CMSIS5 DSP and NN folder. Needs some work
Embed:
(wiki syntax)
Show/hide line numbers
arm_mat_sub_q15.c
00001 /* ---------------------------------------------------------------------- 00002 * Project: CMSIS DSP Library 00003 * Title: arm_mat_sub_q15.c 00004 * Description: Q15 Matrix subtraction 00005 * 00006 * $Date: 27. January 2017 00007 * $Revision: V.1.5.1 00008 * 00009 * Target Processor: Cortex-M cores 00010 * -------------------------------------------------------------------- */ 00011 /* 00012 * Copyright (C) 2010-2017 ARM Limited or its affiliates. All rights reserved. 00013 * 00014 * SPDX-License-Identifier: Apache-2.0 00015 * 00016 * Licensed under the Apache License, Version 2.0 (the License); you may 00017 * not use this file except in compliance with the License. 00018 * You may obtain a copy of the License at 00019 * 00020 * www.apache.org/licenses/LICENSE-2.0 00021 * 00022 * Unless required by applicable law or agreed to in writing, software 00023 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00024 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00025 * See the License for the specific language governing permissions and 00026 * limitations under the License. 00027 */ 00028 00029 #include "arm_math.h" 00030 00031 /** 00032 * @ingroup groupMatrix 00033 */ 00034 00035 /** 00036 * @addtogroup MatrixSub 00037 * @{ 00038 */ 00039 00040 /** 00041 * @brief Q15 matrix subtraction. 00042 * @param[in] *pSrcA points to the first input matrix structure 00043 * @param[in] *pSrcB points to the second input matrix structure 00044 * @param[out] *pDst points to output matrix structure 00045 * @return The function returns either 00046 * <code>ARM_MATH_SIZE_MISMATCH</code> or <code>ARM_MATH_SUCCESS</code> based on the outcome of size checking. 00047 * 00048 * <b>Scaling and Overflow Behavior:</b> 00049 * \par 00050 * The function uses saturating arithmetic. 00051 * Results outside of the allowable Q15 range [0x8000 0x7FFF] will be saturated. 00052 */ 00053 00054 arm_status arm_mat_sub_q15( 00055 const arm_matrix_instance_q15 * pSrcA, 00056 const arm_matrix_instance_q15 * pSrcB, 00057 arm_matrix_instance_q15 * pDst) 00058 { 00059 q15_t *pInA = pSrcA->pData; /* input data matrix pointer A */ 00060 q15_t *pInB = pSrcB->pData; /* input data matrix pointer B */ 00061 q15_t *pOut = pDst->pData; /* output data matrix pointer */ 00062 uint32_t numSamples; /* total number of elements in the matrix */ 00063 uint32_t blkCnt; /* loop counters */ 00064 arm_status status; /* status of matrix subtraction */ 00065 00066 00067 #ifdef ARM_MATH_MATRIX_CHECK 00068 00069 00070 /* Check for matrix mismatch condition */ 00071 if ((pSrcA->numRows != pSrcB->numRows) || 00072 (pSrcA->numCols != pSrcB->numCols) || 00073 (pSrcA->numRows != pDst->numRows) || (pSrcA->numCols != pDst->numCols)) 00074 { 00075 /* Set status as ARM_MATH_SIZE_MISMATCH */ 00076 status = ARM_MATH_SIZE_MISMATCH; 00077 } 00078 else 00079 #endif /* #ifdef ARM_MATH_MATRIX_CHECK */ 00080 00081 { 00082 /* Total number of samples in the input matrix */ 00083 numSamples = (uint32_t) pSrcA->numRows * pSrcA->numCols; 00084 00085 #if defined (ARM_MATH_DSP) 00086 00087 /* Run the below code for Cortex-M4 and Cortex-M3 */ 00088 00089 /* Apply loop unrolling */ 00090 blkCnt = numSamples >> 2U; 00091 00092 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00093 ** a second loop below computes the remaining 1 to 3 samples. */ 00094 while (blkCnt > 0U) 00095 { 00096 /* C(m,n) = A(m,n) - B(m,n) */ 00097 /* Subtract, Saturate and then store the results in the destination buffer. */ 00098 *__SIMD32(pOut)++ = __QSUB16(*__SIMD32(pInA)++, *__SIMD32(pInB)++); 00099 *__SIMD32(pOut)++ = __QSUB16(*__SIMD32(pInA)++, *__SIMD32(pInB)++); 00100 00101 /* Decrement the loop counter */ 00102 blkCnt--; 00103 } 00104 00105 /* If the blockSize is not a multiple of 4, compute any remaining output samples here. 00106 ** No loop unrolling is used. */ 00107 blkCnt = numSamples % 0x4U; 00108 00109 while (blkCnt > 0U) 00110 { 00111 /* C(m,n) = A(m,n) - B(m,n) */ 00112 /* Subtract and then store the results in the destination buffer. */ 00113 *pOut++ = (q15_t) __QSUB16(*pInA++, *pInB++); 00114 00115 /* Decrement the loop counter */ 00116 blkCnt--; 00117 } 00118 00119 #else 00120 00121 /* Run the below code for Cortex-M0 */ 00122 00123 /* Initialize blkCnt with number of samples */ 00124 blkCnt = numSamples; 00125 00126 while (blkCnt > 0U) 00127 { 00128 /* C(m,n) = A(m,n) - B(m,n) */ 00129 /* Subtract and then store the results in the destination buffer. */ 00130 *pOut++ = (q15_t) __SSAT(((q31_t) * pInA++ - *pInB++), 16); 00131 00132 /* Decrement the loop counter */ 00133 blkCnt--; 00134 } 00135 00136 #endif /* #if defined (ARM_MATH_DSP) */ 00137 00138 /* Set status as ARM_MATH_SUCCESS */ 00139 status = ARM_MATH_SUCCESS; 00140 } 00141 00142 /* Return to application */ 00143 return (status); 00144 } 00145 00146 /** 00147 * @} end of MatrixSub group 00148 */ 00149
Generated on Tue Jul 12 2022 16:47:27 by
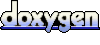