Aded CMSIS5 DSP and NN folder. Needs some work
Embed:
(wiki syntax)
Show/hide line numbers
arm_cos_q31.c
00001 /* ---------------------------------------------------------------------- 00002 * Project: CMSIS DSP Library 00003 * Title: arm_cos_q31.c 00004 * Description: Fast cosine calculation for Q31 values 00005 * 00006 * $Date: 27. January 2017 00007 * $Revision: V.1.5.1 00008 * 00009 * Target Processor: Cortex-M cores 00010 * -------------------------------------------------------------------- */ 00011 /* 00012 * Copyright (C) 2010-2017 ARM Limited or its affiliates. All rights reserved. 00013 * 00014 * SPDX-License-Identifier: Apache-2.0 00015 * 00016 * Licensed under the Apache License, Version 2.0 (the License); you may 00017 * not use this file except in compliance with the License. 00018 * You may obtain a copy of the License at 00019 * 00020 * www.apache.org/licenses/LICENSE-2.0 00021 * 00022 * Unless required by applicable law or agreed to in writing, software 00023 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00024 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00025 * See the License for the specific language governing permissions and 00026 * limitations under the License. 00027 */ 00028 00029 #include "arm_math.h" 00030 #include "arm_common_tables.h" 00031 00032 /** 00033 * @ingroup groupFastMath 00034 */ 00035 00036 /** 00037 * @addtogroup cos 00038 * @{ 00039 */ 00040 00041 /** 00042 * @brief Fast approximation to the trigonometric cosine function for Q31 data. 00043 * @param[in] x Scaled input value in radians. 00044 * @return cos(x). 00045 * 00046 * The Q31 input value is in the range [0 +0.9999] and is mapped to a radian 00047 * value in the range [0 2*pi). 00048 */ 00049 00050 q31_t arm_cos_q31( 00051 q31_t x) 00052 { 00053 q31_t cosVal; /* Temporary variables for input, output */ 00054 int32_t index; /* Index variables */ 00055 q31_t a, b; /* Four nearest output values */ 00056 q31_t fract; /* Temporary values for fractional values */ 00057 00058 /* add 0.25 (pi/2) to read sine table */ 00059 x = (uint32_t)x + 0x20000000; 00060 if (x < 0) 00061 { /* convert negative numbers to corresponding positive ones */ 00062 x = (uint32_t)x + 0x80000000; 00063 } 00064 00065 /* Calculate the nearest index */ 00066 index = (uint32_t)x >> FAST_MATH_Q31_SHIFT; 00067 00068 /* Calculation of fractional value */ 00069 fract = (x - (index << FAST_MATH_Q31_SHIFT)) << 9; 00070 00071 /* Read two nearest values of input value from the sin table */ 00072 a = sinTable_q31[index]; 00073 b = sinTable_q31[index+1]; 00074 00075 /* Linear interpolation process */ 00076 cosVal = (q63_t)(0x80000000-fract)*a >> 32; 00077 cosVal = (q31_t)((((q63_t)cosVal << 32) + ((q63_t)fract*b)) >> 32); 00078 00079 return cosVal << 1; 00080 } 00081 00082 /** 00083 * @} end of cos group 00084 */ 00085
Generated on Tue Jul 12 2022 16:46:23 by
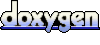