Aded CMSIS5 DSP and NN folder. Needs some work
Embed:
(wiki syntax)
Show/hide line numbers
arm_cos_f32.c
00001 /* ---------------------------------------------------------------------- 00002 * Project: CMSIS DSP Library 00003 * Title: arm_cos_f32.c 00004 * Description: Fast cosine calculation for floating-point values 00005 * 00006 * $Date: 27. January 2017 00007 * $Revision: V.1.5.1 00008 * 00009 * Target Processor: Cortex-M cores 00010 * -------------------------------------------------------------------- */ 00011 /* 00012 * Copyright (C) 2010-2017 ARM Limited or its affiliates. All rights reserved. 00013 * 00014 * SPDX-License-Identifier: Apache-2.0 00015 * 00016 * Licensed under the Apache License, Version 2.0 (the License); you may 00017 * not use this file except in compliance with the License. 00018 * You may obtain a copy of the License at 00019 * 00020 * www.apache.org/licenses/LICENSE-2.0 00021 * 00022 * Unless required by applicable law or agreed to in writing, software 00023 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00024 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00025 * See the License for the specific language governing permissions and 00026 * limitations under the License. 00027 */ 00028 00029 #include "arm_math.h" 00030 #include "arm_common_tables.h" 00031 /** 00032 * @ingroup groupFastMath 00033 */ 00034 00035 /** 00036 * @defgroup cos Cosine 00037 * 00038 * Computes the trigonometric cosine function using a combination of table lookup 00039 * and linear interpolation. There are separate functions for 00040 * Q15, Q31, and floating-point data types. 00041 * The input to the floating-point version is in radians and in the range [0 2*pi) while the 00042 * fixed-point Q15 and Q31 have a scaled input with the range 00043 * [0 +0.9999] mapping to [0 2*pi). The fixed-point range is chosen so that a 00044 * value of 2*pi wraps around to 0. 00045 * 00046 * The implementation is based on table lookup using 256 values together with linear interpolation. 00047 * The steps used are: 00048 * -# Calculation of the nearest integer table index 00049 * -# Compute the fractional portion (fract) of the table index. 00050 * -# The final result equals <code>(1.0f-fract)*a + fract*b;</code> 00051 * 00052 * where 00053 * <pre> 00054 * b=Table[index+0]; 00055 * c=Table[index+1]; 00056 * </pre> 00057 */ 00058 00059 /** 00060 * @addtogroup cos 00061 * @{ 00062 */ 00063 00064 /** 00065 * @brief Fast approximation to the trigonometric cosine function for floating-point data. 00066 * @param[in] x input value in radians. 00067 * @return cos(x). 00068 */ 00069 00070 float32_t arm_cos_f32( 00071 float32_t x) 00072 { 00073 float32_t cosVal, fract, in; /* Temporary variables for input, output */ 00074 uint16_t index; /* Index variable */ 00075 float32_t a, b; /* Two nearest output values */ 00076 int32_t n; 00077 float32_t findex; 00078 00079 /* input x is in radians */ 00080 /* Scale the input to [0 1] range from [0 2*PI] , divide input by 2*pi, add 0.25 (pi/2) to read sine table */ 00081 in = x * 0.159154943092f + 0.25f; 00082 00083 /* Calculation of floor value of input */ 00084 n = (int32_t) in; 00085 00086 /* Make negative values towards -infinity */ 00087 if (in < 0.0f) 00088 { 00089 n--; 00090 } 00091 00092 /* Map input value to [0 1] */ 00093 in = in - (float32_t) n; 00094 00095 /* Calculation of index of the table */ 00096 findex = (float32_t) FAST_MATH_TABLE_SIZE * in; 00097 index = ((uint16_t)findex) & 0x1ff; 00098 00099 /* fractional value calculation */ 00100 fract = findex - (float32_t) index; 00101 00102 /* Read two nearest values of input value from the cos table */ 00103 a = sinTable_f32[index]; 00104 b = sinTable_f32[index+1]; 00105 00106 /* Linear interpolation process */ 00107 cosVal = (1.0f-fract)*a + fract*b; 00108 00109 /* Return the output value */ 00110 return (cosVal); 00111 } 00112 00113 /** 00114 * @} end of cos group 00115 */ 00116
Generated on Tue Jul 12 2022 16:46:23 by
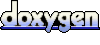