Aded CMSIS5 DSP and NN folder. Needs some work
Embed:
(wiki syntax)
Show/hide line numbers
arm_convolve_HWC_q15_fast.c
00001 /* 00002 * Copyright (C) 2010-2018 Arm Limited or its affiliates. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: Apache-2.0 00005 * 00006 * Licensed under the Apache License, Version 2.0 (the License); you may 00007 * not use this file except in compliance with the License. 00008 * You may obtain a copy of the License at 00009 * 00010 * www.apache.org/licenses/LICENSE-2.0 00011 * 00012 * Unless required by applicable law or agreed to in writing, software 00013 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00014 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 * See the License for the specific language governing permissions and 00016 * limitations under the License. 00017 */ 00018 00019 /* ---------------------------------------------------------------------- 00020 * Project: CMSIS NN Library 00021 * Title: arm_convolve_HWC_q15_fast.c 00022 * Description: Fast Q15 version of convolution 00023 * 00024 * $Date: 17. January 2018 00025 * $Revision: V.1.0.0 00026 * 00027 * Target Processor: Cortex-M cores 00028 * 00029 * -------------------------------------------------------------------- */ 00030 00031 #include "arm_math.h" 00032 #include "arm_nnfunctions.h" 00033 00034 /** 00035 * @ingroup groupNN 00036 */ 00037 00038 /** 00039 * @addtogroup NNConv 00040 * @{ 00041 */ 00042 00043 /** 00044 * @brief Fast Q15 convolution function 00045 * @param[in] Im_in pointer to input tensor 00046 * @param[in] dim_im_in input tensor dimention 00047 * @param[in] ch_im_in number of input tensor channels 00048 * @param[in] wt pointer to kernel weights 00049 * @param[in] ch_im_out number of filters, i.e., output tensor channels 00050 * @param[in] dim_kernel filter kernel size 00051 * @param[in] padding padding sizes 00052 * @param[in] stride convolution stride 00053 * @param[in] bias pointer to bias 00054 * @param[in] bias_shift amount of left-shift for bias 00055 * @param[in] out_shift amount of right-shift for output 00056 * @param[in,out] Im_out pointer to output tensor 00057 * @param[in] dim_im_out output tensor dimension 00058 * @param[in,out] bufferA pointer to buffer space for input 00059 * @param[in,out] bufferB pointer to buffer space for output 00060 * @return The function returns either 00061 * <code>ARM_MATH_SIZE_MISMATCH</code> or <code>ARM_MATH_SUCCESS</code> based on the outcome of size checking. 00062 * 00063 * @details 00064 * 00065 * <b>Buffer size:</b> 00066 * 00067 * bufferA size: 2*ch_im_in*dim_kernel*dim_kernel 00068 * 00069 * bufferB size: 0 00070 * 00071 * <b>Input dimension constraints:</b> 00072 * 00073 * ch_im_in is multiple of 2 00074 * 00075 * ch_im_out is multipe of 2 00076 * 00077 */ 00078 00079 arm_status 00080 arm_convolve_HWC_q15_fast(const q15_t * Im_in, 00081 const uint16_t dim_im_in, 00082 const uint16_t ch_im_in, 00083 const q15_t * wt, 00084 const uint16_t ch_im_out, 00085 const uint16_t dim_kernel, 00086 const uint16_t padding, 00087 const uint16_t stride, 00088 const q15_t * bias, 00089 const uint16_t bias_shift, 00090 const uint16_t out_shift, 00091 q15_t * Im_out, 00092 const uint16_t dim_im_out, 00093 q15_t * bufferA, 00094 q7_t * bufferB) 00095 { 00096 00097 #if defined (ARM_MATH_DSP) 00098 int16_t i_out_y, i_out_x, i_ker_y, i_ker_x; 00099 00100 q15_t *pBuffer = bufferA; 00101 q15_t *im_buffer = bufferA; 00102 q15_t *pOut = Im_out; 00103 00104 if (ch_im_in % 2 != 0 || ch_im_out % 2 != 0) 00105 { 00106 /* check if the input dimension meets the constraints */ 00107 return ARM_MATH_SIZE_MISMATCH; 00108 } 00109 00110 /* Run the following code for Cortex-M4 and Cortex-M7 */ 00111 00112 /* This part implements the im2col function */ 00113 for (i_out_y = 0; i_out_y < dim_im_out; i_out_y++) 00114 { 00115 for (i_out_x = 0; i_out_x < dim_im_out; i_out_x++) 00116 { 00117 for (i_ker_y = i_out_y * stride - padding; i_ker_y < i_out_y * stride - padding + dim_kernel; i_ker_y++) 00118 { 00119 for (i_ker_x = i_out_x * stride - padding; i_ker_x < i_out_x * stride - padding + dim_kernel; i_ker_x++) 00120 { 00121 if (i_ker_y < 0 || i_ker_y >= dim_im_in || i_ker_x < 0 || i_ker_x >= dim_im_in) 00122 { 00123 /* arm_fill_q15(0, pBuffer, ch_im_in); */ 00124 memset(pBuffer, 0, sizeof(q15_t)*ch_im_in); 00125 } else 00126 { 00127 /* arm_copy_q15((q15_t *) Im_in + (i_ker_y * dim_im_in + i_ker_x) * ch_im_in, pBuffer, ch_im_in); */ 00128 memcpy(pBuffer, (q15_t *) Im_in + (i_ker_y * dim_im_in + i_ker_x) * ch_im_in, sizeof(q15_t)*ch_im_in); 00129 } 00130 pBuffer += ch_im_in; 00131 } 00132 } 00133 00134 if (i_out_x & 0x1) 00135 { 00136 int i; 00137 /* initialize the matrix pointers for A */ 00138 const q15_t *pA = wt; 00139 00140 /* set up the second output pointers */ 00141 q15_t *pOut2 = pOut + ch_im_out; 00142 00143 /* this loop over rows in A */ 00144 for (i = 0; i < ch_im_out; i += 2) 00145 { 00146 /* setup pointers for B */ 00147 q15_t *pB = im_buffer; 00148 const q15_t *pB2 = pB + ch_im_in * dim_kernel * dim_kernel; 00149 00150 /* aling the second pointer for A */ 00151 const q15_t *pA2 = pA + ch_im_in * dim_kernel * dim_kernel; 00152 00153 /* init the sum with bias */ 00154 q31_t sum = ((q31_t)bias[i] << bias_shift) + NN_ROUND(out_shift); 00155 q31_t sum2 = ((q31_t)bias[i] << bias_shift) + NN_ROUND(out_shift); 00156 q31_t sum3 = ((q31_t)bias[i + 1] << bias_shift) + NN_ROUND(out_shift); 00157 q31_t sum4 = ((q31_t)bias[i + 1] << bias_shift) + NN_ROUND(out_shift); 00158 00159 uint16_t colCnt = ch_im_in * dim_kernel * dim_kernel >> 1; 00160 /* accumulate over the vector */ 00161 while (colCnt) 00162 { 00163 q31_t inA1 = *__SIMD32(pA)++; 00164 q31_t inB1 = *__SIMD32(pB)++; 00165 q31_t inA2 = *__SIMD32(pA2)++; 00166 q31_t inB2 = *__SIMD32(pB2)++; 00167 00168 sum = __SMLAD(inA1, inB1, sum); 00169 sum2 = __SMLAD(inA1, inB2, sum2); 00170 sum3 = __SMLAD(inA2, inB1, sum3); 00171 sum4 = __SMLAD(inA2, inB2, sum4); 00172 00173 colCnt--; 00174 } /* while over colCnt */ 00175 colCnt = ch_im_in * dim_kernel * dim_kernel & 0x1; 00176 while (colCnt) 00177 { 00178 q15_t inA1 = *pA++; 00179 q15_t inB1 = *pB++; 00180 q15_t inA2 = *pA2++; 00181 q15_t inB2 = *pB2++; 00182 00183 sum += inA1 * inB1; 00184 sum2 += inA1 * inB2; 00185 sum3 += inA2 * inB1; 00186 sum4 += inA2 * inB2; 00187 colCnt--; 00188 } /* while over colCnt */ 00189 *pOut++ = (q15_t) __SSAT(sum >> out_shift, 16); 00190 *pOut++ = (q15_t) __SSAT(sum3 >> out_shift, 16); 00191 *pOut2++ = (q15_t) __SSAT(sum2 >> out_shift, 16); 00192 *pOut2++ = (q15_t) __SSAT(sum4 >> out_shift, 16); 00193 00194 /* skip the row computed with A2 */ 00195 pA += ch_im_in * dim_kernel * dim_kernel; 00196 } /* for over ch_im_out */ 00197 00198 pOut += ch_im_out; 00199 /* counter reset */ 00200 pBuffer = im_buffer; 00201 } 00202 } 00203 } 00204 00205 #else 00206 /* Run the following code as reference implementation for Cortex-M0 and Cortex-M3 */ 00207 uint16_t i, j, k, l, m, n; 00208 int conv_out; 00209 signed char in_row, in_col; 00210 00211 if (ch_im_in % 2 != 0 || ch_im_out % 2 != 0) 00212 { 00213 /* check if the input dimension meets the constraints */ 00214 return ARM_MATH_SIZE_MISMATCH; 00215 } 00216 00217 for (i = 0; i < ch_im_out; i++) 00218 { 00219 for (j = 0; j < dim_im_out; j++) 00220 { 00221 for (k = 0; k < dim_im_out; k++) 00222 { 00223 conv_out = ((q31_t)bias[i] << bias_shift) + NN_ROUND(out_shift); 00224 for (m = 0; m < dim_kernel; m++) 00225 { 00226 for (n = 0; n < dim_kernel; n++) 00227 { 00228 in_row = stride * j + m - padding; 00229 in_col = stride * k + n - padding; 00230 if (in_row >= 0 && in_col >= 0 && in_row < dim_im_in && in_col < dim_im_in) 00231 { 00232 for (l = 0; l < ch_im_in; l++) 00233 { 00234 conv_out += 00235 Im_in[(in_row * dim_im_in + in_col) * ch_im_in + 00236 l] * wt[i * ch_im_in * dim_kernel * dim_kernel + (m * dim_kernel + 00237 n) * ch_im_in + l]; 00238 } 00239 } 00240 } 00241 } 00242 Im_out[i + (j * dim_im_out + k) * ch_im_out] = (q15_t) __SSAT((conv_out >> out_shift), 16); 00243 } 00244 } 00245 } 00246 00247 #endif /* ARM_MATH_DSP */ 00248 00249 /* Return to application */ 00250 return ARM_MATH_SUCCESS; 00251 } 00252 00253 /** 00254 * @} end of NNConv group 00255 */ 00256
Generated on Tue Jul 12 2022 16:46:23 by
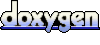