Aded CMSIS5 DSP and NN folder. Needs some work
Embed:
(wiki syntax)
Show/hide line numbers
arm_cmplx_conj_f32.c
00001 /* ---------------------------------------------------------------------- 00002 * Project: CMSIS DSP Library 00003 * Title: arm_cmplx_conj_f32.c 00004 * Description: Floating-point complex conjugate 00005 * 00006 * $Date: 27. January 2017 00007 * $Revision: V.1.5.1 00008 * 00009 * Target Processor: Cortex-M cores 00010 * -------------------------------------------------------------------- */ 00011 /* 00012 * Copyright (C) 2010-2017 ARM Limited or its affiliates. All rights reserved. 00013 * 00014 * SPDX-License-Identifier: Apache-2.0 00015 * 00016 * Licensed under the Apache License, Version 2.0 (the License); you may 00017 * not use this file except in compliance with the License. 00018 * You may obtain a copy of the License at 00019 * 00020 * www.apache.org/licenses/LICENSE-2.0 00021 * 00022 * Unless required by applicable law or agreed to in writing, software 00023 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00024 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00025 * See the License for the specific language governing permissions and 00026 * limitations under the License. 00027 */ 00028 00029 #include "arm_math.h" 00030 00031 /** 00032 * @ingroup groupCmplxMath 00033 */ 00034 00035 /** 00036 * @defgroup cmplx_conj Complex Conjugate 00037 * 00038 * Conjugates the elements of a complex data vector. 00039 * 00040 * The <code>pSrc</code> points to the source data and 00041 * <code>pDst</code> points to the where the result should be written. 00042 * <code>numSamples</code> specifies the number of complex samples 00043 * and the data in each array is stored in an interleaved fashion 00044 * (real, imag, real, imag, ...). 00045 * Each array has a total of <code>2*numSamples</code> values. 00046 * The underlying algorithm is used: 00047 * 00048 * <pre> 00049 * for(n=0; n<numSamples; n++) { 00050 * pDst[(2*n)+0)] = pSrc[(2*n)+0]; // real part 00051 * pDst[(2*n)+1)] = -pSrc[(2*n)+1]; // imag part 00052 * } 00053 * </pre> 00054 * 00055 * There are separate functions for floating-point, Q15, and Q31 data types. 00056 */ 00057 00058 /** 00059 * @addtogroup cmplx_conj 00060 * @{ 00061 */ 00062 00063 /** 00064 * @brief Floating-point complex conjugate. 00065 * @param *pSrc points to the input vector 00066 * @param *pDst points to the output vector 00067 * @param numSamples number of complex samples in each vector 00068 * @return none. 00069 */ 00070 00071 void arm_cmplx_conj_f32( 00072 float32_t * pSrc, 00073 float32_t * pDst, 00074 uint32_t numSamples) 00075 { 00076 uint32_t blkCnt; /* loop counter */ 00077 00078 #if defined (ARM_MATH_DSP) 00079 00080 /* Run the below code for Cortex-M4 and Cortex-M3 */ 00081 float32_t inR1, inR2, inR3, inR4; 00082 float32_t inI1, inI2, inI3, inI4; 00083 00084 /*loop Unrolling */ 00085 blkCnt = numSamples >> 2U; 00086 00087 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00088 ** a second loop below computes the remaining 1 to 3 samples. */ 00089 while (blkCnt > 0U) 00090 { 00091 /* C[0]+jC[1] = A[0]+ j (-1) A[1] */ 00092 /* Calculate Complex Conjugate and then store the results in the destination buffer. */ 00093 /* read real input samples */ 00094 inR1 = pSrc[0]; 00095 /* store real samples to destination */ 00096 pDst[0] = inR1; 00097 inR2 = pSrc[2]; 00098 pDst[2] = inR2; 00099 inR3 = pSrc[4]; 00100 pDst[4] = inR3; 00101 inR4 = pSrc[6]; 00102 pDst[6] = inR4; 00103 00104 /* read imaginary input samples */ 00105 inI1 = pSrc[1]; 00106 inI2 = pSrc[3]; 00107 00108 /* conjugate input */ 00109 inI1 = -inI1; 00110 00111 /* read imaginary input samples */ 00112 inI3 = pSrc[5]; 00113 00114 /* conjugate input */ 00115 inI2 = -inI2; 00116 00117 /* read imaginary input samples */ 00118 inI4 = pSrc[7]; 00119 00120 /* conjugate input */ 00121 inI3 = -inI3; 00122 00123 /* store imaginary samples to destination */ 00124 pDst[1] = inI1; 00125 pDst[3] = inI2; 00126 00127 /* conjugate input */ 00128 inI4 = -inI4; 00129 00130 /* store imaginary samples to destination */ 00131 pDst[5] = inI3; 00132 00133 /* increment source pointer by 8 to process next sampels */ 00134 pSrc += 8U; 00135 00136 /* store imaginary sample to destination */ 00137 pDst[7] = inI4; 00138 00139 /* increment destination pointer by 8 to store next samples */ 00140 pDst += 8U; 00141 00142 /* Decrement the loop counter */ 00143 blkCnt--; 00144 } 00145 00146 /* If the numSamples is not a multiple of 4, compute any remaining output samples here. 00147 ** No loop unrolling is used. */ 00148 blkCnt = numSamples % 0x4U; 00149 00150 #else 00151 00152 /* Run the below code for Cortex-M0 */ 00153 blkCnt = numSamples; 00154 00155 #endif /* #if defined (ARM_MATH_DSP) */ 00156 00157 while (blkCnt > 0U) 00158 { 00159 /* realOut + j (imagOut) = realIn + j (-1) imagIn */ 00160 /* Calculate Complex Conjugate and then store the results in the destination buffer. */ 00161 *pDst++ = *pSrc++; 00162 *pDst++ = -*pSrc++; 00163 00164 /* Decrement the loop counter */ 00165 blkCnt--; 00166 } 00167 } 00168 00169 /** 00170 * @} end of cmplx_conj group 00171 */ 00172
Generated on Tue Jul 12 2022 16:46:23 by
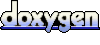