Aded CMSIS5 DSP and NN folder. Needs some work
Embed:
(wiki syntax)
Show/hide line numbers
arm_bitreversal.c
00001 /* ---------------------------------------------------------------------- 00002 * Project: CMSIS DSP Library 00003 * Title: arm_bitreversal.c 00004 * Description: Bitreversal functions 00005 * 00006 * $Date: 27. January 2017 00007 * $Revision: V.1.5.1 00008 * 00009 * Target Processor: Cortex-M cores 00010 * -------------------------------------------------------------------- */ 00011 /* 00012 * Copyright (C) 2010-2017 ARM Limited or its affiliates. All rights reserved. 00013 * 00014 * SPDX-License-Identifier: Apache-2.0 00015 * 00016 * Licensed under the Apache License, Version 2.0 (the License); you may 00017 * not use this file except in compliance with the License. 00018 * You may obtain a copy of the License at 00019 * 00020 * www.apache.org/licenses/LICENSE-2.0 00021 * 00022 * Unless required by applicable law or agreed to in writing, software 00023 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00024 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00025 * See the License for the specific language governing permissions and 00026 * limitations under the License. 00027 */ 00028 00029 #include "arm_math.h" 00030 #include "arm_common_tables.h" 00031 00032 /* 00033 * @brief In-place bit reversal function. 00034 * @param[in, out] *pSrc points to the in-place buffer of floating-point data type. 00035 * @param[in] fftSize length of the FFT. 00036 * @param[in] bitRevFactor bit reversal modifier that supports different size FFTs with the same bit reversal table. 00037 * @param[in] *pBitRevTab points to the bit reversal table. 00038 * @return none. 00039 */ 00040 00041 void arm_bitreversal_f32( 00042 float32_t * pSrc, 00043 uint16_t fftSize, 00044 uint16_t bitRevFactor, 00045 uint16_t * pBitRevTab) 00046 { 00047 uint16_t fftLenBy2, fftLenBy2p1; 00048 uint16_t i, j; 00049 float32_t in; 00050 00051 /* Initializations */ 00052 j = 0U; 00053 fftLenBy2 = fftSize >> 1U; 00054 fftLenBy2p1 = (fftSize >> 1U) + 1U; 00055 00056 /* Bit Reversal Implementation */ 00057 for (i = 0U; i <= (fftLenBy2 - 2U); i += 2U) 00058 { 00059 if (i < j) 00060 { 00061 /* pSrc[i] <-> pSrc[j]; */ 00062 in = pSrc[2U * i]; 00063 pSrc[2U * i] = pSrc[2U * j]; 00064 pSrc[2U * j] = in; 00065 00066 /* pSrc[i+1U] <-> pSrc[j+1U] */ 00067 in = pSrc[(2U * i) + 1U]; 00068 pSrc[(2U * i) + 1U] = pSrc[(2U * j) + 1U]; 00069 pSrc[(2U * j) + 1U] = in; 00070 00071 /* pSrc[i+fftLenBy2p1] <-> pSrc[j+fftLenBy2p1] */ 00072 in = pSrc[2U * (i + fftLenBy2p1)]; 00073 pSrc[2U * (i + fftLenBy2p1)] = pSrc[2U * (j + fftLenBy2p1)]; 00074 pSrc[2U * (j + fftLenBy2p1)] = in; 00075 00076 /* pSrc[i+fftLenBy2p1+1U] <-> pSrc[j+fftLenBy2p1+1U] */ 00077 in = pSrc[(2U * (i + fftLenBy2p1)) + 1U]; 00078 pSrc[(2U * (i + fftLenBy2p1)) + 1U] = 00079 pSrc[(2U * (j + fftLenBy2p1)) + 1U]; 00080 pSrc[(2U * (j + fftLenBy2p1)) + 1U] = in; 00081 00082 } 00083 00084 /* pSrc[i+1U] <-> pSrc[j+1U] */ 00085 in = pSrc[2U * (i + 1U)]; 00086 pSrc[2U * (i + 1U)] = pSrc[2U * (j + fftLenBy2)]; 00087 pSrc[2U * (j + fftLenBy2)] = in; 00088 00089 /* pSrc[i+2U] <-> pSrc[j+2U] */ 00090 in = pSrc[(2U * (i + 1U)) + 1U]; 00091 pSrc[(2U * (i + 1U)) + 1U] = pSrc[(2U * (j + fftLenBy2)) + 1U]; 00092 pSrc[(2U * (j + fftLenBy2)) + 1U] = in; 00093 00094 /* Reading the index for the bit reversal */ 00095 j = *pBitRevTab; 00096 00097 /* Updating the bit reversal index depending on the fft length */ 00098 pBitRevTab += bitRevFactor; 00099 } 00100 } 00101 00102 00103 00104 /* 00105 * @brief In-place bit reversal function. 00106 * @param[in, out] *pSrc points to the in-place buffer of Q31 data type. 00107 * @param[in] fftLen length of the FFT. 00108 * @param[in] bitRevFactor bit reversal modifier that supports different size FFTs with the same bit reversal table 00109 * @param[in] *pBitRevTab points to bit reversal table. 00110 * @return none. 00111 */ 00112 00113 void arm_bitreversal_q31( 00114 q31_t * pSrc, 00115 uint32_t fftLen, 00116 uint16_t bitRevFactor, 00117 uint16_t * pBitRevTable) 00118 { 00119 uint32_t fftLenBy2, fftLenBy2p1, i, j; 00120 q31_t in; 00121 00122 /* Initializations */ 00123 j = 0U; 00124 fftLenBy2 = fftLen / 2U; 00125 fftLenBy2p1 = (fftLen / 2U) + 1U; 00126 00127 /* Bit Reversal Implementation */ 00128 for (i = 0U; i <= (fftLenBy2 - 2U); i += 2U) 00129 { 00130 if (i < j) 00131 { 00132 /* pSrc[i] <-> pSrc[j]; */ 00133 in = pSrc[2U * i]; 00134 pSrc[2U * i] = pSrc[2U * j]; 00135 pSrc[2U * j] = in; 00136 00137 /* pSrc[i+1U] <-> pSrc[j+1U] */ 00138 in = pSrc[(2U * i) + 1U]; 00139 pSrc[(2U * i) + 1U] = pSrc[(2U * j) + 1U]; 00140 pSrc[(2U * j) + 1U] = in; 00141 00142 /* pSrc[i+fftLenBy2p1] <-> pSrc[j+fftLenBy2p1] */ 00143 in = pSrc[2U * (i + fftLenBy2p1)]; 00144 pSrc[2U * (i + fftLenBy2p1)] = pSrc[2U * (j + fftLenBy2p1)]; 00145 pSrc[2U * (j + fftLenBy2p1)] = in; 00146 00147 /* pSrc[i+fftLenBy2p1+1U] <-> pSrc[j+fftLenBy2p1+1U] */ 00148 in = pSrc[(2U * (i + fftLenBy2p1)) + 1U]; 00149 pSrc[(2U * (i + fftLenBy2p1)) + 1U] = 00150 pSrc[(2U * (j + fftLenBy2p1)) + 1U]; 00151 pSrc[(2U * (j + fftLenBy2p1)) + 1U] = in; 00152 00153 } 00154 00155 /* pSrc[i+1U] <-> pSrc[j+1U] */ 00156 in = pSrc[2U * (i + 1U)]; 00157 pSrc[2U * (i + 1U)] = pSrc[2U * (j + fftLenBy2)]; 00158 pSrc[2U * (j + fftLenBy2)] = in; 00159 00160 /* pSrc[i+2U] <-> pSrc[j+2U] */ 00161 in = pSrc[(2U * (i + 1U)) + 1U]; 00162 pSrc[(2U * (i + 1U)) + 1U] = pSrc[(2U * (j + fftLenBy2)) + 1U]; 00163 pSrc[(2U * (j + fftLenBy2)) + 1U] = in; 00164 00165 /* Reading the index for the bit reversal */ 00166 j = *pBitRevTable; 00167 00168 /* Updating the bit reversal index depending on the fft length */ 00169 pBitRevTable += bitRevFactor; 00170 } 00171 } 00172 00173 00174 00175 /* 00176 * @brief In-place bit reversal function. 00177 * @param[in, out] *pSrc points to the in-place buffer of Q15 data type. 00178 * @param[in] fftLen length of the FFT. 00179 * @param[in] bitRevFactor bit reversal modifier that supports different size FFTs with the same bit reversal table 00180 * @param[in] *pBitRevTab points to bit reversal table. 00181 * @return none. 00182 */ 00183 00184 void arm_bitreversal_q15( 00185 q15_t * pSrc16, 00186 uint32_t fftLen, 00187 uint16_t bitRevFactor, 00188 uint16_t * pBitRevTab) 00189 { 00190 q31_t *pSrc = (q31_t *) pSrc16; 00191 q31_t in; 00192 uint32_t fftLenBy2, fftLenBy2p1; 00193 uint32_t i, j; 00194 00195 /* Initializations */ 00196 j = 0U; 00197 fftLenBy2 = fftLen / 2U; 00198 fftLenBy2p1 = (fftLen / 2U) + 1U; 00199 00200 /* Bit Reversal Implementation */ 00201 for (i = 0U; i <= (fftLenBy2 - 2U); i += 2U) 00202 { 00203 if (i < j) 00204 { 00205 /* pSrc[i] <-> pSrc[j]; */ 00206 /* pSrc[i+1U] <-> pSrc[j+1U] */ 00207 in = pSrc[i]; 00208 pSrc[i] = pSrc[j]; 00209 pSrc[j] = in; 00210 00211 /* pSrc[i + fftLenBy2p1] <-> pSrc[j + fftLenBy2p1]; */ 00212 /* pSrc[i + fftLenBy2p1+1U] <-> pSrc[j + fftLenBy2p1+1U] */ 00213 in = pSrc[i + fftLenBy2p1]; 00214 pSrc[i + fftLenBy2p1] = pSrc[j + fftLenBy2p1]; 00215 pSrc[j + fftLenBy2p1] = in; 00216 } 00217 00218 /* pSrc[i+1U] <-> pSrc[j+fftLenBy2]; */ 00219 /* pSrc[i+2] <-> pSrc[j+fftLenBy2+1U] */ 00220 in = pSrc[i + 1U]; 00221 pSrc[i + 1U] = pSrc[j + fftLenBy2]; 00222 pSrc[j + fftLenBy2] = in; 00223 00224 /* Reading the index for the bit reversal */ 00225 j = *pBitRevTab; 00226 00227 /* Updating the bit reversal index depending on the fft length */ 00228 pBitRevTab += bitRevFactor; 00229 } 00230 } 00231
Generated on Tue Jul 12 2022 16:46:22 by
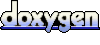