Aded CMSIS5 DSP and NN folder. Needs some work
Embed:
(wiki syntax)
Show/hide line numbers
arm_biquad_cascade_df2T_init_f32.c
00001 /* ---------------------------------------------------------------------- 00002 * Project: CMSIS DSP Library 00003 * Title: arm_biquad_cascade_df2T_init_f32.c 00004 * Description: Initialization function for floating-point transposed direct form II Biquad cascade filter 00005 * 00006 * $Date: 27. January 2017 00007 * $Revision: V.1.5.1 00008 * 00009 * Target Processor: Cortex-M cores 00010 * -------------------------------------------------------------------- */ 00011 /* 00012 * Copyright (C) 2010-2017 ARM Limited or its affiliates. All rights reserved. 00013 * 00014 * SPDX-License-Identifier: Apache-2.0 00015 * 00016 * Licensed under the Apache License, Version 2.0 (the License); you may 00017 * not use this file except in compliance with the License. 00018 * You may obtain a copy of the License at 00019 * 00020 * www.apache.org/licenses/LICENSE-2.0 00021 * 00022 * Unless required by applicable law or agreed to in writing, software 00023 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00024 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00025 * See the License for the specific language governing permissions and 00026 * limitations under the License. 00027 */ 00028 00029 #include "arm_math.h" 00030 00031 /** 00032 * @ingroup groupFilters 00033 */ 00034 00035 /** 00036 * @addtogroup BiquadCascadeDF2T 00037 * @{ 00038 */ 00039 00040 /** 00041 * @brief Initialization function for the floating-point transposed direct form II Biquad cascade filter. 00042 * @param[in,out] *S points to an instance of the filter data structure. 00043 * @param[in] numStages number of 2nd order stages in the filter. 00044 * @param[in] *pCoeffs points to the filter coefficients. 00045 * @param[in] *pState points to the state buffer. 00046 * @return none 00047 * 00048 * <b>Coefficient and State Ordering:</b> 00049 * \par 00050 * The coefficients are stored in the array <code>pCoeffs</code> in the following order: 00051 * <pre> 00052 * {b10, b11, b12, a11, a12, b20, b21, b22, a21, a22, ...} 00053 * </pre> 00054 * 00055 * \par 00056 * where <code>b1x</code> and <code>a1x</code> are the coefficients for the first stage, 00057 * <code>b2x</code> and <code>a2x</code> are the coefficients for the second stage, 00058 * and so on. The <code>pCoeffs</code> array contains a total of <code>5*numStages</code> values. 00059 * 00060 * \par 00061 * The <code>pState</code> is a pointer to state array. 00062 * Each Biquad stage has 2 state variables <code>d1,</code> and <code>d2</code>. 00063 * The 2 state variables for stage 1 are first, then the 2 state variables for stage 2, and so on. 00064 * The state array has a total length of <code>2*numStages</code> values. 00065 * The state variables are updated after each block of data is processed; the coefficients are untouched. 00066 */ 00067 00068 void arm_biquad_cascade_df2T_init_f32( 00069 arm_biquad_cascade_df2T_instance_f32 * S, 00070 uint8_t numStages, 00071 float32_t * pCoeffs, 00072 float32_t * pState) 00073 { 00074 /* Assign filter stages */ 00075 S->numStages = numStages; 00076 00077 /* Assign coefficient pointer */ 00078 S->pCoeffs = pCoeffs; 00079 00080 /* Clear state buffer and size is always 2 * numStages */ 00081 memset(pState, 0, (2U * (uint32_t) numStages) * sizeof(float32_t)); 00082 00083 /* Assign state pointer */ 00084 S->pState = pState; 00085 } 00086 00087 /** 00088 * @} end of BiquadCascadeDF2T group 00089 */ 00090
Generated on Tue Jul 12 2022 16:46:22 by
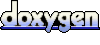