Aded CMSIS5 DSP and NN folder. Needs some work
Embed:
(wiki syntax)
Show/hide line numbers
arm_biquad_cascade_df1_init_q15.c
00001 /* ---------------------------------------------------------------------- 00002 * Project: CMSIS DSP Library 00003 * Title: arm_biquad_cascade_df1_init_q15.c 00004 * Description: Q15 Biquad cascade DirectFormI(DF1) filter initialization function 00005 * 00006 * $Date: 27. January 2017 00007 * $Revision: V.1.5.1 00008 * 00009 * Target Processor: Cortex-M cores 00010 * -------------------------------------------------------------------- */ 00011 /* 00012 * Copyright (C) 2010-2017 ARM Limited or its affiliates. All rights reserved. 00013 * 00014 * SPDX-License-Identifier: Apache-2.0 00015 * 00016 * Licensed under the Apache License, Version 2.0 (the License); you may 00017 * not use this file except in compliance with the License. 00018 * You may obtain a copy of the License at 00019 * 00020 * www.apache.org/licenses/LICENSE-2.0 00021 * 00022 * Unless required by applicable law or agreed to in writing, software 00023 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00024 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00025 * See the License for the specific language governing permissions and 00026 * limitations under the License. 00027 */ 00028 00029 #include "arm_math.h" 00030 00031 /** 00032 * @ingroup groupFilters 00033 */ 00034 00035 /** 00036 * @addtogroup BiquadCascadeDF1 00037 * @{ 00038 */ 00039 00040 /** 00041 * @details 00042 * 00043 * @param[in,out] *S points to an instance of the Q15 Biquad cascade structure. 00044 * @param[in] numStages number of 2nd order stages in the filter. 00045 * @param[in] *pCoeffs points to the filter coefficients. 00046 * @param[in] *pState points to the state buffer. 00047 * @param[in] postShift Shift to be applied to the accumulator result. Varies according to the coefficients format 00048 * @return none 00049 * 00050 * <b>Coefficient and State Ordering:</b> 00051 * 00052 * \par 00053 * The coefficients are stored in the array <code>pCoeffs</code> in the following order: 00054 * <pre> 00055 * {b10, 0, b11, b12, a11, a12, b20, 0, b21, b22, a21, a22, ...} 00056 * </pre> 00057 * where <code>b1x</code> and <code>a1x</code> are the coefficients for the first stage, 00058 * <code>b2x</code> and <code>a2x</code> are the coefficients for the second stage, 00059 * and so on. The <code>pCoeffs</code> array contains a total of <code>6*numStages</code> values. 00060 * The zero coefficient between <code>b1</code> and <code>b2</code> facilities use of 16-bit SIMD instructions on the Cortex-M4. 00061 * 00062 * \par 00063 * The state variables are stored in the array <code>pState</code>. 00064 * Each Biquad stage has 4 state variables <code>x[n-1], x[n-2], y[n-1],</code> and <code>y[n-2]</code>. 00065 * The state variables are arranged in the <code>pState</code> array as: 00066 * <pre> 00067 * {x[n-1], x[n-2], y[n-1], y[n-2]} 00068 * </pre> 00069 * The 4 state variables for stage 1 are first, then the 4 state variables for stage 2, and so on. 00070 * The state array has a total length of <code>4*numStages</code> values. 00071 * The state variables are updated after each block of data is processed; the coefficients are untouched. 00072 */ 00073 00074 void arm_biquad_cascade_df1_init_q15( 00075 arm_biquad_casd_df1_inst_q15 * S, 00076 uint8_t numStages, 00077 q15_t * pCoeffs, 00078 q15_t * pState, 00079 int8_t postShift) 00080 { 00081 /* Assign filter stages */ 00082 S->numStages = numStages; 00083 00084 /* Assign postShift to be applied to the output */ 00085 S->postShift = postShift; 00086 00087 /* Assign coefficient pointer */ 00088 S->pCoeffs = pCoeffs; 00089 00090 /* Clear state buffer and size is always 4 * numStages */ 00091 memset(pState, 0, (4U * (uint32_t) numStages) * sizeof(q15_t)); 00092 00093 /* Assign state pointer */ 00094 S->pState = pState; 00095 } 00096 00097 /** 00098 * @} end of BiquadCascadeDF1 group 00099 */ 00100
Generated on Tue Jul 12 2022 16:46:22 by
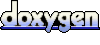