test
Dependencies: FATFileSystem MPU9250_SPI mbed-src
Fork of SDFileSystem by
main.cpp
00001 #include "SDFileSystem.h" 00002 #include "MPU9250.h" 00003 #include "InterruptManager.h" 00004 00005 #define BLENANO 00006 00007 /* Pin Definitions */ 00008 #ifdef BLENANO 00009 #define MOSI p9 00010 #define MISO p11 00011 #define SCLK p8 00012 #define CS_SD p10 00013 #define CS_MPU p29 00014 #define BUTTON p28 00015 #define MIC p5 00016 #define LOAD_CELL p4 00017 #define LED p15 00018 #else 00019 #define MOSI p20 00020 #define MISO p22 00021 #define SCLK p25 00022 #define CS_SD p23 00023 #define CS_MPU p21 00024 #define BUTTON p17 00025 #define MIC p5 00026 #define LOAD_CELL p4 00027 #define LED p15 00028 #endif 00029 00030 /* Un/comment to determine which tests will be run */ 00031 #define IMU_TEST 00032 //#define MIC_TEST 00033 //#define LOAD_TEST 00034 00035 /* Parameters for sampling */ 00036 #define FILE_NAME "trick" 00037 #define SAMPLE_RATE 1000 00038 00039 /* Objects used */ 00040 DigitalOut led(LED); 00041 AnalogIn mic(MIC); 00042 InterruptIn button(BUTTON); 00043 Ticker timer; 00044 SPI spi(MOSI, MISO, SCLK); 00045 SDFileSystem sd(MOSI, MISO, SCLK, CS_SD, "sd"); 00046 mpu9250_spi imu(spi , CS_MPU); 00047 00048 /* Flags for sampling */ 00049 #define IMU_FLAG (1<<1U) 00050 #define MIC_FLAG (1<<2U) 00051 #define CREATE_FILE (1<<3U) 00052 #define CLOSE_FILE (1<<4U) 00053 00054 /* Status */ 00055 #define START_SAMPLE 1 00056 #define END_SAMPLE 0 00057 00058 static uint16_t status = 0; 00059 static int button_status = START_SAMPLE; 00060 static uint16_t file_count = 0; 00061 00062 static float sample_rate = (1/(float)SAMPLE_RATE) ; 00063 00064 static FILE *fp = NULL; 00065 static char file_name[17]; 00066 00067 static void openNextFile(void) 00068 { 00069 00070 if( fp ){ 00071 fclose(fp); 00072 } 00073 00074 snprintf(file_name, sizeof(file_name), "/sd/%s_%02d.txt", FILE_NAME, file_count++); 00075 fp = fopen(file_name, "w"); 00076 fprintf(fp, "Sample Rate: %d Hz\n", SAMPLE_RATE); 00077 00078 } 00079 00080 static void button_interrupt(void) 00081 { 00082 00083 //CREATE FILE 00084 if ( button_status == START_SAMPLE ){ 00085 status |= CREATE_FILE; 00086 button_status = END_SAMPLE; 00087 00088 //CLOSE FILE 00089 } else if ( button_status == END_SAMPLE ){ 00090 status |= CLOSE_FILE; 00091 button_status = START_SAMPLE; 00092 } 00093 00094 } 00095 00096 static void timer_interrupt(void) 00097 { 00098 00099 if ( button_status == END_SAMPLE ){ 00100 //ENABLE SAMPLE FLAGS 00101 #ifdef IMU_TEST 00102 status |= IMU_FLAG; 00103 #endif 00104 00105 #ifdef MIC_TEST 00106 status |= MIC_FLAG; 00107 #endif 00108 00109 #ifdef LOAD_TEST 00110 status |= LOAD_FLAG; 00111 #endif 00112 } 00113 00114 } 00115 00116 int main() 00117 { 00118 00119 #ifdef IMU_TEST 00120 //initialize imu 00121 imu.init(1, BITS_DLPF_CFG_2100HZ_NOLPF); 00122 #endif 00123 00124 //set up button as active low to trigger file creation 00125 button.fall(&button_interrupt); 00126 timer.attach(&timer_interrupt, sample_rate ); 00127 led.write(0); 00128 00129 snprintf(file_name, sizeof(file_name), "/sd/%s_%02d.txt", FILE_NAME, file_count++); 00130 fp = fopen(file_name, "w"); 00131 fprintf(fp, "Sample Rate: %d Hz\n", SAMPLE_RATE); 00132 00133 while(1){ 00134 00135 if ( status & CREATE_FILE ){ 00136 led.write(1); 00137 openNextFile(); 00138 status ^= CREATE_FILE; 00139 } 00140 00141 #ifdef IMU_TEST 00142 if ( status & IMU_FLAG ){ 00143 led.write(!led.read()); 00144 imu.read_all(); 00145 00146 #ifndef MIC_TEST 00147 fprintf(fp, "%5d\t%5d\t%5d\t%5d\t%5d\t%5d\t%5d\t%5d\t%5d\n", (signed int) imu.gyroscope_data[0], 00148 (signed int) imu.gyroscope_data[1], 00149 (signed int) imu.gyroscope_data[2], 00150 (signed int) imu.accelerometer_data[0], 00151 (signed int) imu.accelerometer_data[1], 00152 (signed int) imu.accelerometer_data[2], 00153 (signed int) imu.Magnetometer[0], 00154 (signed int) imu.Magnetometer[1], 00155 (signed int) imu.Magnetometer[2] ); 00156 #endif 00157 00158 status ^= IMU_FLAG; 00159 } 00160 #endif 00161 00162 #ifdef MIC_TEST 00163 if ( status & MIC_FLAG ){ 00164 led.write(!led.read()); 00165 00166 #ifndef IMU_TEST 00167 fprintf(fp, "%d\n", mic.read_u16()); 00168 00169 #else 00170 fprintf(fp, "%5d\t%5d\t%5d\t%5d\t%5d\t%5d\t%5d\t%5d\t%5d\t%4d\n", (signed int) imu.gyroscope_data[0], 00171 (signed int) imu.gyroscope_data[1], 00172 (signed int) imu.gyroscope_data[2], 00173 (signed int) imu.accelerometer_data[0], 00174 (signed int) imu.accelerometer_data[1], 00175 (signed int) imu.accelerometer_data[2], 00176 (signed int) imu.Magnetometer[0], 00177 (signed int) imu.Magnetometer[1], 00178 (signed int) imu.Magnetometer[2], 00179 mic.read_u16() ); 00180 #endif 00181 00182 status ^= MIC_FLAG; 00183 00184 } 00185 #endif 00186 00187 if ( status & CLOSE_FILE ){ 00188 led.write(0); 00189 fclose(fp); 00190 status ^= CLOSE_FILE; 00191 } 00192 00193 } 00194 00195 } 00196
Generated on Thu Jul 14 2022 17:23:23 by
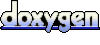