
mbed I2C LCD draw bitmap
Embed:
(wiki syntax)
Show/hide line numbers
ssd1306.h
00001 #define LCD_Xmax 128 00002 #define LCD_Ymax 64 00003 #define FG_COLOR 0xFFFF 00004 #define BG_COLOR 0x0000 00005 00006 #define SSD1306_slave_addr 0x78 00007 00008 class SSD1306 { 00009 public: 00010 void initialize(void); 00011 void clearscreen(void); 00012 void printC_5x7(int x, int y, unsigned char ascii_code); 00013 void printC(int x, int y, unsigned char ascii_code); 00014 void printLine(int line, char text[]); 00015 void printS(int x, int y, char text[]); 00016 void printS_5x7(int x, int y, char text[]); 00017 void drawPixel(int x, int y, int fgColor, int bgColor); 00018 void drawBmp8x8(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]); 00019 void drawBmp32x8(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]); 00020 void drawBmp120x8(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]); 00021 void drawBmp8x16(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]); 00022 void drawBmp16x8(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]); 00023 void drawBmp16x16(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]); 00024 void drawBmp16x24(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]); 00025 void drawBmp16x32(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]); 00026 void drawBmp16x40(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]); 00027 void drawBmp16x48(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]); 00028 void drawBmp16x64(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]); 00029 void drawBmp32x16(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]); 00030 void drawBmp32x32(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]); 00031 void drawBmp32x48(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]); 00032 void drawBmp32x64(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]); 00033 void drawBmp64x64(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]); 00034 void drawBMP(unsigned char *buffer); 00035 };
Generated on Fri Jul 15 2022 21:50:58 by
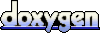