coursework
Embed:
(wiki syntax)
Show/hide line numbers
signal.h
00001 #ifndef _SIGNAL_H 00002 #define _SIGNAL_H 00003 00004 enum SIGNAL_TYPE { 00005 NONE, 00006 CONSTANT, 00007 SINE, 00008 SQUARE 00009 }; 00010 00011 class Signal { 00012 public: 00013 enum SIGNAL_TYPE type; 00014 float amplitude; 00015 float frequency; 00016 00017 Signal(enum SIGNAL_TYPE type = NONE, 00018 float amplitude = 0, 00019 float frequency = 0) : 00020 type(type), amplitude(amplitude), frequency(frequency) {} 00021 00022 void set(enum SIGNAL_TYPE _type, float _amplitude, float _frequency) { 00023 type=_type; amplitude=_amplitude; frequency=_frequency; } 00024 00025 bool operator== (const Signal & s) { 00026 return type==s.type && amplitude==s.amplitude && frequency==s.frequency; } 00027 00028 const char* c_str (char* str); 00029 }; 00030 00031 #endif 00032
Generated on Thu Jul 14 2022 20:41:36 by
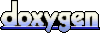