Code for my numitron clock. Based on the STM32F042 and using a TLC5916 per numitron to drive them.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "SWSPI.h" 00003 00004 SWSPI spi( PA_2, PA_1, PA_3 ); // create the SPI port 00005 DigitalOut OE(PA_0); 00006 DigitalOut LE(PA_4); // the LE pin and the number of connected chips 00007 DigitalIn B1(PA_5), B2(PA_6), B3(PA_7), B4(PB_1); //B1 is the most left button 00008 BusIn buttons(PA_5, PA_6, PA_7, PB_1); 00009 const uint8_t numbers[10] = {0x7B, 0x60, 0x57, 0x76, 0x6C, 0x3E, 0x3F, 0x70, 0x7F, 0x7E}; 00010 uint8_t flip = 1; 00011 00012 uint8_t SetSysClock_PLL_HSE_16M(void); 00013 00014 RTC_HandleTypeDef hrtc; 00015 HAL_StatusTypeDef status; 00016 void SystemClock_Config(void); 00017 static void MX_RTC_Init(void); 00018 00019 typedef struct 00020 { 00021 uint8_t second_right; 00022 uint8_t second_left; 00023 uint8_t minute_right; 00024 uint8_t minute_left; 00025 uint8_t hour_right; 00026 uint8_t hour_left; 00027 }clock_typ; 00028 00029 struct tm t; 00030 time_t rawtime; 00031 clock_typ numiclock; 00032 Ticker secondticker; 00033 void secondtick(void); 00034 void updatetime(void); 00035 int main() 00036 { 00037 MX_RTC_Init(); 00038 static struct tm *t3; 00039 buttons.mode(PullUp); 00040 00041 LE = 0; 00042 OE = 1; 00043 00044 t.tm_sec = 0; 00045 t.tm_min = 0; 00046 t.tm_hour = 0; 00047 t.tm_mday = 1; 00048 t.tm_mon = 1-1; 00049 t.tm_year = 2016-1900; 00050 set_time(mktime(&t)); 00051 00052 rawtime = time(NULL); 00053 00054 spi.write(0x00); 00055 spi.write(0x00); 00056 spi.write(0x00); 00057 spi.write(0x00); 00058 00059 LE = 1; 00060 wait_us(10); 00061 LE = 0; 00062 wait_us(10); 00063 00064 OE = 0; 00065 secondticker.attach(&updatetime, 1); 00066 while( 1 ) 00067 { 00068 uint8_t bpressed = buttons; 00069 wait_ms(50); 00070 if((bpressed == buttons) && (bpressed != 0xf)) 00071 { 00072 rawtime = time(NULL); 00073 t3 = localtime(&rawtime); 00074 bpressed = (~bpressed)&0x0f; 00075 switch(bpressed) 00076 { 00077 case 0x01: 00078 t3->tm_hour += 10; 00079 if(t3->tm_hour >= 30) 00080 { 00081 t3->tm_hour -= 30; 00082 } 00083 else if(t3->tm_hour >= 24) 00084 { 00085 t3->tm_hour -= 20; 00086 } 00087 break; 00088 case 0x02: 00089 t3->tm_hour += 1; 00090 if((t3->tm_hour%10) == 0) 00091 { 00092 t3->tm_hour -= 10; 00093 } 00094 else if(t3->tm_hour >= 24) 00095 { 00096 t3->tm_hour -= 4; 00097 } 00098 break; 00099 case 0x04: 00100 t3->tm_min += 10; 00101 if(t3->tm_min >= 60) 00102 { 00103 t3->tm_min -= 60; 00104 } 00105 break; 00106 case 0x08: 00107 t3->tm_min += 1; 00108 if((t3->tm_min%10) == 0) 00109 { 00110 t3->tm_min -= 10; 00111 } 00112 break; 00113 } 00114 set_time(mktime(t3)); 00115 flip = 0; 00116 updatetime(); 00117 flip = 1; 00118 wait_ms(150); 00119 } 00120 } 00121 } 00122 00123 void updatetime(void) 00124 { 00125 static uint8_t flipbit; 00126 static struct tm *t2; 00127 rawtime = time(NULL); 00128 t2 = localtime(&rawtime); 00129 00130 numiclock.second_right = t2->tm_sec%10; 00131 numiclock.second_left = (t2->tm_sec - numiclock.second_right)/10; 00132 numiclock.minute_right = t2->tm_min%10; 00133 numiclock.minute_left = (t2->tm_min - numiclock.minute_right)/10; 00134 numiclock.hour_right = t2->tm_hour%10; 00135 numiclock.hour_left = (t2->tm_hour-numiclock.hour_right)/10; 00136 00137 spi.write(numbers[numiclock.hour_left]); 00138 if(flip) 00139 { 00140 if(flipbit == 1) 00141 { 00142 spi.write((numbers[numiclock.hour_right])+0x80); 00143 flipbit = 0; 00144 } 00145 else 00146 { 00147 spi.write(numbers[numiclock.hour_right]); 00148 flipbit = 1; 00149 } 00150 } 00151 else 00152 { 00153 spi.write(numbers[numiclock.hour_right]); 00154 } 00155 spi.write(numbers[numiclock.minute_left]); 00156 spi.write(numbers[numiclock.minute_right]); 00157 00158 LE = 1; 00159 wait_us(10); 00160 LE = 0; 00161 wait_us(10); 00162 } 00163 00164 /* RTC init function */ 00165 void MX_RTC_Init(void) 00166 { 00167 00168 RTC_TimeTypeDef sTime; 00169 RTC_DateTypeDef sDate; 00170 00171 /**Initialize RTC and set the Time and Date 00172 */ 00173 hrtc.Instance = RTC; 00174 hrtc.Init.HourFormat = RTC_HOURFORMAT_24; 00175 hrtc.Init.AsynchPrediv = 124; 00176 hrtc.Init.SynchPrediv = 1999; 00177 hrtc.Init.OutPut = RTC_OUTPUT_DISABLE; 00178 hrtc.Init.OutPutPolarity = RTC_OUTPUT_POLARITY_HIGH; 00179 hrtc.Init.OutPutType = RTC_OUTPUT_TYPE_OPENDRAIN; 00180 status = HAL_RTC_Init(&hrtc); 00181 00182 sTime.Hours = 0x0; 00183 sTime.Minutes = 0x0; 00184 sTime.Seconds = 0x0; 00185 sTime.DayLightSaving = RTC_DAYLIGHTSAVING_NONE; 00186 sTime.StoreOperation = RTC_STOREOPERATION_RESET; 00187 HAL_RTC_SetTime(&hrtc, &sTime, RTC_FORMAT_BCD); 00188 00189 sDate.WeekDay = RTC_WEEKDAY_MONDAY; 00190 sDate.Month = RTC_MONTH_JANUARY; 00191 sDate.Date = 0x1; 00192 sDate.Year = 0x0; 00193 00194 HAL_RTC_SetDate(&hrtc, &sDate, RTC_FORMAT_BCD); 00195 00196 }
Generated on Wed Jul 13 2022 04:52:58 by
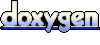