HMC6352 Library
Embed:
(wiki syntax)
Show/hide line numbers
HMC6352.h
00001 // Copyright 2009 Richard Parker 00002 00003 #ifndef MBED_HMC6352_H 00004 #define MBED_HMC6352_H 00005 00006 #include "mbed.h" 00007 00008 class HMC6352 00009 { 00010 public: 00011 // Enum to make the returned mode more readable. 00012 enum Mode { 00013 Standby = 0x0, 00014 Query = 0x1, 00015 Continuous = 0x2, 00016 None = 0x3 00017 }; 00018 00019 // Enum to make the returned output type more readable. 00020 enum Output { 00021 Heading = 0x0, 00022 RawX = 0x1, 00023 RawY = 0x2, 00024 X = 0x3, 00025 Y = 0x4, 00026 Unknown = 0x5 00027 }; 00028 00029 HMC6352(PinName sda, PinName scl); 00030 ~HMC6352(); 00031 00032 // Address. 00033 inline int address() { return _address; } 00034 inline void setAddress(int address) { _address = address; } 00035 00036 // Version. 00037 char getVersion(); 00038 void setVersion(const char version); 00039 00040 // Time delay. 00041 char getTimeDelay(); 00042 void setTimeDelay(const char delay); 00043 00044 // Summing. 00045 char getSumming(); 00046 void setSumming(const char samples); 00047 00048 // Heading. 00049 float getHeading(); 00050 00051 // Control 00052 void reset(); 00053 00054 void wakeUp(); 00055 void goToSleep(); 00056 00057 void startCalibrate(); 00058 void endCalibrate(); 00059 void calibrate(int delay = 20); 00060 00061 // Get the mode section of tyhe operation byte from RAM. 00062 HMC6352::Mode getMode(); 00063 void setMode(HMC6352::Mode mode); 00064 00065 // Save the operation byte in ram to eeprom. 00066 void saveOperation(); 00067 00068 // Get the current output mode from the RAM register. 00069 HMC6352::Output getOutput(); 00070 void setOutput(HMC6352::Output output); 00071 00072 inline float version() const { return 0.1; } 00073 00074 private: 00075 // Clears the contents of the buffer to 0x0 00076 void _clearBuffer(); 00077 00078 // Converts the values in the lower two bytes of the buffer to a heading. 00079 float _bufferToHeading(); 00080 00081 // Read or write to memory. 00082 void _readFromMemory(const char address, const bool eeprom); 00083 void _writeToMemory(const char address, const char data, const bool eeprom); 00084 00085 // Set up a buffer to read and write data. The maximum amount of data 00086 // returned or sent is 3 bytes. 00087 char _buffer[3]; 00088 00089 // Set up the i2c communication channel object. 00090 I2C _i2c; 00091 00092 // To hold the address currently being used. 00093 int _address; 00094 00095 }; 00096 00097 #endif
Generated on Thu Jul 21 2022 08:32:57 by
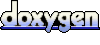