
TestApp
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 //------------------------------------ 00004 // Hyperterminal configuration 00005 // 9600 bauds, 8-bit data, no parity 00006 //------------------------------------ 00007 00008 Serial pc(SERIAL_TX, SERIAL_RX); 00009 00010 DigitalOut myled(PC_13); 00011 00012 int main() 00013 { 00014 __disable_irq(); //Should still be disabled from when you jumped out of the bootloader, but disable again for safety. 00015 uint32_t *old_vectors = (uint32_t *)(0x08010000U); //Or wherever you placed your main application 00016 uint32_t *vectors = (uint32_t*)NVIC_RAM_VECTOR_ADDRESS; 00017 for (int i = 0; i < NVIC_NUM_VECTORS; i++) { 00018 vectors[i] = old_vectors[i]; 00019 } 00020 SCB->VTOR = (uint32_t)NVIC_RAM_VECTOR_ADDRESS; 00021 __enable_irq(); //Re-enable IRQ. In theory your vector table should now be copied and interrupt *should* work. 00022 00023 00024 pc.baud(115200); 00025 int i = 1; 00026 pc.printf("Hello World !\n"); 00027 while(1) { 00028 wait(1); 00029 pc.printf("This program runs since %d seconds.\n", i++); 00030 myled = !myled; 00031 } 00032 }
Generated on Tue Jul 12 2022 20:57:50 by
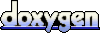