why do I need to publish this
Fork of NeoStrip by
Embed:
(wiki syntax)
Show/hide line numbers
NeoStrip.h
00001 /** 00002 * NeoStrip.h 00003 * 00004 * Allen Wild 00005 * March 2014 00006 * 00007 * Library for the control of Adafruit NeoPixel addressable RGB LEDs. 00008 */ 00009 00010 #include "mbed.h" 00011 #include <stdint.h> 00012 00013 00014 #ifndef NEOSTRIP_H 00015 #define NEOSTRIP_H 00016 00017 #ifndef TARGET_LPC1768 00018 #error NeoStrip only supports the NXP LPC1768! 00019 #endif 00020 00021 // NeoColor struct definition to hold 24 bit 00022 // color data for each pixel, in GRB order 00023 typedef struct _NeoColor 00024 { 00025 uint8_t green; 00026 uint8_t red; 00027 uint8_t blue; 00028 } NeoColor; 00029 00030 /** 00031 * NeoStrip objects manage the buffering and assigning of 00032 * addressable NeoPixels 00033 */ 00034 class NeoStrip 00035 { 00036 public: 00037 00038 /** 00039 * Create a NeoStrip object 00040 * 00041 * @param pin The mbed data pin name 00042 * @param N The number of pixels in the strip 00043 */ 00044 NeoStrip(PinName pin, int N); 00045 00046 /** 00047 * Set an overall brightness scale for the entire strip. 00048 * When colors are set using setPixel(), they are scaled 00049 * according to this brightness. 00050 * 00051 * Because NeoPixels are very bright and draw a lot of current, 00052 * the brightness is set to 0.5 by default. 00053 * 00054 * @param bright The brightness scale between 0 and 1.0 00055 */ 00056 void setBrightness(float bright); 00057 00058 /** 00059 * Set a single pixel to the specified color. 00060 * 00061 * This method (and all other setPixel methods) uses modulo-N arithmetic 00062 * when addressing pixels. If p >= N, the pixel address will wrap around. 00063 * 00064 * @param p The pixel number (starting at 0) to set 00065 * @param color A 24-bit color packed into a single int, 00066 * using standard hex color codes (e.g. 0xFF0000 is red) 00067 */ 00068 void setPixel(int p, int color); 00069 00070 /** 00071 * Set a single pixel to the specified color, with red, green, and blue 00072 * values in separate arguments. 00073 */ 00074 void setPixel(int p, uint8_t red, uint8_t green, uint8_t blue); 00075 00076 /** 00077 * Multiply a pixel value by mask so that you can turn colored pixels on/off 00078 * for displaying text 00079 */ 00080 00081 00082 /** 00083 * Set n pixels starting at pixel p. 00084 * 00085 * @param p The first pixel in the strip to set. 00086 * @param n The number of pixels to set. 00087 * @param colors An array of length n containing the 24-bit colors for each pixel 00088 */ 00089 void setPixels(int p, int n, const int *colors); 00090 00091 /** 00092 * Reset all pixels in the strip to be of (0x000000) 00093 */ 00094 void clear(); 00095 00096 /** 00097 * Write the colors out to the strip; this method must be called 00098 * to see any hardware effect. 00099 * 00100 * This function disables interrupts while the strip data is being sent, 00101 * each pixel takes approximately 30us to send, plus a 50us reset pulse 00102 * at the end. 00103 */ 00104 void write(); 00105 00106 00107 00108 00109 protected: 00110 NeoColor *strip; // pixel data buffer modified by setPixel() and used by neo_out() 00111 int N; // the number of pixels in the strip 00112 int Nbytes; // the number of bytes of pixel data (always N*3) 00113 float bright; // the master strip brightness 00114 gpio_t gpio; // gpio struct for initialization and getting register addresses 00115 }; 00116 00117 #endif 00118 00119
Generated on Fri Aug 5 2022 08:06:06 by
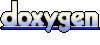