Unit Testing framework based on http://cpputest.github.io/
Embed:
(wiki syntax)
Show/hide line numbers
Utest.h
00001 /* 00002 * Copyright (c) 2007, Michael Feathers, James Grenning and Bas Vodde 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without 00006 * modification, are permitted provided that the following conditions are met: 00007 * * Redistributions of source code must retain the above copyright 00008 * notice, this list of conditions and the following disclaimer. 00009 * * Redistributions in binary form must reproduce the above copyright 00010 * notice, this list of conditions and the following disclaimer in the 00011 * documentation and/or other materials provided with the distribution. 00012 * * Neither the name of the <organization> nor the 00013 * names of its contributors may be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE EARLIER MENTIONED AUTHORS ``AS IS'' AND ANY 00017 * EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00018 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00019 * DISCLAIMED. IN NO EVENT SHALL <copyright holder> BE LIABLE FOR ANY 00020 * DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00021 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00022 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00023 * ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00024 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00025 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00026 */ 00027 00028 // This file contains the Test class along with the macros which make effective 00029 // in the harness. 00030 00031 #ifndef D_UTest_h 00032 #define D_UTest_h 00033 00034 #include "SimpleString.h" 00035 00036 class TestResult; 00037 class TestPlugin; 00038 class TestFailure; 00039 class TestFilter; 00040 class TestTerminator; 00041 00042 extern bool doubles_equal(double d1, double d2, double threshold); 00043 00044 //////////////////// Utest 00045 00046 class UtestShell; 00047 00048 class Utest 00049 { 00050 public: 00051 Utest(); 00052 virtual ~Utest(); 00053 virtual void run(); 00054 00055 virtual void setup(); 00056 virtual void teardown(); 00057 virtual void testBody(); 00058 }; 00059 00060 //////////////////// TestTerminator 00061 00062 class TestTerminator 00063 { 00064 public: 00065 virtual void exitCurrentTest() const=0; 00066 virtual ~TestTerminator(); 00067 }; 00068 00069 class NormalTestTerminator : public TestTerminator 00070 { 00071 public: 00072 virtual void exitCurrentTest() const _override; 00073 virtual ~NormalTestTerminator(); 00074 }; 00075 00076 class TestTerminatorWithoutExceptions : public TestTerminator 00077 { 00078 public: 00079 virtual void exitCurrentTest() const _override; 00080 virtual ~TestTerminatorWithoutExceptions(); 00081 }; 00082 00083 //////////////////// UtestShell 00084 00085 class UtestShell 00086 { 00087 public: 00088 static UtestShell *getCurrent(); 00089 00090 public: 00091 UtestShell(const char* groupName, const char* testName, const char* fileName, int lineNumber); 00092 virtual ~UtestShell(); 00093 00094 virtual UtestShell* addTest(UtestShell* test); 00095 virtual UtestShell *getNext() const; 00096 virtual bool isNull() const; 00097 virtual int countTests(); 00098 00099 bool shouldRun(const TestFilter& groupFilter, const TestFilter& nameFilter) const; 00100 const SimpleString getName() const; 00101 const SimpleString getGroup() const; 00102 virtual SimpleString getFormattedName() const; 00103 const SimpleString getFile() const; 00104 int getLineNumber() const; 00105 virtual const char *getProgressIndicator() const; 00106 virtual bool hasFailed() const; 00107 00108 virtual void assertTrue(bool condition, const char *checkString, const char *conditionString, const char *fileName, int lineNumber, const TestTerminator& testTerminator = NormalTestTerminator()); 00109 virtual void assertTrueText(bool condition, const char *checkString, const char *conditionString, const char* text, const char *fileName, int lineNumber, const TestTerminator& testTerminator = NormalTestTerminator()); 00110 virtual void assertCstrEqual(const char *expected, const char *actual, const char *fileName, int lineNumber, const TestTerminator& testTerminator = NormalTestTerminator()); 00111 virtual void assertCstrNoCaseEqual(const char *expected, const char *actual, const char *fileName, int lineNumber); 00112 virtual void assertCstrContains(const char *expected, const char *actual, const char *fileName, int lineNumber); 00113 virtual void assertCstrNoCaseContains(const char *expected, const char *actual, const char *fileName, int lineNumber); 00114 virtual void assertLongsEqual(long expected, long actual, const char *fileName, int lineNumber, const TestTerminator& testTerminator = NormalTestTerminator()); 00115 virtual void assertUnsignedLongsEqual(unsigned long expected, unsigned long actual, const char *fileName, int lineNumber, const TestTerminator& testTerminator = NormalTestTerminator()); 00116 virtual void assertPointersEqual(const void *expected, const void *actual, const char *fileName, int lineNumber); 00117 virtual void assertDoublesEqual(double expected, double actual, double threshold, const char *fileName, int lineNumber, const TestTerminator& testTerminator = NormalTestTerminator()); 00118 virtual void assertEquals(bool failed, const char* expected, const char* actual, const char* file, int line, const TestTerminator& testTerminator = NormalTestTerminator()); 00119 virtual void fail(const char *text, const char *fileName, int lineNumber, const TestTerminator& testTerminator = NormalTestTerminator()); 00120 00121 virtual void print(const char *text, const char *fileName, int lineNumber); 00122 virtual void print(const SimpleString & text, const char *fileName, int lineNumber); 00123 00124 void setFileName(const char *fileName); 00125 void setLineNumber(int lineNumber); 00126 void setGroupName(const char *groupName); 00127 void setTestName(const char *testName); 00128 00129 static void crash(); 00130 static void setCrashMethod(void (*crashme)()); 00131 static void resetCrashMethod(); 00132 00133 virtual bool isRunInSeperateProcess() const; 00134 virtual void setRunInSeperateProcess(); 00135 00136 virtual Utest* createTest(); 00137 virtual void destroyTest(Utest* test); 00138 00139 virtual void runOneTest(TestPlugin* plugin, TestResult& result); 00140 virtual void runOneTestInCurrentProcess(TestPlugin *plugin, TestResult & result); 00141 00142 virtual void failWith(const TestFailure& failure); 00143 virtual void failWith(const TestFailure& failure, const TestTerminator& terminator); 00144 00145 protected: 00146 UtestShell(); 00147 UtestShell(const char *groupName, const char *testName, const char *fileName, int lineNumber, UtestShell *nextTest); 00148 00149 virtual SimpleString getMacroName() const; 00150 TestResult *getTestResult(); 00151 private: 00152 const char *group_; 00153 const char *name_; 00154 const char *file_; 00155 int lineNumber_; 00156 UtestShell *next_; 00157 bool isRunAsSeperateProcess_; 00158 bool hasFailed_; 00159 00160 void setTestResult(TestResult* result); 00161 void setCurrentTest(UtestShell* test); 00162 00163 static UtestShell* currentTest_; 00164 static TestResult* testResult_; 00165 00166 }; 00167 00168 //////////////////// NullTest 00169 00170 class NullTestShell: public UtestShell 00171 { 00172 public: 00173 explicit NullTestShell(); 00174 explicit NullTestShell(const char* fileName, int lineNumber); 00175 virtual ~NullTestShell(); 00176 00177 void testBody(); 00178 00179 static NullTestShell& instance(); 00180 00181 virtual int countTests() _override; 00182 virtual UtestShell*getNext() const _override; 00183 virtual bool isNull() const _override; 00184 private: 00185 00186 NullTestShell(const NullTestShell&); 00187 NullTestShell& operator=(const NullTestShell&); 00188 00189 }; 00190 00191 //////////////////// ExecFunctionTest 00192 00193 class ExecFunctionTestShell; 00194 00195 class ExecFunctionTest : public Utest 00196 { 00197 public: 00198 ExecFunctionTest(ExecFunctionTestShell* shell); 00199 void testBody(); 00200 virtual void setup() _override; 00201 virtual void teardown() _override; 00202 private: 00203 ExecFunctionTestShell* shell_; 00204 }; 00205 00206 //////////////////// ExecFunctionTestShell 00207 00208 class ExecFunctionTestShell: public UtestShell 00209 { 00210 public: 00211 void (*setup_)(); 00212 void (*teardown_)(); 00213 void (*testFunction_)(); 00214 00215 ExecFunctionTestShell(void(*set)() = 0, void(*tear)() = 0) : 00216 UtestShell("Generic", "Generic", "Generic", 1), setup_(set), teardown_( 00217 tear), testFunction_(0) 00218 { 00219 } 00220 Utest* createTest() { return new ExecFunctionTest(this); } 00221 virtual ~ExecFunctionTestShell(); 00222 }; 00223 00224 //////////////////// CppUTestFailedException 00225 00226 class CppUTestFailedException 00227 { 00228 public: 00229 int dummy_; 00230 }; 00231 00232 //////////////////// IgnoredTest 00233 00234 class IgnoredUtestShell : public UtestShell 00235 { 00236 public: 00237 IgnoredUtestShell(); 00238 virtual ~IgnoredUtestShell(); 00239 explicit IgnoredUtestShell(const char* groupName, const char* testName, 00240 const char* fileName, int lineNumber); 00241 virtual const char* getProgressIndicator() const; 00242 protected: virtual SimpleString getMacroName() const _override; 00243 virtual void runOneTest(TestPlugin* plugin, TestResult& result) _override; 00244 00245 private: 00246 00247 IgnoredUtestShell(const IgnoredUtestShell&); 00248 IgnoredUtestShell& operator=(const IgnoredUtestShell&); 00249 00250 }; 00251 00252 //////////////////// TestInstaller 00253 00254 class TestInstaller 00255 { 00256 public: 00257 explicit TestInstaller(UtestShell& shell, const char* groupName, const char* testName, 00258 const char* fileName, int lineNumber); 00259 virtual ~TestInstaller(); 00260 00261 void unDo(); 00262 00263 private: 00264 00265 TestInstaller(const TestInstaller&); 00266 TestInstaller& operator=(const TestInstaller&); 00267 00268 }; 00269 00270 #endif
Generated on Tue Jul 12 2022 21:43:38 by
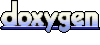