Unit Testing framework based on http://cpputest.github.io/
Embed:
(wiki syntax)
Show/hide line numbers
UtestMacros.h
00001 /* 00002 * Copyright (c) 2007, Michael Feathers, James Grenning and Bas Vodde 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without 00006 * modification, are permitted provided that the following conditions are met: 00007 * * Redistributions of source code must retain the above copyright 00008 * notice, this list of conditions and the following disclaimer. 00009 * * Redistributions in binary form must reproduce the above copyright 00010 * notice, this list of conditions and the following disclaimer in the 00011 * documentation and/or other materials provided with the distribution. 00012 * * Neither the name of the <organization> nor the 00013 * names of its contributors may be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE EARLIER MENTIONED AUTHORS ``AS IS'' AND ANY 00017 * EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00018 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00019 * DISCLAIMED. IN NO EVENT SHALL <copyright holder> BE LIABLE FOR ANY 00020 * DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00021 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00022 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00023 * ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00024 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00025 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00026 */ 00027 00028 #ifndef D_UTestMacros_h 00029 #define D_UTestMacros_h 00030 00031 /*! \brief Define a group of tests 00032 * 00033 * All tests in a TEST_GROUP share the same setup() 00034 * and teardown(). setup() is run before the opening 00035 * curly brace of each TEST and teardown() is 00036 * called after the closing curly brace of TEST. 00037 * 00038 */ 00039 00040 00041 #define TEST_GROUP_BASE(testGroup, baseclass) \ 00042 extern int externTestGroup##testGroup; \ 00043 int externTestGroup##testGroup = 0; \ 00044 struct TEST_GROUP_##CppUTestGroup##testGroup : public baseclass 00045 00046 #define TEST_BASE(testBaseClass) \ 00047 struct testBaseClass : public Utest 00048 00049 #define TEST_GROUP(testGroup) \ 00050 TEST_GROUP_BASE(testGroup, Utest) 00051 00052 #define TEST_SETUP() \ 00053 virtual void setup() 00054 00055 #define TEST_TEARDOWN() \ 00056 virtual void teardown() 00057 00058 #define TEST(testGroup, testName) \ 00059 /* External declarations for strict compilers */ \ 00060 class TEST_##testGroup##_##testName##_TestShell; \ 00061 extern TEST_##testGroup##_##testName##_TestShell TEST_##testGroup##_##testName##_TestShell_instance; \ 00062 \ 00063 class TEST_##testGroup##_##testName##_Test : public TEST_GROUP_##CppUTestGroup##testGroup \ 00064 { public: TEST_##testGroup##_##testName##_Test () : TEST_GROUP_##CppUTestGroup##testGroup () {} \ 00065 void testBody(); }; \ 00066 class TEST_##testGroup##_##testName##_TestShell : public UtestShell { \ 00067 virtual Utest* createTest() _override { return new TEST_##testGroup##_##testName##_Test; } \ 00068 } TEST_##testGroup##_##testName##_TestShell_instance; \ 00069 static TestInstaller TEST_##testGroup##_##testName##_Installer(TEST_##testGroup##_##testName##_TestShell_instance, #testGroup, #testName, __FILE__,__LINE__); \ 00070 void TEST_##testGroup##_##testName##_Test::testBody() 00071 00072 #define IGNORE_TEST(testGroup, testName)\ 00073 /* External declarations for strict compilers */ \ 00074 class IGNORE##testGroup##_##testName##_TestShell; \ 00075 extern IGNORE##testGroup##_##testName##_TestShell IGNORE##testGroup##_##testName##_TestShell_instance; \ 00076 \ 00077 class IGNORE##testGroup##_##testName##_Test : public TEST_GROUP_##CppUTestGroup##testGroup \ 00078 { public: IGNORE##testGroup##_##testName##_Test () : TEST_GROUP_##CppUTestGroup##testGroup () {} \ 00079 public: void testBodyThatNeverRuns (); }; \ 00080 class IGNORE##testGroup##_##testName##_TestShell : public IgnoredUtestShell { \ 00081 virtual Utest* createTest() _override { return new IGNORE##testGroup##_##testName##_Test; } \ 00082 } IGNORE##testGroup##_##testName##_TestShell_instance; \ 00083 static TestInstaller TEST_##testGroup##testName##_Installer(IGNORE##testGroup##_##testName##_TestShell_instance, #testGroup, #testName, __FILE__,__LINE__); \ 00084 void IGNORE##testGroup##_##testName##_Test::testBodyThatNeverRuns () 00085 00086 #define IMPORT_TEST_GROUP(testGroup) \ 00087 extern int externTestGroup##testGroup;\ 00088 extern int* p##testGroup; \ 00089 int* p##testGroup = &externTestGroup##testGroup 00090 00091 // Different checking macros 00092 00093 #define CHECK(condition)\ 00094 CHECK_LOCATION_TRUE(condition, "CHECK", #condition, __FILE__, __LINE__) 00095 00096 #define CHECK_TEXT(condition, text) \ 00097 CHECK_LOCATION_TEXT(condition, "CHECK", #condition, text, __FILE__, __LINE__) 00098 00099 #define CHECK_TRUE(condition)\ 00100 CHECK_LOCATION_TRUE(condition, "CHECK_TRUE", #condition, __FILE__, __LINE__) 00101 00102 #define CHECK_FALSE(condition)\ 00103 CHECK_LOCATION_FALSE(condition, "CHECK_FALSE", #condition, __FILE__, __LINE__) 00104 00105 #define CHECK_LOCATION_TEXT(condition, checkString, conditionString, text, file, line) \ 00106 { UtestShell::getCurrent()->assertTrueText((condition) != 0, checkString, conditionString, text, file, line); } 00107 00108 #define CHECK_LOCATION_TRUE(condition, checkString, conditionString, file, line)\ 00109 { UtestShell::getCurrent()->assertTrue((condition) != 0, checkString, conditionString, file, line); } 00110 00111 #define CHECK_LOCATION_FALSE(condition, checkString, conditionString, file, line)\ 00112 { UtestShell::getCurrent()->assertTrue((condition) == 0, checkString, conditionString, file, line); } 00113 00114 //This check needs the operator!=(), and a StringFrom(YourType) function 00115 #define CHECK_EQUAL(expected,actual)\ 00116 CHECK_EQUAL_LOCATION(expected, actual, __FILE__, __LINE__) 00117 00118 #define CHECK_EQUAL_LOCATION(expected,actual, file, line)\ 00119 { if ((expected) != (actual)) { \ 00120 if ((actual) != (actual)) \ 00121 UtestShell::getCurrent()->print("WARNING:\n\tThe \"Actual Parameter\" parameter is evaluated multiple times resulting in different values.\n\tThus the value in the error message is probably incorrect.", file, line); \ 00122 if ((expected) != (expected)) \ 00123 UtestShell::getCurrent()->print("WARNING:\n\tThe \"Expected Parameter\" parameter is evaluated multiple times resulting in different values.\n\tThus the value in the error message is probably incorrect.", file, line); \ 00124 UtestShell::getCurrent()->assertEquals(true, StringFrom(expected).asCharString(), StringFrom(actual).asCharString(), file, line); \ 00125 } \ 00126 else \ 00127 { \ 00128 UtestShell::getCurrent()->assertLongsEqual((long)0, (long)0, file, line); \ 00129 } } 00130 00131 //This check checks for char* string equality using strcmp. 00132 //This makes up for the fact that CHECK_EQUAL only compares the pointers to char*'s 00133 #define STRCMP_EQUAL(expected,actual)\ 00134 STRCMP_EQUAL_LOCATION(expected, actual, __FILE__, __LINE__) 00135 00136 #define STRCMP_EQUAL_LOCATION(expected,actual, file, line)\ 00137 { UtestShell::getCurrent()->assertCstrEqual(expected, actual, file, line); } 00138 00139 #define STRCMP_NOCASE_EQUAL(expected,actual)\ 00140 STRCMP_NOCASE_EQUAL_LOCATION(expected, actual, __FILE__, __LINE__) 00141 00142 #define STRCMP_NOCASE_EQUAL_LOCATION(expected,actual, file, line)\ 00143 { UtestShell::getCurrent()->assertCstrNoCaseEqual(expected, actual, file, line); } 00144 00145 #define STRCMP_CONTAINS(expected,actual)\ 00146 STRCMP_CONTAINS_LOCATION(expected, actual, __FILE__, __LINE__) 00147 00148 #define STRCMP_CONTAINS_LOCATION(expected,actual, file, line)\ 00149 { UtestShell::getCurrent()->assertCstrContains(expected, actual, file, line); } 00150 00151 #define STRCMP_NOCASE_CONTAINS(expected,actual)\ 00152 STRCMP_NOCASE_CONTAINS_LOCATION(expected, actual, __FILE__, __LINE__) 00153 00154 #define STRCMP_NOCASE_CONTAINS_LOCATION(expected,actual, file, line)\ 00155 { UtestShell::getCurrent()->assertCstrNoCaseContains(expected, actual, file, line); } 00156 00157 //Check two long integers for equality 00158 #define LONGS_EQUAL(expected,actual)\ 00159 LONGS_EQUAL_LOCATION(expected,actual,__FILE__, __LINE__) 00160 00161 #define UNSIGNED_LONGS_EQUAL(expected,actual)\ 00162 UNSIGNED_LONGS_EQUAL_LOCATION(expected,actual,__FILE__, __LINE__) 00163 00164 #define LONGS_EQUAL_LOCATION(expected,actual,file,line)\ 00165 { UtestShell::getCurrent()->assertLongsEqual((long)expected, (long)actual, file, line); } 00166 00167 #define UNSIGNED_LONGS_EQUAL_LOCATION(expected,actual,file,line)\ 00168 { UtestShell::getCurrent()->assertUnsignedLongsEqual((unsigned long)expected, (unsigned long)actual, file, line); } 00169 00170 #define BYTES_EQUAL(expected, actual)\ 00171 LONGS_EQUAL((expected) & 0xff,(actual) & 0xff) 00172 00173 #define POINTERS_EQUAL(expected, actual)\ 00174 POINTERS_EQUAL_LOCATION((expected),(actual), __FILE__, __LINE__) 00175 00176 #define POINTERS_EQUAL_LOCATION(expected,actual,file,line)\ 00177 { UtestShell::getCurrent()->assertPointersEqual((void *)expected, (void *)actual, file, line); } 00178 00179 //Check two doubles for equality within a tolerance threshold 00180 #define DOUBLES_EQUAL(expected,actual,threshold)\ 00181 DOUBLES_EQUAL_LOCATION(expected,actual,threshold,__FILE__,__LINE__) 00182 00183 #define DOUBLES_EQUAL_LOCATION(expected,actual,threshold,file,line)\ 00184 { UtestShell::getCurrent()->assertDoublesEqual(expected, actual, threshold, file, line); } 00185 00186 //Fail if you get to this macro 00187 //The macro FAIL may already be taken, so allow FAIL_TEST too 00188 #ifndef FAIL 00189 #define FAIL(text)\ 00190 FAIL_LOCATION(text, __FILE__,__LINE__) 00191 00192 #define FAIL_LOCATION(text, file, line)\ 00193 { UtestShell::getCurrent()->fail(text, file, line); } 00194 #endif 00195 00196 #define FAIL_TEST(text)\ 00197 FAIL_TEST_LOCATION(text, __FILE__,__LINE__) 00198 00199 #define FAIL_TEST_LOCATION(text, file,line)\ 00200 { UtestShell::getCurrent()->fail(text, file, line); } 00201 00202 #define UT_PRINT_LOCATION(text, file, line) \ 00203 { UtestShell::getCurrent()->print(text, file, line); } 00204 00205 #define UT_PRINT(text) \ 00206 UT_PRINT_LOCATION(text, __FILE__, __LINE__) 00207 00208 #if CPPUTEST_USE_STD_CPP_LIB 00209 #define CHECK_THROWS(expected, expression) \ 00210 { \ 00211 SimpleString msg("expected to throw "#expected "\nbut threw nothing"); \ 00212 bool caught_expected = false; \ 00213 try { \ 00214 (expression); \ 00215 } catch(const expected &) { \ 00216 caught_expected = true; \ 00217 } catch(...) { \ 00218 msg = "expected to throw " #expected "\nbut threw a different type"; \ 00219 } \ 00220 if (!caught_expected) { \ 00221 UtestShell::getCurrent()->fail(msg.asCharString(), __FILE__, __LINE__); \ 00222 } \ 00223 } 00224 #endif /* CPPUTEST_USE_STD_CPP_LIB */ 00225 00226 #define UT_CRASH() { UtestShell::crash(); } 00227 #define RUN_ALL_TESTS(ac, av) CommandLineTestRunner::RunAllTests(ac, av) 00228 00229 #endif /*D_UTestMacros_h*/
Generated on Tue Jul 12 2022 21:43:38 by
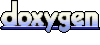