Unit Testing framework based on http://cpputest.github.io/
Embed:
(wiki syntax)
Show/hide line numbers
TestOutput.cpp
00001 /* 00002 * Copyright (c) 2007, Michael Feathers, James Grenning and Bas Vodde 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without 00006 * modification, are permitted provided that the following conditions are met: 00007 * * Redistributions of source code must retain the above copyright 00008 * notice, this list of conditions and the following disclaimer. 00009 * * Redistributions in binary form must reproduce the above copyright 00010 * notice, this list of conditions and the following disclaimer in the 00011 * documentation and/or other materials provided with the distribution. 00012 * * Neither the name of the <organization> nor the 00013 * names of its contributors may be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE EARLIER MENTIONED AUTHORS ``AS IS'' AND ANY 00017 * EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00018 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00019 * DISCLAIMED. IN NO EVENT SHALL <copyright holder> BE LIABLE FOR ANY 00020 * DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00021 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00022 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00023 * ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00024 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00025 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00026 */ 00027 00028 #include "CppUTest/TestHarness.h" 00029 #include "CppUTest/TestOutput.h" 00030 #include "CppUTest/PlatformSpecificFunctions.h" 00031 00032 TestOutput::WorkingEnvironment TestOutput::workingEnvironment_ = TestOutput::detectEnvironment; 00033 00034 void TestOutput::setWorkingEnvironment(TestOutput::WorkingEnvironment workEnvironment) 00035 { 00036 workingEnvironment_ = workEnvironment; 00037 } 00038 00039 TestOutput::WorkingEnvironment TestOutput::getWorkingEnvironment() 00040 { 00041 if (workingEnvironment_ == TestOutput::detectEnvironment) 00042 return PlatformSpecificGetWorkingEnvironment(); 00043 return workingEnvironment_; 00044 } 00045 00046 00047 TestOutput::TestOutput() : 00048 dotCount_(0), verbose_(false), progressIndication_(".") 00049 { 00050 } 00051 00052 TestOutput::~TestOutput() 00053 { 00054 } 00055 00056 void TestOutput::verbose() 00057 { 00058 verbose_ = true; 00059 } 00060 00061 void TestOutput::print(const char* str) 00062 { 00063 printBuffer(str); 00064 } 00065 00066 void TestOutput::print(long n) 00067 { 00068 print(StringFrom(n).asCharString()); 00069 } 00070 00071 void TestOutput::printDouble(double d) 00072 { 00073 print(StringFrom(d).asCharString()); 00074 } 00075 00076 void TestOutput::printHex(long n) 00077 { 00078 print(HexStringFrom(n).asCharString()); 00079 } 00080 00081 TestOutput& operator<<(TestOutput& p, const char* s) 00082 { 00083 p.print(s); 00084 return p; 00085 } 00086 00087 TestOutput& operator<<(TestOutput& p, long int i) 00088 { 00089 p.print(i); 00090 return p; 00091 } 00092 00093 void TestOutput::printCurrentTestStarted(const UtestShell& test) 00094 { 00095 if (verbose_) print(test.getFormattedName().asCharString()); 00096 } 00097 00098 void TestOutput::printCurrentTestEnded(const TestResult& res) 00099 { 00100 if (verbose_) { 00101 print(" - "); 00102 print(res.getCurrentTestTotalExecutionTime()); 00103 print(" ms\n"); 00104 } 00105 else { 00106 printProgressIndicator(); 00107 } 00108 } 00109 00110 void TestOutput::printProgressIndicator() 00111 { 00112 print(progressIndication_); 00113 if (++dotCount_ % 50 == 0) print("\n"); 00114 } 00115 00116 void TestOutput::setProgressIndicator(const char* indicator) 00117 { 00118 progressIndication_ = indicator; 00119 } 00120 00121 void TestOutput::printTestsStarted() 00122 { 00123 } 00124 00125 void TestOutput::printCurrentGroupStarted(const UtestShell& /*test*/) 00126 { 00127 } 00128 00129 void TestOutput::printCurrentGroupEnded(const TestResult& /*res*/) 00130 { 00131 } 00132 00133 void TestOutput::flush() 00134 { 00135 } 00136 00137 void TestOutput::printTestsEnded(const TestResult& result) 00138 { 00139 if (result.getFailureCount() > 0) { 00140 print("\nErrors ("); 00141 print(result.getFailureCount()); 00142 print(" failures, "); 00143 } 00144 else { 00145 print("\nOK ("); 00146 } 00147 print(result.getTestCount()); 00148 print(" tests, "); 00149 print(result.getRunCount()); 00150 print(" ran, "); 00151 print(result.getCheckCount()); 00152 print(" checks, "); 00153 print(result.getIgnoredCount()); 00154 print(" ignored, "); 00155 print(result.getFilteredOutCount()); 00156 print(" filtered out, "); 00157 print(result.getTotalExecutionTime()); 00158 print(" ms)\n\n"); 00159 } 00160 00161 void TestOutput::printTestRun(int number, int total) 00162 { 00163 if (total > 1) { 00164 print("Test run "); 00165 print(number); 00166 print(" of "); 00167 print(total); 00168 print("\n"); 00169 } 00170 } 00171 00172 void TestOutput::print(const TestFailure& failure) 00173 { 00174 if (failure.isOutsideTestFile() || failure.isInHelperFunction()) 00175 printFileAndLineForTestAndFailure(failure); 00176 else 00177 printFileAndLineForFailure(failure); 00178 00179 printFailureMessage(failure.getMessage()); 00180 } 00181 00182 void TestOutput::printFileAndLineForTestAndFailure(const TestFailure& failure) 00183 { 00184 printErrorInFileOnLineFormattedForWorkingEnvironment(failure.getTestFileName(), failure.getTestLineNumber()); 00185 printFailureInTest(failure.getTestName()); 00186 printErrorInFileOnLineFormattedForWorkingEnvironment(failure.getFileName(), failure.getFailureLineNumber()); 00187 } 00188 00189 void TestOutput::printFileAndLineForFailure(const TestFailure& failure) 00190 { 00191 printErrorInFileOnLineFormattedForWorkingEnvironment(failure.getFileName(), failure.getFailureLineNumber()); 00192 printFailureInTest(failure.getTestName()); 00193 } 00194 00195 void TestOutput::printFailureInTest(SimpleString testName) 00196 { 00197 print(" Failure in "); 00198 print(testName.asCharString()); 00199 } 00200 00201 void TestOutput::printFailureMessage(SimpleString reason) 00202 { 00203 print("\n"); 00204 print("\t"); 00205 print(reason.asCharString()); 00206 print("\n\n"); 00207 } 00208 00209 void TestOutput::printErrorInFileOnLineFormattedForWorkingEnvironment(SimpleString file, int lineNumber) 00210 { 00211 if (TestOutput::getWorkingEnvironment() == TestOutput::vistualStudio) 00212 printVistualStudioErrorInFileOnLine(file, lineNumber); 00213 else 00214 printEclipseErrorInFileOnLine(file, lineNumber); 00215 } 00216 00217 void TestOutput::printEclipseErrorInFileOnLine(SimpleString file, int lineNumber) 00218 { 00219 print("\n"); 00220 print(file.asCharString()); 00221 print(":"); 00222 print(lineNumber); 00223 print(":"); 00224 print(" error:"); 00225 } 00226 00227 void TestOutput::printVistualStudioErrorInFileOnLine(SimpleString file, int lineNumber) 00228 { 00229 print("\n"); 00230 print(file.asCharString()); 00231 print("("); 00232 print(lineNumber); 00233 print("):"); 00234 print(" error:"); 00235 } 00236 00237 void ConsoleTestOutput::printBuffer(const char* s) 00238 { 00239 while (*s) { 00240 PlatformSpecificPutchar(*s); 00241 s++; 00242 } 00243 flush(); 00244 } 00245 00246 void ConsoleTestOutput::flush() 00247 { 00248 PlatformSpecificFlush(); 00249 } 00250 00251 StringBufferTestOutput::~StringBufferTestOutput() 00252 { 00253 }
Generated on Tue Jul 12 2022 21:43:37 by
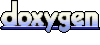