Unit Testing framework based on http://cpputest.github.io/
Embed:
(wiki syntax)
Show/hide line numbers
TestMemoryAllocator.cpp
00001 /* 00002 * Copyright (c) 2007, Michael Feathers, James Grenning and Bas Vodde 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without 00006 * modification, are permitted provided that the following conditions are met: 00007 * * Redistributions of source code must retain the above copyright 00008 * notice, this list of conditions and the following disclaimer. 00009 * * Redistributions in binary form must reproduce the above copyright 00010 * notice, this list of conditions and the following disclaimer in the 00011 * documentation and/or other materials provided with the distribution. 00012 * * Neither the name of the <organization> nor the 00013 * names of its contributors may be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE EARLIER MENTIONED AUTHORS ``AS IS'' AND ANY 00017 * EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00018 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00019 * DISCLAIMED. IN NO EVENT SHALL <copyright holder> BE LIABLE FOR ANY 00020 * DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00021 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00022 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00023 * ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00024 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00025 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00026 */ 00027 00028 #include "CppUTest/TestHarness.h" 00029 #include "CppUTest/TestMemoryAllocator.h" 00030 #include "CppUTest/PlatformSpecificFunctions.h" 00031 #include "CppUTest/MemoryLeakDetector.h" 00032 00033 static char* checkedMalloc(size_t size) 00034 { 00035 char* mem = (char*) PlatformSpecificMalloc(size); 00036 if (mem == 0) 00037 FAIL("malloc returned null pointer"); 00038 return mem; 00039 } 00040 00041 static TestMemoryAllocator* currentNewAllocator = 0; 00042 static TestMemoryAllocator* currentNewArrayAllocator = 0; 00043 static TestMemoryAllocator* currentMallocAllocator = 0; 00044 00045 void setCurrentNewAllocator(TestMemoryAllocator* allocator) 00046 { 00047 currentNewAllocator = allocator; 00048 } 00049 00050 TestMemoryAllocator* getCurrentNewAllocator() 00051 { 00052 if (currentNewAllocator == 0) setCurrentNewAllocatorToDefault(); 00053 return currentNewAllocator; 00054 } 00055 00056 void setCurrentNewAllocatorToDefault() 00057 { 00058 currentNewAllocator = defaultNewAllocator(); 00059 } 00060 00061 TestMemoryAllocator* defaultNewAllocator() 00062 { 00063 static TestMemoryAllocator allocator("Standard New Allocator", "new", "delete"); 00064 return &allocator; 00065 } 00066 00067 void setCurrentNewArrayAllocator(TestMemoryAllocator* allocator) 00068 { 00069 currentNewArrayAllocator = allocator; 00070 } 00071 00072 TestMemoryAllocator* getCurrentNewArrayAllocator() 00073 { 00074 if (currentNewArrayAllocator == 0) setCurrentNewArrayAllocatorToDefault(); 00075 return currentNewArrayAllocator; 00076 } 00077 00078 void setCurrentNewArrayAllocatorToDefault() 00079 { 00080 currentNewArrayAllocator = defaultNewArrayAllocator(); 00081 } 00082 00083 TestMemoryAllocator* defaultNewArrayAllocator() 00084 { 00085 static TestMemoryAllocator allocator("Standard New [] Allocator", "new []", "delete []"); 00086 return &allocator; 00087 } 00088 00089 void setCurrentMallocAllocator(TestMemoryAllocator* allocator) 00090 { 00091 currentMallocAllocator = allocator; 00092 } 00093 00094 TestMemoryAllocator* getCurrentMallocAllocator() 00095 { 00096 if (currentMallocAllocator == 0) setCurrentMallocAllocatorToDefault(); 00097 return currentMallocAllocator; 00098 } 00099 00100 void setCurrentMallocAllocatorToDefault() 00101 { 00102 currentMallocAllocator = defaultMallocAllocator(); 00103 } 00104 00105 TestMemoryAllocator* defaultMallocAllocator() 00106 { 00107 static TestMemoryAllocator allocator("Standard Malloc Allocator", "malloc", "free"); 00108 return &allocator; 00109 } 00110 00111 ///////////////////////////////////////////// 00112 00113 TestMemoryAllocator::TestMemoryAllocator(const char* name_str, const char* alloc_name_str, const char* free_name_str) 00114 : name_(name_str), alloc_name_(alloc_name_str), free_name_(free_name_str), hasBeenDestroyed_(false) 00115 { 00116 } 00117 00118 TestMemoryAllocator::~TestMemoryAllocator() 00119 { 00120 hasBeenDestroyed_ = true; 00121 } 00122 00123 bool TestMemoryAllocator::hasBeenDestroyed() 00124 { 00125 return hasBeenDestroyed_; 00126 } 00127 00128 bool TestMemoryAllocator::isOfEqualType(TestMemoryAllocator* allocator) 00129 { 00130 return PlatformSpecificStrCmp(this->name(), allocator->name()) == 0; 00131 } 00132 00133 char* TestMemoryAllocator::allocMemoryLeakNode(size_t size) 00134 { 00135 return alloc_memory(size, "MemoryLeakNode", 1); 00136 } 00137 00138 void TestMemoryAllocator::freeMemoryLeakNode(char* memory) 00139 { 00140 free_memory(memory, "MemoryLeakNode", 1); 00141 } 00142 00143 char* TestMemoryAllocator::alloc_memory(size_t size, const char*, int) 00144 { 00145 return checkedMalloc(size); 00146 } 00147 00148 void TestMemoryAllocator::free_memory(char* memory, const char*, int) 00149 { 00150 PlatformSpecificFree(memory); 00151 } 00152 const char* TestMemoryAllocator::name() 00153 { 00154 return name_; 00155 } 00156 00157 const char* TestMemoryAllocator::alloc_name() 00158 { 00159 return alloc_name_; 00160 } 00161 00162 const char* TestMemoryAllocator::free_name() 00163 { 00164 return free_name_; 00165 } 00166 00167 CrashOnAllocationAllocator::CrashOnAllocationAllocator() : allocationToCrashOn_(0) 00168 { 00169 } 00170 00171 void CrashOnAllocationAllocator::setNumberToCrashOn(unsigned allocationToCrashOn) 00172 { 00173 allocationToCrashOn_ = allocationToCrashOn; 00174 } 00175 00176 char* CrashOnAllocationAllocator::alloc_memory(size_t size, const char* file, int line) 00177 { 00178 if (MemoryLeakWarningPlugin::getGlobalDetector()->getCurrentAllocationNumber() == allocationToCrashOn_) 00179 UT_CRASH(); 00180 00181 return TestMemoryAllocator::alloc_memory(size, file, line); 00182 } 00183 00184 00185 char* NullUnknownAllocator::alloc_memory(size_t /*size*/, const char*, int) 00186 { 00187 return 0; 00188 } 00189 00190 void NullUnknownAllocator::free_memory(char* /*memory*/, const char*, int) 00191 { 00192 } 00193 00194 NullUnknownAllocator::NullUnknownAllocator() 00195 : TestMemoryAllocator("Null Allocator", "unknown", "unknown") 00196 { 00197 } 00198 00199 00200 TestMemoryAllocator* NullUnknownAllocator::defaultAllocator() 00201 { 00202 static NullUnknownAllocator allocator; 00203 return &allocator; 00204 }
Generated on Tue Jul 12 2022 21:43:37 by
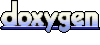