Unit Testing framework based on http://cpputest.github.io/
Embed:
(wiki syntax)
Show/hide line numbers
PlatformSpecificFunctions_c.h
00001 /* 00002 * Copyright (c) 2007, Michael Feathers, James Grenning and Bas Vodde 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without 00006 * modification, are permitted provided that the following conditions are met: 00007 * * Redistributions of source code must retain the above copyright 00008 * notice, this list of conditions and the following disclaimer. 00009 * * Redistributions in binary form must reproduce the above copyright 00010 * notice, this list of conditions and the following disclaimer in the 00011 * documentation and/or other materials provided with the distribution. 00012 * * Neither the name of the <organization> nor the 00013 * names of its contributors may be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE EARLIER MENTIONED AUTHORS ``AS IS'' AND ANY 00017 * EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00018 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00019 * DISCLAIMED. IN NO EVENT SHALL <copyright holder> BE LIABLE FOR ANY 00020 * DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00021 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00022 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00023 * ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00024 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00025 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00026 */ 00027 00028 /****************************************************************************** 00029 * 00030 * PlatformSpecificFunctions_c.H 00031 * 00032 * Provides an interface for when working with pure C 00033 * 00034 *******************************************************************************/ 00035 00036 00037 #ifndef PLATFORMSPECIFICFUNCTIONS_C_H_ 00038 #define PLATFORMSPECIFICFUNCTIONS_C_H_ 00039 00040 #ifdef __cplusplus 00041 extern "C" { 00042 #endif 00043 00044 /* Jumping operations. They manage their own jump buffers */ 00045 int PlatformSpecificSetJmp(void (*function) (void*), void* data); 00046 void PlatformSpecificLongJmp(void); 00047 void PlatformSpecificRestoreJumpBuffer(void); 00048 00049 /* Time operations */ 00050 long GetPlatformSpecificTimeInMillis(void); 00051 void SetPlatformSpecificTimeInMillisMethod(long(*platformSpecific)(void)); 00052 00053 const char* GetPlatformSpecificTimeString(void); 00054 void SetPlatformSpecificTimeStringMethod(const char* (*platformMethod)(void)); 00055 00056 /* String operations */ 00057 int PlatformSpecificAtoI(const char*str); 00058 size_t PlatformSpecificStrLen(const char* str); 00059 int PlatformSpecificStrCmp(const char* s1, const char* s2); 00060 int PlatformSpecificStrNCmp(const char* s1, const char* s2, size_t size); 00061 char* PlatformSpecificStrStr(const char* s1, const char* s2); 00062 00063 int PlatformSpecificVSNprintf(char *str, size_t size, const char* format, 00064 va_list va_args_list); 00065 00066 char PlatformSpecificToLower(char c); 00067 00068 /* Misc */ 00069 double PlatformSpecificFabs(double d); 00070 int PlatformSpecificIsNan(double d); 00071 int PlatformSpecificAtExit(void(*func)(void)); 00072 00073 /* IO operations */ 00074 typedef void* PlatformSpecificFile; 00075 00076 PlatformSpecificFile PlatformSpecificFOpen(const char* filename, 00077 const char* flag); 00078 void PlatformSpecificFPuts(const char* str, PlatformSpecificFile file); 00079 void PlatformSpecificFClose(PlatformSpecificFile file); 00080 00081 int PlatformSpecificPutchar(int c); 00082 void PlatformSpecificFlush(void); 00083 00084 /* Dynamic Memory operations */ 00085 void* PlatformSpecificMalloc(size_t size); 00086 void* PlatformSpecificRealloc(void* memory, size_t size); 00087 void PlatformSpecificFree(void* memory); 00088 void* PlatformSpecificMemCpy(void* s1, const void* s2, size_t size); 00089 void* PlatformSpecificMemset(void* mem, int c, size_t size); 00090 00091 #ifdef __cplusplus 00092 } 00093 #endif 00094 00095 #endif /* PLATFORMSPECIFICFUNCTIONS_C_H_ */
Generated on Tue Jul 12 2022 21:43:37 by
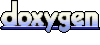