Unit Testing framework based on http://cpputest.github.io/
Embed:
(wiki syntax)
Show/hide line numbers
CppUTestConfig.h
00001 /* 00002 * Copyright (c) 2007, Michael Feathers, James Grenning and Bas Vodde 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without 00006 * modification, are permitted provided that the following conditions are met: 00007 * * Redistributions of source code must retain the above copyright 00008 * notice, this list of conditions and the following disclaimer. 00009 * * Redistributions in binary form must reproduce the above copyright 00010 * notice, this list of conditions and the following disclaimer in the 00011 * documentation and/or other materials provided with the distribution. 00012 * * Neither the name of the <organization> nor the 00013 * names of its contributors may be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE EARLIER MENTIONED AUTHORS ``AS IS'' AND ANY 00017 * EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00018 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00019 * DISCLAIMED. IN NO EVENT SHALL <copyright holder> BE LIABLE FOR ANY 00020 * DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00021 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00022 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00023 * ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00024 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00025 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00026 */ 00027 00028 00029 #ifndef CPPUTESTCONFIG_H_ 00030 #define CPPUTESTCONFIG_H_ 00031 00032 #ifdef HAVE_CONFIG_H 00033 #include "config.h" 00034 #endif 00035 00036 /* 00037 * This file is added for some specific CppUTest configurations that earlier were spread out into multiple files. 00038 * 00039 * The goal of this file is to stay really small and not to include other things, but mainly to remove duplication 00040 * from other files and resolve dependencies in #includes. 00041 * 00042 */ 00043 00044 /* 00045 * Lib C dependencies that are currently still left: 00046 * 00047 * stdarg.h -> We use formatting functions and va_list requires to include stdarg.h in SimpleString 00048 * stdlib.h -> The TestHarness_c.h includes this to try to avoid conflicts in its malloc #define. This dependency can 00049 * easily be removed by not enabling the MALLOC overrides. 00050 * 00051 * Lib C++ dependencies are all under the CPPUTEST_USE_STD_CPP_LIB. 00052 * The only dependency is to <new> which has the bad_alloc struct 00053 * 00054 */ 00055 00056 /* Do we use Standard C or not? When doing Kernel development, standard C usage is out. */ 00057 #ifndef CPPUTEST_USE_STD_C_LIB 00058 #ifdef CPPUTEST_STD_C_LIB_DISABLED 00059 #define CPPUTEST_USE_STD_C_LIB 0 00060 #else 00061 #define CPPUTEST_USE_STD_C_LIB 1 00062 #endif 00063 #endif 00064 00065 #if NEED_TO_ENABLE_CPP_SUPPORT /* disabled by default because our 00066 * toolchain doesn't support exceptions. */ 00067 /* Do we use Standard C++ or not? */ 00068 #ifndef CPPUTEST_USE_STD_CPP_LIB 00069 #ifdef CPPUTEST_STD_CPP_LIB_DISABLED 00070 #define CPPUTEST_USE_STD_CPP_LIB 0 00071 #else 00072 #define CPPUTEST_USE_STD_CPP_LIB 1 00073 #endif 00074 #endif 00075 #endif /* #if NEED_TO_ENABLE_CPP_SUPPORT */ 00076 00077 /* Is memory leak detection enabled? 00078 * Controls the override of the global operator new/deleted and malloc/free. 00079 * Without this, there will be no memory leak detection in C/C++. 00080 */ 00081 #if NEED_TO_ENABLE_MEMORY_LEAK_DETECTION 00082 #ifndef CPPUTEST_USE_MEM_LEAK_DETECTION 00083 #ifdef CPPUTEST_MEM_LEAK_DETECTION_DISABLED 00084 #define CPPUTEST_USE_MEM_LEAK_DETECTION 0 00085 #else 00086 #define CPPUTEST_USE_MEM_LEAK_DETECTION 1 00087 #endif 00088 #endif 00089 #endif /* #if NEED_TO_ENABLE_MEMORY_LEAK_DETECTION */ 00090 00091 /* Create a __no_return__ macro, which is used to flag a function as not returning. 00092 * Used for functions that always throws for instance. 00093 * 00094 * This is needed for compiling with clang, without breaking other compilers. 00095 */ 00096 #ifndef __has_attribute 00097 #define __has_attribute(x) 0 00098 #endif 00099 00100 #if __has_attribute(noreturn) 00101 #define __no_return__ __attribute__((noreturn)) 00102 #else 00103 #define __no_return__ 00104 #endif 00105 00106 #if __has_attribute(format) 00107 #define __check_format__(type, format_parameter, other_parameters) __attribute__ ((format (type, format_parameter, other_parameters))) 00108 #else 00109 #define __check_format__(type, format_parameter, other_parameters) /* type, format_parameter, other_parameters */ 00110 #endif 00111 00112 /* 00113 * When we don't link Standard C++, then we won't throw exceptions as we assume the compiler might not support that! 00114 */ 00115 00116 #if CPPUTEST_USE_STD_CPP_LIB 00117 #if defined(__cplusplus) && __cplusplus >= 201103L 00118 #define UT_THROW(exception) 00119 #define UT_NOTHROW noexcept 00120 #else 00121 #define UT_THROW(exception) throw (exception) 00122 #define UT_NOTHROW throw() 00123 #endif 00124 #else 00125 #define UT_THROW(exception) 00126 #ifdef __clang__ 00127 #define UT_NOTHROW throw() 00128 #else 00129 #define UT_NOTHROW 00130 #endif 00131 #endif 00132 00133 /* 00134 * Visual C++ doesn't define __cplusplus as C++11 yet (201103), however it doesn't want the throw(exception) either, but 00135 * it does want throw(). 00136 */ 00137 00138 #ifdef _MSC_VER 00139 #undef UT_THROW 00140 #define UT_THROW(exception) 00141 #endif 00142 00143 #if defined(__cplusplus) && __cplusplus >= 201103L 00144 #define DEFAULT_COPY_CONSTRUCTOR(classname) classname(const classname &) = default; 00145 #else 00146 #define DEFAULT_COPY_CONSTRUCTOR(classname) 00147 #endif 00148 00149 /* 00150 * g++-4.7 with stdc++11 enabled On MacOSX! will have a different exception specifier for operator new (and thank you!) 00151 * I assume they'll fix this in the future, but for now, we'll change that here. 00152 * (This should perhaps also be done in the configure.ac) 00153 */ 00154 00155 #ifdef __GXX_EXPERIMENTAL_CXX0X__ 00156 #ifdef __APPLE__ 00157 #ifdef _GLIBCXX_THROW 00158 #undef UT_THROW 00159 #define UT_THROW(exception) _GLIBCXX_THROW(exception) 00160 #endif 00161 #endif 00162 #endif 00163 00164 /* 00165 * Detection of different 64 bit environments 00166 */ 00167 00168 #if defined(__LP64__) || defined(_LP64) || (defined(__WORDSIZE) && (__WORDSIZE == 64 )) || defined(__x86_64) || defined(_WIN64) 00169 #define CPPUTEST_64BIT 00170 #if defined(_WIN64) 00171 #define CPPUTEST_64BIT_32BIT_LONGS 00172 #endif 00173 #endif 00174 00175 /* Visual C++ 10.0+ (2010+) supports the override keyword, but doesn't define the C++ version as C++11 */ 00176 #if defined(__cplusplus) && ((__cplusplus >= 201103L) || (defined(_MSC_VER) && (_MSC_VER >= 1600))) 00177 #define _override override 00178 #else 00179 #define _override 00180 #endif 00181 00182 /* Should be the only #include here. Standard C library wrappers */ 00183 #include "StandardCLibrary.h" 00184 00185 #endif
Generated on Tue Jul 12 2022 21:43:37 by
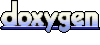