Unit Testing framework based on http://cpputest.github.io/
Embed:
(wiki syntax)
Show/hide line numbers
CommandLineArguments.h
00001 /* 00002 * Copyright (c) 2007, Michael Feathers, James Grenning and Bas Vodde 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without 00006 * modification, are permitted provided that the following conditions are met: 00007 * * Redistributions of source code must retain the above copyright 00008 * notice, this list of conditions and the following disclaimer. 00009 * * Redistributions in binary form must reproduce the above copyright 00010 * notice, this list of conditions and the following disclaimer in the 00011 * documentation and/or other materials provided with the distribution. 00012 * * Neither the name of the <organization> nor the 00013 * names of its contributors may be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE EARLIER MENTIONED AUTHORS ``AS IS'' AND ANY 00017 * EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00018 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00019 * DISCLAIMED. IN NO EVENT SHALL <copyright holder> BE LIABLE FOR ANY 00020 * DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00021 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00022 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00023 * ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00024 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00025 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00026 */ 00027 00028 #ifndef D_CommandLineArguments_H 00029 #define D_CommandLineArguments_H 00030 00031 #include "SimpleString.h" 00032 #include "TestOutput.h" 00033 #include "TestFilter.h" 00034 00035 class TestPlugin; 00036 00037 class CommandLineArguments 00038 { 00039 public: 00040 explicit CommandLineArguments(int ac, const char** av); 00041 virtual ~CommandLineArguments(); 00042 00043 bool parse(TestPlugin* plugin); 00044 bool isVerbose() const; 00045 int getRepeatCount() const; 00046 TestFilter getGroupFilter() const; 00047 TestFilter getNameFilter() const; 00048 bool isJUnitOutput() const; 00049 bool isEclipseOutput() const; 00050 bool runTestsInSeperateProcess() const; 00051 const SimpleString& getPackageName() const; 00052 const char* usage() const; 00053 00054 private: 00055 00056 enum OutputType 00057 { 00058 OUTPUT_ECLIPSE, OUTPUT_JUNIT 00059 }; 00060 int ac_; 00061 const char** av_; 00062 00063 bool verbose_; 00064 bool runTestsAsSeperateProcess_; 00065 int repeat_; 00066 TestFilter groupFilter_; 00067 TestFilter nameFilter_; 00068 OutputType outputType_; 00069 SimpleString packageName_; 00070 00071 SimpleString getParameterField(int ac, const char** av, int& i, const SimpleString& parameterName); 00072 void SetRepeatCount(int ac, const char** av, int& index); 00073 void SetGroupFilter(int ac, const char** av, int& index); 00074 void SetStrictGroupFilter(int ac, const char** av, int& index); 00075 void SetNameFilter(int ac, const char** av, int& index); 00076 void SetStrictNameFilter(int ac, const char** av, int& index); 00077 void SetTestToRunBasedOnVerboseOutput(int ac, const char** av, int& index, const char* parameterName); 00078 bool SetOutputType(int ac, const char** av, int& index); 00079 void SetPackageName(int ac, const char** av, int& index); 00080 00081 CommandLineArguments(const CommandLineArguments&); 00082 CommandLineArguments& operator=(const CommandLineArguments&); 00083 00084 }; 00085 00086 #endif
Generated on Tue Jul 12 2022 21:43:37 by
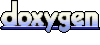