This driver is meant for the monochrome LCD display (model no: LS013B4DN04) from Sharp; but it should be easily adaptable to other Sharp displays.
font.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include <stdint.h> 00018 00019 #ifndef __GUI_FONT_H__ 00020 #define __GUI_FONT_H__ 00021 00022 #ifdef __cplusplus 00023 extern "C" { 00024 #endif 00025 00026 /*---------------------------------------------------------------------- 00027 * Type declarations 00028 *----------------------------------------------------------------------*/ 00029 00030 typedef struct glyph_t { 00031 int8_t bearingX; /* The horizontal distance from the 00032 * current pen position to the glyph's 00033 * left bbox edge. */ 00034 int8_t bearingY; /* The vertical distance from the 00035 * baseline to the top of the glyph's 00036 * bbox. */ 00037 unsigned int width : 6; /* The glyph's horizontal extent. */ 00038 unsigned int height : 6; /* The glyph's vertical extent. */ 00039 unsigned int advance : 6; /* The horizontal distance the 00040 * pen position must be 00041 * incremented (for 00042 * left-to-right writing) or 00043 * decremented (for 00044 * right-to-left writing) by 00045 * after each glyph is rendered 00046 * when processing text. */ 00047 unsigned int bitmapIndex : 14; /* byte-index of the start of 00048 * the glyph's bitmap as 00049 * contained in the font-face 00050 * bitmaps array */ 00051 } __attribute__((__packed__)) glyph_t; 00052 00053 typedef struct font_face_t { 00054 const char *familyName; 00055 unsigned int pointSize; 00056 const glyph_t *glyphs; 00057 const uint8_t *bitmaps; 00058 } font_face_t; 00059 00060 00061 #define FONT_LOWEST_SUPPORTED_CHAR_ENCODING ' ' 00062 #define FONT_HIGHEST_SUPPORTED_CHAR_ENCODING '~' 00063 00064 00065 typedef enum font_color_t { 00066 BLACK = 0, 00067 WHITE = 1 00068 } font_color_t; 00069 00070 00071 /*---------------------------------------------------------------------- 00072 * External globals 00073 *----------------------------------------------------------------------*/ 00074 00075 extern const font_face_t fonts[]; 00076 00077 00078 /*---------------------------------------------------------------------- 00079 * Exported functions. 00080 *----------------------------------------------------------------------*/ 00081 00082 extern const font_face_t *lookupFontFace(const char *familyName, 00083 unsigned int pointSize); 00084 00085 #ifdef __cplusplus 00086 } 00087 #endif 00088 00089 #endif /* __GUI_FONT_H__ */
Generated on Tue Jul 12 2022 19:17:11 by
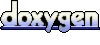