This driver is meant for the monochrome LCD display (model no: LS013B4DN04) from Sharp; but it should be easily adaptable to other Sharp displays.
font.c
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include <string.h> 00018 #include <stddef.h> 00019 00020 #include "font.h" 00021 00022 /* Externs private to the font subsystem. */ 00023 /* extern const glyph_t glyphs_DejaVu_Serif_10[]; */ 00024 /* extern const uint8_t bitmaps_DejaVu_Serif_10[]; */ 00025 extern const glyph_t glyphs_DejaVu_Serif_9[]; 00026 extern const uint8_t bitmaps_DejaVu_Serif_9[]; 00027 extern const glyph_t glyphs_DejaVu_Serif_8[]; 00028 extern const uint8_t bitmaps_DejaVu_Serif_8[]; 00029 00030 /* Accumulation of all avaialble fonts */ 00031 const font_face_t fonts[] = { 00032 /* { */ 00033 /* "DejaVu Serif", */ 00034 /* 10, /\* pointSize *\/ */ 00035 /* glyphs_DejaVu_Serif_10, */ 00036 /* bitmaps_DejaVu_Serif_10 */ 00037 /* }, */ 00038 { 00039 "DejaVu Serif", 00040 9, /* pointSize */ 00041 glyphs_DejaVu_Serif_9, 00042 bitmaps_DejaVu_Serif_9 00043 }, 00044 { 00045 "DejaVu Serif", 00046 8, /* pointSize */ 00047 glyphs_DejaVu_Serif_8, 00048 bitmaps_DejaVu_Serif_8 00049 }, 00050 00051 /* sentinel value */ 00052 { 00053 NULL, 00054 0, 00055 NULL, 00056 NULL 00057 } 00058 }; 00059 00060 00061 const font_face_t * 00062 lookupFontFace(const char *familyName, 00063 unsigned int pointSize) 00064 { 00065 unsigned fontIndex; 00066 00067 for (fontIndex = 0; fonts[fontIndex].familyName != NULL; fontIndex++) { 00068 if ((strcmp(fonts[fontIndex].familyName, familyName) == 0) && 00069 (fonts[fontIndex].pointSize == pointSize)) { 00070 /* found it! */ 00071 return (&fonts[fontIndex]); 00072 } 00073 } 00074 00075 return (NULL); 00076 }
Generated on Tue Jul 12 2022 19:17:11 by
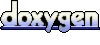