MEMS pressure sensor by STMicroelectronics. FIFO Hardware digital filter as default.
Fork of LPS25H by
LPS25H.h
00001 /* 00002 * mbed library program 00003 * LPS25H MEMS pressure sensor: 260-1260 hPa absolute digital output barometer 00004 * made by STMicroelectronics 00005 * http://www.st-japan.co.jp/web/catalog/sense_power/FM89/SC1316/PF255230 00006 * 00007 * Copyright (c) 2015,'17 Kenji Arai / JH1PJL 00008 * http://www.page.sannet.ne.jp/kenjia/index.html 00009 * http://mbed.org/users/kenjiArai/ 00010 * Created: Feburary 21st, 2015 00011 * Revised: August 21st, 2017 00012 */ 00013 /* 00014 *---------------- REFERENCE --------------------------------------------------- 00015 * Original Information 00016 * http://www.st.com/content/st_com/ja/products/ 00017 * mems-and-sensors/pressure-sensors/lps25h.html 00018 * AN4450 Application Note (as follows) 00019 * en.DM00108837.pdf 00020 * Device kit (not avairable) 00021 * http://akizukidenshi.com/catalog/g/gK-08338/ 00022 */ 00023 00024 #ifndef LPS25H_H 00025 #define LPS25H_H 00026 00027 #include "mbed.h" 00028 00029 // LPS25H Address 00030 // 7bit address = 0b101110x(0x5c or 0x5d depends on SA0/SDO) 00031 #define LPS25H_G_CHIP_ADDR (0x5c << 1) // SA0(=SDO pin) = Ground 00032 #define LPS25H_V_CHIP_ADDR (0x5d << 1) // SA0(=SDO pin) = Vdd 00033 00034 // MODE Selection 00035 #define FIFO_HW_FILTER 1 00036 #define FIFO_BYPASS 0 00037 00038 // LPS25H ID 00039 #define I_AM_LPS25H 0xbd 00040 00041 // Register's definition 00042 #define LPS25H_WHO_AM_I 0x0f 00043 #define LPS25H_RES_CONF 0x10 00044 00045 #define LPS25H_CTRL_REG1 0x20 00046 #define LPS25H_CTRL_REG2 0x21 00047 #define LPS25H_CTRL_REG3 0x22 00048 #define LPS25H_CTRL_REG4 0x23 00049 00050 #define LPS25H_STATUS_REG 0x27 00051 #define LPS25H_PRESS_POUT_XL 0x28 00052 #define LPS25H_PRESS_OUT_L 0x29 00053 #define LPS25H_PRESS_OUT_H 0x2a 00054 #define LPS25H_TEMP_OUT_L 0x2b 00055 #define LPS25H_TEMP_OUT_H 0x2c 00056 00057 #define LPS25H_FIFO_CTRL 0x2e 00058 #define LPS25H_FIFO_STATUS 0x2e 00059 00060 // Control Reg. 00061 #define PD (0UL << 7) 00062 #define ACTIVE (1UL << 7) 00063 #define ODR_ONESHOT (0UL << 4) 00064 #define ODR_1HZ (1UL << 4) 00065 #define ODR_7HZ (1UL << 4) 00066 #define ODR_12R5HZ (2UL << 4) 00067 #define ODR_25HZ (3UL << 4) 00068 #define BDU_SET (1UL << 2) 00069 #define CR_STD_SET (ACTIVE + ODR_7HZ + BDU_SET) 00070 00071 // FIFO Control 00072 #define FIFO_MEAN_MODE 0xc0 00073 #define FIFO_SAMPLE_2 0x01 00074 #define FIFO_SAMPLE_4 0x03 00075 #define FIFO_SAMPLE_8 0x07 00076 #define FIFO_SAMPLE_16 0x0f 00077 #define FIFO_SAMPLE_32 0x1f 00078 00079 /** Interface for STMicronics MEMS pressure sensor 00080 * Chip: LPS25H 00081 * 00082 * @code 00083 * #include "mbed.h" 00084 * #include "LPS25H.h" 00085 * 00086 * // I2C Communication 00087 * LPS25H baro(p_sda, p_scl, LPS25H_G_CHIP_ADDR); 00088 * // If you connected I2C line not only this device but also other devices, 00089 * // you need to declare following method. 00090 * I2C i2c(dp5,dp27); // SDA, SCL 00091 * LPS25H baro(i2c, LPS25H_G_CHIP_ADDR); 00092 * 00093 * int main() { 00094 * while( trure){ 00095 * baro.get(); 00096 * printf("Presere: 0x%6.1f, Temperature: 0x%+4.1f\r\n", 00097 * baro.pressue(), baro.temperature()); 00098 * wait(1.0); 00099 * } 00100 * } 00101 * @endcode 00102 */ 00103 00104 class LPS25H 00105 { 00106 public: 00107 /** Configure data pin 00108 * @param data SDA and SCL pins 00109 * @param device address LPS25H(SA0=0 or 1), LPS25H_G_CHIP_ADDR or _V_ 00110 * @param Operation mode FIFO_HW_FILTER(default) or FIFO_BYPASS 00111 */ 00112 LPS25H(PinName p_sda, PinName p_scl); 00113 00114 // LPS25H(PinName p_sda, PinName p_scl, uint8_t addr, uint8_t mode); 00115 00116 /** Configure data pin (with other devices on I2C line) 00117 * @param I2C previous definition 00118 * @param device address LPS25H(SA0=0 or 1), LPS25H_G_CHIP_ADDR or _V_ 00119 * @param Operation mode FIFO_HW_FILTER(default) or FIFO_BYPASS 00120 */ 00121 // LPS25H(I2C& p_i2c, uint8_t addr); 00122 // LPS25H(I2C& p_i2c, uint8_t addr, uint8_t mode); 00123 00124 /** Start convertion & data save 00125 * @param none 00126 * @return none 00127 */ 00128 void get(void); 00129 00130 /** Read pressure data 00131 * @param none 00132 * @return pressure in hpa 00133 */ 00134 float pressure(void); 00135 00136 /** Read temperature data 00137 * @param none 00138 * @return temperature 00139 */ 00140 float temperature(void); 00141 00142 /** Read a ID number 00143 * @param none 00144 * @return if STM MEMS LPS25H, it should be I_AM_ LPS25H 00145 */ 00146 /** Read altitude data 00147 * @param none 00148 * @return altitude in metters 00149 */ 00150 float altitude(void); 00151 00152 /** Read temperature data 00153 * @param none 00154 * @return temperature 00155 */ 00156 00157 //uint8_t read_id(void); 00158 00159 /** Read Data Ready flag 00160 * @param none 00161 * @return 1 = Ready 00162 */ 00163 uint8_t data_ready(void); 00164 00165 /** Set I2C clock frequency 00166 * @param freq. 00167 * @return none 00168 */ 00169 void frequency(int hz); 00170 00171 /** Read register (general purpose) 00172 * @param register's address 00173 * @return register data 00174 */ 00175 uint8_t read_reg(uint8_t addr); 00176 00177 /** Write register (general purpose) 00178 * @param register's address 00179 * @param data 00180 * @return none 00181 */ 00182 void write_reg(uint8_t addr, uint8_t data); 00183 00184 protected: 00185 I2C *_i2c_p; 00186 I2C &_i2c; 00187 00188 void init(void); 00189 00190 private: 00191 char dt[6]; // working buffer 00192 uint8_t LPS25H_addr; // Sensor address 00193 //uint8_t LPS25H_id; // ID 00194 uint8_t LPS25H_ready; // Device is on I2C line = 1, not = 0 00195 uint8_t LPS25H_mode; // Operation mode 00196 uint32_t press; // pressure raw data 00197 //int16_t temp; // temperature raw data 00198 int32_t alt; 00199 }; 00200 00201 #endif // LPS25H_H
Generated on Tue Jul 19 2022 15:15:57 by
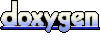