
brw1
Embed:
(wiki syntax)
Show/hide line numbers
TextDisplay.cpp
00001 /* mbed TextDisplay Display Library Base Class 00002 * Copyright (c) 2007-2009 sford 00003 * Released under the MIT License: http://mbed.org/license/mit 00004 */ 00005 00006 #include "TextDisplay.h" 00007 00008 //#define DEBUG "Text" 00009 // ... 00010 // INFO("Stuff to show %d", var); // new-line is automatically appended 00011 // 00012 #if (defined(DEBUG) && !defined(TARGET_LPC11U24)) 00013 #define INFO(x, ...) std::printf("[INF %s %3d] "x"\r\n", DEBUG, __LINE__, ##__VA_ARGS__); 00014 #define WARN(x, ...) std::printf("[WRN %s %3d] "x"\r\n", DEBUG, __LINE__, ##__VA_ARGS__); 00015 #define ERR(x, ...) std::printf("[ERR %s %3d] "x"\r\n", DEBUG, __LINE__, ##__VA_ARGS__); 00016 #else 00017 #define INFO(x, ...) 00018 #define WARN(x, ...) 00019 #define ERR(x, ...) 00020 #endif 00021 00022 TextDisplay::TextDisplay(const char *name) : Stream(name) 00023 { 00024 _row = 0; 00025 _column = 0; 00026 if (name == NULL) { 00027 _path = NULL; 00028 } else { 00029 _path = new char[strlen(name) + 2]; 00030 sprintf(_path, "/%s", name); 00031 } 00032 } 00033 00034 int TextDisplay::_putc(int value) 00035 { 00036 INFO("_putc(%d)", value); 00037 if(value == '\n') { 00038 _column = 0; 00039 _row++; 00040 if(_row >= rows()) { 00041 _row = 0; 00042 } 00043 } else { 00044 character(_column, _row, value); 00045 _column++; 00046 if(_column >= columns()) { 00047 _column = 0; 00048 _row++; 00049 if(_row >= rows()) { 00050 _row = 0; 00051 } 00052 } 00053 } 00054 return value; 00055 } 00056 00057 // crude cls implementation, should generally be overwritten in derived class 00058 RetCode_t TextDisplay::cls(uint16_t layers) 00059 { 00060 INFO("cls()"); 00061 locate(0, 0); 00062 for(int i=0; i<columns()*rows(); i++) { 00063 putc(' '); 00064 } 00065 return noerror; 00066 } 00067 00068 RetCode_t TextDisplay::locate(textloc_t column, textloc_t row) 00069 { 00070 INFO("locate(%d,%d)", column, row); 00071 _column = column; 00072 _row = row; 00073 return noerror; 00074 } 00075 00076 int TextDisplay::_getc() 00077 { 00078 return -1; 00079 } 00080 00081 RetCode_t TextDisplay::foreground(uint16_t color) 00082 { 00083 //INFO("foreground(%4X)", color); 00084 _foreground = color; 00085 return noerror; 00086 } 00087 00088 RetCode_t TextDisplay::background(uint16_t color) 00089 { 00090 //INFO("background(%4X)", color); 00091 _background = color; 00092 return noerror; 00093 } 00094 00095 bool TextDisplay::claim(FILE *stream) 00096 { 00097 if ( _path == NULL) { 00098 fprintf(stderr, "claim requires a name to be given in the instantiator of the TextDisplay instance!\r\n"); 00099 return false; 00100 } 00101 if (freopen(_path, "w", stream) == NULL) { 00102 return false; // Failed, should not happen 00103 } 00104 // make sure we use line buffering 00105 setvbuf(stdout, NULL, _IOLBF, columns()); 00106 return true; 00107 } 00108
Generated on Fri Jul 15 2022 05:44:41 by
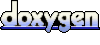