
Ported Version of emwin eink and modus toolbox tft for psoc 6 cy8ckit wifi bt and cy8ckit-028-tft display. Works with the WiFi-BT pioneer kit hardware.
main.cpp
00001 #include "mbed.h" 00002 #include "GUI.h" 00003 #include "cy8ckit_028_tft.h" 00004 #define Cy_SysLib_Delay wait_ms 00005 00006 DigitalOut ledGreen(LED_GREEN); 00007 DigitalIn sw2(SWITCH2, PullUp); 00008 DigitalOut ledRed(LED_RED); 00009 DigitalOut ledBlue(LED_BLUE); 00010 00011 DigitalInOut LCD_REG0(P9_0); 00012 DigitalInOut LCD_REG1(P9_1); 00013 DigitalInOut LCD_REG2(P9_2); 00014 DigitalInOut LCD_REG3(P9_4); 00015 DigitalInOut LCD_REG4(P9_5); 00016 DigitalInOut LCD_REG5(P0_2); 00017 DigitalInOut LCD_REG6(P13_0); 00018 DigitalInOut LCD_REG7(P13_1); 00019 00020 DigitalOut LCD_NWR(P12_0); 00021 DigitalOut LCD_DC(P12_1); 00022 DigitalOut LCD_RESET(P12_2); 00023 DigitalOut LCD_NRD(P12_3); 00024 00025 #define NUMBER_OF_DEMO_PAGES (10u) 00026 00027 00028 /* Macros for switch press status */ 00029 #define BTN_PRESSED (0u) 00030 #define BTN_RELEASED (1u) 00031 00032 /* Macros to control LEDs */ 00033 #define LED_ON (0) 00034 #define LED_OFF (1) 00035 00036 00037 00038 /* External global references */ 00039 //extern GUI_CONST_STORAGE GUI_BITMAP bmCypressLogo_1bpp; 00040 00041 extern GUI_CONST_STORAGE GUI_BITMAP bmExampleImage; 00042 extern GUI_CONST_STORAGE GUI_BITMAP bmCypressLogo; 00043 00044 /* Function prototypes */ 00045 void ShowTextModes(void); 00046 void ShowTextColors(void); 00047 void ShowFontSizesNormal(void); 00048 void ShowFontSizesBold(void); 00049 void ShowColorBar(void); 00050 void Show2DGraphics1(void); 00051 void Show2DGraphics2(void); 00052 void ShowConcentricCircles(void); 00053 void ShowTextWrapAndOrientation(void); 00054 void ShowBitmap(void); 00055 00056 /* Array of demo pages functions */ 00057 void (*demoPageArray[NUMBER_OF_DEMO_PAGES])(void) = { 00058 ShowTextModes, 00059 ShowTextColors, 00060 ShowFontSizesNormal, 00061 ShowFontSizesBold, 00062 ShowTextWrapAndOrientation, 00063 ShowColorBar, 00064 Show2DGraphics1, 00065 Show2DGraphics2, 00066 ShowConcentricCircles, 00067 ShowBitmap 00068 }; 00069 00070 00071 /******************************************************************************* 00072 * Function Name: void ShowStartupScreen(void) 00073 ******************************************************************************** 00074 * 00075 * Summary: This function displays the startup screen. 00076 * 00077 * Parameters: 00078 * None 00079 * 00080 * Return: 00081 * None 00082 * 00083 *******************************************************************************/ 00084 void ShowStartupScreen(void) 00085 { 00086 /* Set font size, foreground and background Colours */ 00087 GUI_SetFont(GUI_FONT_16B_1); 00088 GUI_SetColor(GUI_WHITE); 00089 GUI_SetBkColor(GUI_BLACK); 00090 00091 /* Clear the screen */ 00092 GUI_Clear(); 00093 00094 /* Draw the Cypress Logo */ 00095 GUI_DrawBitmap(&bmCypressLogo, 4, 4); 00096 00097 /* Print the text CYPRESS EMWIN GRAPHICS DEMO TFT DISPLAY */ 00098 GUI_SetTextAlign(GUI_TA_HCENTER); 00099 GUI_DispStringAt("CYPRESS", 160, 120); 00100 GUI_SetTextAlign(GUI_TA_HCENTER); 00101 GUI_DispStringAt("EMWIN GRAPHICS DEMO", 160, 140); 00102 GUI_SetTextAlign(GUI_TA_HCENTER); 00103 GUI_DispStringAt("TFT DISPLAY", 160, 160); 00104 } 00105 00106 00107 /******************************************************************************* 00108 * Function Name: void ShowInstructionsScreen(void) 00109 ******************************************************************************** 00110 * 00111 * Summary: This function shows screen with instructions to press SW2 to 00112 * scroll through various display pages 00113 * 00114 * Parameters: 00115 * None 00116 * 00117 * Return: 00118 * None 00119 * 00120 *******************************************************************************/ 00121 void ShowInstructionsScreen(void) 00122 { 00123 /* Set font size, background Colour and text mode */ 00124 GUI_SetFont(GUI_FONT_16B_1); 00125 GUI_SetBkColor(GUI_BLACK); 00126 GUI_SetColor(GUI_WHITE); 00127 GUI_SetTextMode(GUI_TM_NORMAL); 00128 00129 /* Clear the display */ 00130 GUI_Clear(); 00131 00132 /* Display instructions text */ 00133 GUI_SetTextAlign(GUI_TA_HCENTER); 00134 GUI_DispStringAt("PRESS SW2 ON THE KIT", 160, 90); 00135 GUI_SetTextAlign(GUI_TA_HCENTER); 00136 GUI_DispStringAt("TO SCROLL THROUGH ", 160, 110); 00137 GUI_SetTextAlign(GUI_TA_HCENTER); 00138 GUI_DispStringAt("DEMO PAGES!", 160, 130); 00139 } 00140 00141 00142 /******************************************************************************* 00143 * Function Name: void ShowTextModes(void) 00144 ******************************************************************************** 00145 * 00146 * Summary: This function displays the following 00147 * 1. Left, Center and Right aligned text 00148 * 2. Underline, overline and strikethrough style text 00149 * 3. Normal, reverse, transparent and XOR text modes 00150 * 00151 * Parameters: 00152 * None 00153 * 00154 * Return: 00155 * None 00156 * 00157 *******************************************************************************/ 00158 void ShowTextModes(void) 00159 { 00160 /* Set font size, foreground and background Colours */ 00161 GUI_SetFont(GUI_FONT_16B_1); 00162 GUI_SetColor(GUI_WHITE); 00163 GUI_SetBkColor(GUI_BLACK); 00164 GUI_SetTextMode(GUI_TM_NORMAL); 00165 GUI_SetTextStyle(GUI_TS_NORMAL); 00166 00167 /* Clear the screen */ 00168 GUI_Clear(); 00169 00170 /* Display page title */ 00171 GUI_SetTextAlign(GUI_TA_HCENTER); 00172 GUI_SetTextStyle(GUI_TS_UNDERLINE); 00173 GUI_DispStringAt("1 OF 10: TEXT ALIGNMENTS, STYLES AND MODES", 160, 10); 00174 GUI_SetTextStyle(GUI_TS_NORMAL); 00175 00176 /* Display left aligned text */ 00177 GUI_SetTextAlign(GUI_TA_LEFT); 00178 GUI_DispStringAt("TEXT ALIGNMENT LEFT", 0, 40); 00179 00180 /* Display center aligned text */ 00181 GUI_SetTextAlign(GUI_TA_HCENTER); 00182 GUI_DispStringAt("TEXT ALIGNMENT CENTER", 160, 60); 00183 00184 /* Display right aligned text */ 00185 GUI_SetTextAlign(GUI_TA_RIGHT); 00186 GUI_DispStringAt("TEXT ALIGNMENT RIGHT", 319, 80); 00187 00188 /* Display underlined text */ 00189 GUI_SetTextStyle(GUI_TS_UNDERLINE); 00190 GUI_SetTextAlign(GUI_TA_LEFT); 00191 GUI_DispStringAt("TEXT STYLE UNDERLINE", 0, 100); 00192 00193 /* Display overlined text */ 00194 GUI_SetTextStyle(GUI_TS_OVERLINE); 00195 GUI_SetTextAlign(GUI_TA_LEFT); 00196 GUI_DispStringAt("TEXT STYLE OVERLINE", 0, 120); 00197 00198 /* Display strikethrough text */ 00199 GUI_SetTextStyle(GUI_TS_STRIKETHRU); 00200 GUI_SetTextAlign(GUI_TA_LEFT); 00201 GUI_DispStringAt("TEXT STYLE STRIKETHROUGH", 0, 140); 00202 00203 /* Create a rectangle filled with blue Colour */ 00204 GUI_SetColor(GUI_BROWN); 00205 GUI_FillRect(0, 160, 319, 239); 00206 00207 /* Draw two diagonal lines */ 00208 GUI_SetColor(GUI_BLUE); 00209 GUI_SetPenSize(3); 00210 GUI_DrawLine(0, 160, 319, 239); 00211 GUI_DrawLine(0, 239, 319, 160); 00212 00213 /* Set text Colour to white with black background */ 00214 GUI_SetColor(GUI_WHITE); 00215 GUI_SetBkColor(GUI_BLACK); 00216 00217 /* Set text style to normal */ 00218 GUI_SetTextStyle(GUI_TS_NORMAL); 00219 00220 /* Display text in normal mode. This will print white text in 00221 a black box */ 00222 GUI_SetTextAlign(GUI_TA_HCENTER); 00223 GUI_SetTextMode(GUI_TM_NORMAL); 00224 GUI_DispStringAt("TEXT MODE NORMAL", 160, 165); 00225 00226 /* Display text in reverse mode. This will print black text n 00227 a white box */ 00228 GUI_SetTextAlign(GUI_TA_HCENTER); 00229 GUI_SetTextMode(GUI_TM_REV); 00230 GUI_DispStringAt("TEXT MODE REVERSE", 160, 185); 00231 00232 /* Display transparent text. This will display white text 00233 on the blue background already present in the rectangle */ 00234 GUI_SetTextAlign(GUI_TA_HCENTER); 00235 GUI_SetTextMode(GUI_TM_TRANS); 00236 GUI_DispStringAt("TEXT MODE TRANSPARENT", 160, 205); 00237 00238 /* Display XOR text. This will XOR the blue background with 00239 the white text */ 00240 GUI_SetTextAlign(GUI_TA_HCENTER); 00241 GUI_SetTextMode(GUI_TM_XOR); 00242 GUI_DispStringAt("TEXT MODE XOR", 160, 225); 00243 } 00244 00245 00246 /******************************************************************************* 00247 * Function Name: void ShowTextColors(void) 00248 ******************************************************************************** 00249 * 00250 * Summary: This function displays text in various Colours 00251 * 00252 * Parameters: 00253 * None 00254 * 00255 * Return: 00256 * None 00257 * 00258 *******************************************************************************/ 00259 void ShowTextColors(void) 00260 { 00261 /* Set font size, background Colour and text mode */ 00262 GUI_SetFont(GUI_FONT_16B_1); 00263 GUI_SetBkColor(GUI_BLACK); 00264 GUI_SetTextMode(GUI_TM_NORMAL); 00265 00266 /* Clear the display */ 00267 GUI_Clear(); 00268 00269 /* Display page title */ 00270 GUI_SetTextAlign(GUI_TA_HCENTER); 00271 GUI_SetTextStyle(GUI_TS_UNDERLINE); 00272 GUI_SetColor(GUI_WHITE); 00273 GUI_DispStringAt("2 OF 10: TEXT COLOURS", 160, 10); 00274 GUI_SetTextStyle(GUI_TS_NORMAL); 00275 00276 /* White */ 00277 GUI_SetTextAlign(GUI_TA_HCENTER); 00278 GUI_SetColor(GUI_WHITE); 00279 GUI_DispStringAt("TEXT COLOUR WHITE", 160, 40); 00280 00281 /* Gray */ 00282 GUI_SetTextAlign(GUI_TA_HCENTER); 00283 GUI_SetColor(GUI_GRAY); 00284 GUI_DispStringAt("TEXT COLOUR GRAY", 160, 60); 00285 00286 /* Red */ 00287 GUI_SetTextAlign(GUI_TA_HCENTER); 00288 GUI_SetColor(GUI_RED); 00289 GUI_DispStringAt("TEXT COLOUR RED", 160, 80); 00290 00291 /* Green */ 00292 GUI_SetTextAlign(GUI_TA_HCENTER); 00293 GUI_SetColor(GUI_GREEN); 00294 GUI_DispStringAt("TEXT COLOUR GREEN", 160, 100); 00295 00296 /* Blue */ 00297 GUI_SetTextAlign(GUI_TA_HCENTER); 00298 GUI_SetColor(GUI_BLUE); 00299 GUI_DispStringAt("TEXT COLOUR BLUE", 160, 120); 00300 00301 /* Yellow */ 00302 GUI_SetTextAlign(GUI_TA_HCENTER); 00303 GUI_SetColor(GUI_YELLOW); 00304 GUI_DispStringAt("TEXT COLOUR YELLOW", 160, 140); 00305 00306 /* Brown */ 00307 GUI_SetTextAlign(GUI_TA_HCENTER); 00308 GUI_SetColor(GUI_BROWN); 00309 GUI_DispStringAt("TEXT COLOUR BROWN", 160, 160); 00310 00311 /* Magenta */ 00312 GUI_SetTextAlign(GUI_TA_HCENTER); 00313 GUI_SetColor(GUI_MAGENTA); 00314 GUI_DispStringAt("TEXT COLOUR MAGENTA", 160, 180); 00315 00316 /* Cyan */ 00317 GUI_SetTextAlign(GUI_TA_HCENTER); 00318 GUI_SetColor(GUI_CYAN); 00319 GUI_DispStringAt("TEXT COLOUR CYAN", 160, 200); 00320 00321 /* Orange */ 00322 GUI_SetTextAlign(GUI_TA_HCENTER); 00323 GUI_SetColor(GUI_ORANGE); 00324 GUI_DispStringAt("TEXT COLOUR ORANGE", 160, 220); 00325 } 00326 00327 00328 /******************************************************************************* 00329 * Function Name: void ShowFontSizesNormal(void) 00330 ******************************************************************************** 00331 * 00332 * Summary: This function shows various font sizes 00333 * 00334 * Parameters: 00335 * None 00336 * 00337 * Return: 00338 * None 00339 * 00340 *******************************************************************************/ 00341 void ShowFontSizesNormal(void) 00342 { 00343 /* Set font size, background colour and text mode */ 00344 GUI_SetFont(GUI_FONT_16B_1); 00345 GUI_SetBkColor(GUI_BLACK); 00346 GUI_SetTextMode(GUI_TM_NORMAL); 00347 GUI_SetColor(GUI_GRAY); 00348 00349 /* Clear the display */ 00350 GUI_Clear(); 00351 00352 /* Display page title */ 00353 GUI_SetTextAlign(GUI_TA_HCENTER); 00354 GUI_SetTextStyle(GUI_TS_UNDERLINE); 00355 GUI_DispStringAt("3 OF 10: NORMAL FONTS", 160, 10); 00356 GUI_SetTextStyle(GUI_TS_NORMAL); 00357 00358 /* Font8_1*/ 00359 GUI_SetFont(GUI_FONT_8_1); 00360 GUI_SetBkColor(GUI_BLACK); 00361 GUI_SetColor(GUI_GRAY); 00362 GUI_SetTextMode(GUI_TM_NORMAL); 00363 GUI_DispStringAt("GUI_Font8_1", 10, 40); 00364 00365 /* Font10_1*/ 00366 GUI_SetFont(GUI_FONT_10_1); 00367 GUI_DispStringAt("GUI_Font10_1", 10, 50); 00368 00369 /* Font13_1*/ 00370 GUI_SetFont(GUI_FONT_13_1); 00371 GUI_DispStringAt("GUI_Font13_1", 10, 62); 00372 00373 /* Font16_1*/ 00374 GUI_SetFont(GUI_FONT_16_1); 00375 GUI_DispStringAt("GUI_Font16_1", 10, 77); 00376 00377 /* Font20_1*/ 00378 GUI_SetFont(GUI_FONT_20_1); 00379 GUI_DispStringAt("GUI_Font20_1", 10, 95); 00380 00381 /* Font24_1*/ 00382 GUI_SetFont(GUI_FONT_24_1); 00383 GUI_DispStringAt("GUI_Font24_1", 10, 117); 00384 00385 /* Font32_1*/ 00386 GUI_SetFont(GUI_FONT_32_1); 00387 GUI_DispStringAt("GUI_Font32_1", 10, 143); 00388 00389 /* Font48_1*/ 00390 GUI_SetFont(GUI_FONT_8X16X2X2); 00391 GUI_DispStringAt("GUI_Font8x16x2x2", 10, 180); 00392 } 00393 00394 00395 /******************************************************************************* 00396 * Function Name: void ShowFontSizesBold(void) 00397 ******************************************************************************** 00398 * 00399 * Summary: This function shows various font sizes 00400 * 00401 * Parameters: 00402 * None 00403 * 00404 * Return: 00405 * None 00406 * 00407 *******************************************************************************/ 00408 void ShowFontSizesBold(void) 00409 { 00410 /* Set font size, background colour and text mode */ 00411 GUI_SetFont(GUI_FONT_16B_1); 00412 GUI_SetBkColor(GUI_BLACK); 00413 GUI_SetColor(GUI_GRAY); 00414 GUI_SetTextMode(GUI_TM_NORMAL); 00415 00416 /* Clear the display */ 00417 GUI_Clear(); 00418 00419 /* Display page title */ 00420 GUI_SetTextAlign(GUI_TA_HCENTER); 00421 GUI_SetTextStyle(GUI_TS_UNDERLINE); 00422 GUI_DispStringAt("4 OF 10: BOLD FONTS", 160, 10); 00423 GUI_SetTextStyle(GUI_TS_NORMAL); 00424 00425 /* Font13B_1*/ 00426 GUI_SetFont(GUI_FONT_13B_1); 00427 GUI_DispStringAt("GUI_Font13B_1", 10, 40); 00428 00429 /* Font13HB_1*/ 00430 GUI_SetFont(GUI_FONT_13HB_1); 00431 GUI_DispStringAt("GUI_Font13HB_1", 10, 55); 00432 00433 /* Font16B_1*/ 00434 GUI_SetFont(GUI_FONT_16B_1); 00435 GUI_DispStringAt("GUI_Font6B_1", 10, 70); 00436 00437 /* FontComic18B_1*/ 00438 GUI_SetFont(GUI_FONT_COMIC18B_1); 00439 GUI_DispStringAt("GUI_FontComic18B_1", 10, 88); 00440 00441 /* Font20B_1*/ 00442 GUI_SetFont(GUI_FONT_20B_1); 00443 GUI_DispStringAt("GUI_Font20B_1", 10, 108); 00444 00445 /* Font24B_1*/ 00446 GUI_SetFont(GUI_FONT_24B_1); 00447 GUI_DispStringAt("GUI_Font24B_1", 10, 130); 00448 00449 /* Font32B_1*/ 00450 GUI_SetFont(GUI_FONT_32B_1); 00451 GUI_DispStringAt("GUI_Font32B_1", 10, 156); 00452 00453 /* Font48B_1*/ 00454 GUI_SetFont(GUI_FONT_8X16X3X3); 00455 GUI_DispStringAt("GUI_Font8x16x3x3", 10, 190); 00456 } 00457 00458 00459 /******************************************************************************* 00460 * Function Name: void ShowColorBar(void) 00461 ******************************************************************************** 00462 * 00463 * Summary: This function displays displays horizontal colour bars. For each 00464 * colour, two bars are printed, one bar that has a gradient from 00465 * black to the colour and another bar that has a gradient from white 00466 * to the colour. 00467 * 00468 * Note: This function is provided by EmWin as a demo code. 00469 * 00470 * Parameters: 00471 * None 00472 * 00473 * Return: 00474 * None 00475 * 00476 *******************************************************************************/ 00477 void ShowColorBar(void) 00478 { 00479 /* Local variables */ 00480 int x0; 00481 int y0; 00482 int yStep; 00483 int i; 00484 int xsize; 00485 U16 cs; 00486 U16 x; 00487 00488 /* Initialize parameters */ 00489 x0 = 60; 00490 y0 = 40; 00491 yStep = 15; 00492 xsize = LCD_GetDevCap(LCD_DEVCAP_XSIZE) - x0; 00493 00494 /* Clear the screen */ 00495 GUI_Clear(); 00496 00497 /* Display page title */ 00498 GUI_SetFont(GUI_FONT_16B_1); 00499 GUI_SetColor(GUI_WHITE); 00500 GUI_SetBkColor(GUI_BLACK); 00501 GUI_SetTextMode(GUI_TM_NORMAL); 00502 GUI_SetTextAlign(GUI_TA_HCENTER); 00503 GUI_SetTextStyle(GUI_TS_UNDERLINE); 00504 GUI_DispStringAt("6 OF 10: COLOUR BARS", 160, 5); 00505 00506 /* Set text mode to normal and left alignment */ 00507 GUI_SetTextMode(GUI_TM_NORMAL); 00508 GUI_SetTextAlign(GUI_TA_LEFT); 00509 GUI_SetTextStyle(GUI_TS_NORMAL); 00510 00511 /* Display labels for the bars */ 00512 GUI_SetFont(&GUI_Font8x16); 00513 GUI_SetColor(GUI_WHITE); 00514 GUI_SetBkColor(GUI_BLACK); 00515 GUI_DispStringAt("Red", 0, y0 + yStep); 00516 GUI_DispStringAt("Green", 0, y0 + 3 * yStep); 00517 GUI_DispStringAt("Blue", 0, y0 + 5 * yStep); 00518 GUI_DispStringAt("Yellow", 0, y0 + 7 * yStep); 00519 GUI_DispStringAt("Cyan", 0, y0 + 9 * yStep); 00520 GUI_DispStringAt("Magenta", 0, y0 + 11 * yStep); 00521 00522 /* Draw the colour bars */ 00523 for (i = 0; i < xsize; i++) 00524 { 00525 cs = (0xFF * (U32)i) / xsize; 00526 x = x0 + i;; 00527 00528 /* Red */ 00529 GUI_SetColor(cs); 00530 GUI_DrawVLine(x, y0 , y0 + yStep - 1); 00531 GUI_SetColor(0xff + (255 - cs) * 0x10100uL); 00532 GUI_DrawVLine(x, y0 + yStep, y0 + 2 * yStep - 1); 00533 00534 /* Green */ 00535 GUI_SetColor(cs<<8); 00536 GUI_DrawVLine(x, y0 + 2 * yStep, y0 + 3 * yStep - 1); 00537 GUI_SetColor(0xff00 + (255 - cs) * 0x10001uL); 00538 GUI_DrawVLine(x, y0 + 3 * yStep, y0 + 4 * yStep - 1); 00539 00540 /* Blue */ 00541 GUI_SetColor(cs * 0x10000uL); 00542 GUI_DrawVLine(x, y0 + 4 * yStep, y0 + 5 * yStep - 1); 00543 GUI_SetColor(0xff0000 + (255 - cs) * 0x101uL); 00544 GUI_DrawVLine(x, y0 + 5 * yStep, y0 + 6 * yStep - 1); 00545 00546 /* Yellow */ 00547 GUI_SetColor(cs * 0x101uL); 00548 GUI_DrawVLine(x, y0 + 6 * yStep, y0 + 7 * yStep - 1); 00549 GUI_SetColor(0xffff + (255 - cs) * 0x10000uL); 00550 GUI_DrawVLine(x, y0 + 7 * yStep, y0 + 8 * yStep - 1); 00551 00552 /* Cyan */ 00553 GUI_SetColor(cs * 0x10100uL); 00554 GUI_DrawVLine(x, y0 + 8 * yStep, y0 + 9 * yStep - 1); 00555 GUI_SetColor(0xffff00 + (255 - cs) * 0x1L); 00556 GUI_DrawVLine(x, y0 + 9 * yStep, y0 + 10 * yStep - 1); 00557 00558 /* Magenta */ 00559 GUI_SetColor(cs * 0x10001uL); 00560 GUI_DrawVLine(x, y0 + 10 * yStep, y0 + 11 * yStep - 1); 00561 GUI_SetColor(0xff00ff + (255 - cs) * 0x100uL); 00562 GUI_DrawVLine(x, y0 + 11 * yStep, y0 + 12 * yStep - 1); 00563 } 00564 } 00565 00566 00567 /******************************************************************************* 00568 * Function Name: void Show2DGraphics1(void) 00569 ******************************************************************************** 00570 * 00571 * Summary: This function displays the following 2D graphics 00572 * 1. Horizontal lines with various pen widths 00573 * 2. Vertical lines with various pen widths 00574 * 3. Arcs 00575 * 4. Filled rounded rectangle 00576 * 00577 * Parameters: 00578 * None 00579 * 00580 * Return: 00581 * None 00582 * 00583 *******************************************************************************/ 00584 void Show2DGraphics1(void) 00585 { 00586 /* Set font size, foreground and background colours */ 00587 GUI_SetFont(GUI_FONT_16B_1); 00588 GUI_SetColor(GUI_WHITE); 00589 GUI_SetBkColor(GUI_BLACK); 00590 GUI_SetTextMode(GUI_TM_NORMAL); 00591 GUI_SetTextStyle(GUI_TS_NORMAL); 00592 00593 /* Clear the screen */ 00594 GUI_Clear(); 00595 00596 /* Display page title */ 00597 GUI_SetTextAlign(GUI_TA_HCENTER); 00598 GUI_SetTextStyle(GUI_TS_UNDERLINE); 00599 GUI_DispStringAt("7 OF 10: 2D GRAPHICS - 1", 160, 10); 00600 GUI_SetTextStyle(GUI_TS_NORMAL); 00601 00602 /* Set drawing colour to gray */ 00603 GUI_SetColor(GUI_GRAY); 00604 00605 /* Display labels */ 00606 GUI_SetTextAlign(GUI_TA_HCENTER); 00607 GUI_DispStringAt("H-LINES", 80, 110); 00608 GUI_SetTextAlign(GUI_TA_HCENTER); 00609 GUI_DispStringAt("V-LINES", 240, 110); 00610 GUI_SetTextAlign(GUI_TA_HCENTER); 00611 GUI_DispStringAt("ARCS", 80, 220); 00612 GUI_SetTextAlign(GUI_TA_HCENTER); 00613 GUI_DispStringAt("ROUNDED RECT", 240, 220); 00614 00615 /* Horizontal lines */ 00616 GUI_SetPenSize(1); 00617 GUI_DrawLine(10, 35, 150, 35); 00618 GUI_SetPenSize(2); 00619 GUI_DrawLine(10, 50, 150, 50); 00620 GUI_SetPenSize(3); 00621 GUI_DrawLine(10, 65, 150, 65); 00622 GUI_SetPenSize(4); 00623 GUI_DrawLine(10, 80, 150, 80); 00624 GUI_SetPenSize(5); 00625 GUI_DrawLine(10, 95, 150, 95); 00626 00627 /* Vertical lines */ 00628 GUI_SetPenSize(1); 00629 GUI_DrawLine(210, 30, 210, 100); 00630 GUI_SetPenSize(2); 00631 GUI_DrawLine(225, 30, 225, 100); 00632 GUI_SetPenSize(3); 00633 GUI_DrawLine(240, 30, 240, 100); 00634 GUI_SetPenSize(4); 00635 GUI_DrawLine(255, 30, 255, 100); 00636 GUI_SetPenSize(5); 00637 GUI_DrawLine(270, 30, 270, 100); 00638 00639 /* Arcs */ 00640 GUI_SetPenSize(2); 00641 GUI_DrawArc(80, 210, 10, 10, 0, 180); 00642 GUI_DrawArc(80, 210, 20, 20, 0, 180); 00643 GUI_DrawArc(80, 210, 30, 30, 0, 180); 00644 GUI_DrawArc(80, 210, 40, 40, 0, 180); 00645 GUI_DrawArc(80, 210, 50, 50, 0, 180); 00646 GUI_DrawArc(80, 210, 60, 60, 0, 180); 00647 00648 /* Rounded rectangle */ 00649 GUI_FillRoundedRect(180, 150, 300, 210, 5); 00650 } 00651 00652 00653 /******************************************************************************* 00654 * Function Name: void Show2DGraphics2(void) 00655 ******************************************************************************** 00656 * 00657 * Summary: This function displays the following 2D graphics 00658 * 1. Concentric circles 00659 * 2. Concentric ellipses 00660 * 3. Filled rectangle with horizontal colour gradient 00661 * 4. Filled rectangle with vertical colour gradient 00662 * 00663 * Parameters: 00664 * None 00665 * 00666 * Return: 00667 * None 00668 * 00669 *******************************************************************************/ 00670 void Show2DGraphics2(void) 00671 { 00672 /* Set font size, foreground and background colours */ 00673 GUI_SetFont(GUI_FONT_16B_1); 00674 GUI_SetColor(GUI_WHITE); 00675 GUI_SetBkColor(GUI_BLACK); 00676 GUI_SetTextMode(GUI_TM_NORMAL); 00677 GUI_SetTextStyle(GUI_TS_NORMAL); 00678 00679 /* Clear the screen */ 00680 GUI_Clear(); 00681 00682 /* Display page title */ 00683 GUI_SetTextAlign(GUI_TA_HCENTER); 00684 GUI_SetTextStyle(GUI_TS_UNDERLINE); 00685 GUI_DispStringAt("8 OF 10: 2D GRAPHICS - 2", 160, 10); 00686 GUI_SetTextStyle(GUI_TS_NORMAL); 00687 00688 /* Set drawing colour to gray */ 00689 GUI_SetColor(GUI_GRAY); 00690 00691 /* Display labels */ 00692 GUI_SetTextAlign(GUI_TA_HCENTER); 00693 GUI_DispStringAt("CIRCLE", 80, 110); 00694 GUI_SetTextAlign(GUI_TA_HCENTER); 00695 GUI_DispStringAt("ELLIPSE", 240, 110); 00696 GUI_SetTextAlign(GUI_TA_HCENTER); 00697 GUI_DispStringAt("RECT-HGRAD", 80, 220); 00698 GUI_SetTextAlign(GUI_TA_HCENTER); 00699 GUI_DispStringAt("RECT-VGRAD", 240, 220); 00700 00701 /* Concentric Circles */ 00702 GUI_DrawCircle(80, 65, 35); 00703 GUI_DrawCircle(80, 65, 25); 00704 GUI_DrawCircle(80, 65, 15); 00705 GUI_DrawCircle(80, 65, 5); 00706 00707 /* Concentric Ellipses */ 00708 GUI_DrawEllipse(240, 65, 60, 35); 00709 GUI_DrawEllipse(240, 65, 50, 25); 00710 GUI_DrawEllipse(240, 65, 40, 15); 00711 GUI_DrawEllipse(240, 65, 30, 5); 00712 00713 /* Rectangle horizontal gradient */ 00714 GUI_DrawGradientH(20, 150, 140, 210, GUI_BLACK, GUI_GREEN); 00715 00716 /* Rectangle vertical gradient */ 00717 GUI_DrawGradientV(180, 150, 300, 210, GUI_RED, GUI_BLACK); 00718 } 00719 00720 00721 /******************************************************************************* 00722 * Function Name: void ShowConcentricCircles(void) 00723 ******************************************************************************** 00724 * 00725 * Summary: This function displays a short animation by drawing concentric circles 00726 * with colour gradients 00727 * 00728 * Parameters: 00729 * None 00730 * 00731 * Return: 00732 * None 00733 * 00734 *******************************************************************************/ 00735 void ShowConcentricCircles(void) 00736 { 00737 #define NUMBER_OF_COLOURS (3u) 00738 #define MAX_RADIUS (100u) 00739 00740 uint16 radius = 0; 00741 uint16 colourIndex = 0; 00742 00743 const uint32 colourArray[NUMBER_OF_COLOURS] = 00744 { 00745 GUI_RED, 00746 GUI_BLUE, 00747 GUI_GREEN, 00748 }; 00749 00750 /* Set font size, background colour and text mode */ 00751 GUI_SetFont(GUI_FONT_16B_1); 00752 GUI_SetBkColor(GUI_BLACK); 00753 GUI_SetTextMode(GUI_TM_NORMAL); 00754 00755 /* Clear the display */ 00756 GUI_Clear(); 00757 00758 /* Display page title */ 00759 GUI_SetTextAlign(GUI_TA_HCENTER); 00760 GUI_SetTextStyle(GUI_TS_UNDERLINE); 00761 GUI_SetColor(GUI_WHITE); 00762 GUI_DispStringAt("9 OF 10: CONCENTRIC CIRCLES", 160, 10); 00763 GUI_SetTextStyle(GUI_TS_NORMAL); 00764 00765 /* Draw concentric circles with colour gradient */ 00766 /* Cycle through number of colours */ 00767 for(colourIndex = 0; colourIndex < NUMBER_OF_COLOURS; colourIndex++) 00768 { 00769 /* Draw circles with increasing radius */ 00770 for(radius = 0; radius < MAX_RADIUS; radius++) 00771 { 00772 GUI_SetColor(radius*colourArray[colourIndex]); 00773 GUI_DrawCircle(160, 130, radius); 00774 CyDelay(2); 00775 } 00776 } 00777 } 00778 00779 00780 /******************************************************************************* 00781 * Function Name: void ShowBitmap(void) 00782 ******************************************************************************** 00783 * 00784 * Summary: This function displays a bitmap image with an overlay text 00785 * 00786 * Parameters: 00787 * None 00788 * 00789 * Return: 00790 * None 00791 * 00792 *******************************************************************************/ 00793 void ShowTextWrapAndOrientation(void) 00794 { 00795 GUI_RECT leftRect = {4, 19, 24, 166}; 00796 GUI_RECT rightRect = {238, 19, 258, 166}; 00797 GUI_RECT middleRect = {29, 19, 233, 166}; 00798 GUI_RECT middleRectMargins = {31, 20, 232, 165}; 00799 GUI_RECT bottomRect = {31, 170, 232, 220}; 00800 00801 const char leftText[] = "ROTATED TEXT CCW"; 00802 const char rightText[] = "ROTATED TEXT CW"; 00803 const char bottomText[] = "INVERTED TEXT 180"; 00804 00805 const char middleText[] = "This project demonstrates displaying 2D graphics in a TFT display using Segger EmWin Graphics Library. \n\nThis page shows the text wrap and text rotation features. In the left rectangle, the text is rotated counter clockwise and in the right rectangle, the text is rotated clockwise."; 00806 00807 00808 /* Set font size, foreground and background colours */ 00809 GUI_SetFont(GUI_FONT_13B_1); 00810 GUI_SetColor(GUI_WHITE); 00811 GUI_SetBkColor(GUI_BLACK); 00812 GUI_SetTextMode(GUI_TM_NORMAL); 00813 GUI_SetTextStyle(GUI_TS_NORMAL); 00814 00815 /* Clear the screen */ 00816 GUI_Clear(); 00817 00818 /* Display page title */ 00819 GUI_SetTextAlign(GUI_TA_HCENTER); 00820 GUI_DispStringAt("5 OF 10: TEXT WRAP AND ROTATION", 132, 5); 00821 00822 /* Draw rectangles to hold text */ 00823 GUI_DrawRectEx(&leftRect); 00824 GUI_DrawRectEx(&rightRect); 00825 GUI_DrawRectEx(&middleRect); 00826 GUI_DrawRectEx(&bottomRect); 00827 00828 /* Display string in left rectangle rotated counter clockwise */ 00829 GUI_DispStringInRectEx(leftText, &leftRect, GUI_TA_HCENTER | GUI_TA_VCENTER, strlen(leftText), GUI_ROTATE_CCW); 00830 00831 /* Display string in right rectangle rotated clockwise */ 00832 GUI_DispStringInRectEx(rightText, &rightRect, GUI_TA_HCENTER | GUI_TA_VCENTER, strlen(rightText), GUI_ROTATE_CW); 00833 00834 /* Display string in right rectangle rotated clockwise */ 00835 GUI_DispStringInRectEx(bottomText, &bottomRect, GUI_TA_HCENTER | GUI_TA_VCENTER, strlen(bottomText), GUI_ROTATE_180); 00836 00837 /* Display string in middle rectangle with word wrap */ 00838 GUI_DispStringInRectWrap(middleText, &middleRectMargins, GUI_TA_LEFT, GUI_WRAPMODE_WORD); 00839 00840 } 00841 void ShowBitmap(void) 00842 { 00843 /* Set background colour to black and clear screen */ 00844 GUI_SetBkColor(GUI_BLACK); 00845 GUI_Clear(); 00846 00847 /* Display the bitmap image on the screen */ 00848 GUI_DrawBitmap(&bmExampleImage, 0, 4); 00849 00850 /* Set font size, font colour to black */ 00851 GUI_SetFont(GUI_FONT_16B_1); 00852 GUI_SetColor(GUI_BLACK); 00853 00854 /* Set text mode to transparent, underlined and center aligned */ 00855 GUI_SetTextMode(GUI_TM_TRANS); 00856 GUI_SetTextAlign(GUI_TA_HCENTER); 00857 GUI_SetTextStyle(GUI_TS_UNDERLINE); 00858 00859 /* Print the page title text */ 00860 GUI_DispStringAt("10 OF 10: BITMAP IMAGE", 160, 10); 00861 } 00862 00863 00864 /******************************************************************************* 00865 * Function Name: bool IsBtnClicked 00866 ******************************************************************************** 00867 * 00868 * Summary: This non-blocking function implements SW2 button click check. 00869 * 00870 * Parameters: 00871 * None 00872 * 00873 * Return: 00874 * Status of the SW2 button: 00875 * true when button was pressed and then released and 00876 * false in other cases 00877 * 00878 *******************************************************************************/ 00879 bool IsBtnClicked(void) 00880 { 00881 int currBtnState; 00882 static int prevBtnState = BTN_RELEASED; 00883 00884 bool result = false; 00885 00886 currBtnState = sw2; 00887 00888 if((prevBtnState == BTN_RELEASED) && (currBtnState == BTN_PRESSED)) 00889 { 00890 result = true; 00891 } 00892 00893 prevBtnState = currBtnState; 00894 00895 wait_ms(5); 00896 00897 return result; 00898 } 00899 00900 00901 /******************************************************************************* 00902 * Function Name: int main(void) 00903 ******************************************************************************** 00904 * 00905 * Summary: This is the main for this code example. This function does the following 00906 * 1. Initializes the EmWin display engine 00907 * 2. Displays startup screen for 3 seconds 00908 * 3. In an infinite loop, displays the following screens on 00909 * key press and release 00910 * a. Text alignment, styles and modes 00911 * b. Text colour 00912 * c. Normal fonts 00913 * d. Bold fonts 00914 * e. colour bars 00915 * f. 2D graphics #1 00916 * g. 2D graphics #2 00917 * h. Concentric circles 00918 * i. Bitmap image 00919 * 00920 * Parameters: 00921 * None 00922 * 00923 * Return: 00924 * None 00925 * 00926 *******************************************************************************/ 00927 int main(void) 00928 { 00929 uint8_t pageNumber = 0; 00930 00931 /* Turn off both red and green LEDs */ 00932 ledGreen = LED_OFF; 00933 ledRed = LED_OFF; 00934 ledBlue = LED_ON; 00935 00936 /* Initialize EmWin driver*/ 00937 GUI_Init(); 00938 00939 /* Display the startup screen for 2 seconds */ 00940 ShowStartupScreen(); 00941 Cy_SysLib_Delay(2000); 00942 00943 /* Show Instructions Screen */ 00944 ShowInstructionsScreen(); 00945 00946 /* Display various demo pages in a loop */ 00947 for(;;) 00948 { 00949 if(IsBtnClicked()) 00950 { 00951 /* Using pageNumber as index, update the display with a demo screen 00952 Following are the functions that are called in sequence 00953 ShowTextModes() 00954 ShowTextColors() 00955 ShowFontSizesNormal() 00956 ShowFontSizesBold() 00957 ShowTextWrapAndOrientation() 00958 ShowColorBar() 00959 Show2DGraphics1() 00960 Show2DGraphics2() 00961 ShowConcentricCircles() 00962 ShowBitmap() 00963 */ 00964 (*demoPageArray[pageNumber])(); 00965 00966 ledBlue = !ledBlue; 00967 00968 /* Increment page number */ 00969 pageNumber++; 00970 00971 /* If page number exceeds maximum pages, reset */ 00972 if(pageNumber >= NUMBER_OF_DEMO_PAGES) 00973 { 00974 pageNumber = 0; 00975 } 00976 } 00977 } 00978 } 00979 00980 00981 /* [] END OF FILE */
Generated on Wed Jul 13 2022 05:29:37 by
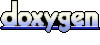