An example program that uses FRDM-TFC library written by Eli Hughes.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "TFC.h" 00003 00004 00005 //This macro is to maintain compatibility with Codewarrior version of the sample. This version uses the MBED libraries for serial port access 00006 Serial PC(USBTX,USBRX); 00007 00008 #define TERMINAL_PRINTF PC.printf 00009 00010 00011 //This ticker code is used to maintain compability with the Codewarrior version of the sample. This code uses an MBED Ticker for background timing. 00012 00013 #define NUM_TFC_TICKERS 4 00014 00015 Ticker TFC_TickerObj; 00016 00017 volatile uint32_t TFC_Ticker[NUM_TFC_TICKERS]; 00018 00019 void TFC_TickerUpdate() 00020 { 00021 int i; 00022 00023 for(i=0; i<NUM_TFC_TICKERS; i++) 00024 { 00025 if(TFC_Ticker[i]<0xFFFFFFFF) 00026 { 00027 TFC_Ticker[i]++; 00028 } 00029 } 00030 } 00031 00032 00033 00034 00035 int main() 00036 { 00037 uint32_t i,t = 0; 00038 00039 PC.baud(115200); 00040 TFC_TickerObj.attach_us(&TFC_TickerUpdate,2000); 00041 00042 TFC_Init(); 00043 00044 for(;;) 00045 { 00046 //TFC_Task must be called in your main loop. This keeps certain processing happy (I.E. Serial port queue check) 00047 // TFC_Task(); 00048 00049 //This Demo program will look at the middle 2 switch to select one of 4 demo modes. 00050 //Let's look at the middle 2 switches 00051 switch((TFC_GetDIP_Switch()>>1)&0x03) 00052 { 00053 default: 00054 case 0 : 00055 //Demo mode 0 just tests the switches and LED's 00056 if(TFC_PUSH_BUTTON_0_PRESSED) 00057 TFC_BAT_LED0_ON; 00058 else 00059 TFC_BAT_LED0_OFF; 00060 00061 if(TFC_PUSH_BUTTON_1_PRESSED) 00062 TFC_BAT_LED3_ON; 00063 else 00064 TFC_BAT_LED3_OFF; 00065 00066 00067 if(TFC_GetDIP_Switch()&0x01) 00068 TFC_BAT_LED1_ON; 00069 else 00070 TFC_BAT_LED1_OFF; 00071 00072 if(TFC_GetDIP_Switch()&0x08) 00073 TFC_BAT_LED2_ON; 00074 else 00075 TFC_BAT_LED2_OFF; 00076 00077 break; 00078 00079 case 1: 00080 00081 //Demo mode 1 will just move the servos with the on-board potentiometers 00082 if(TFC_Ticker[0]>=20) 00083 { 00084 TFC_Ticker[0] = 0; //reset the Ticker 00085 //Every 20 mSeconds, update the Servos 00086 TFC_SetServo(0,TFC_ReadPot(0)); 00087 TFC_SetServo(1,TFC_ReadPot(1)); 00088 } 00089 //Let's put a pattern on the LEDs 00090 if(TFC_Ticker[1] >= 125) 00091 { 00092 TFC_Ticker[1] = 0; 00093 t++; 00094 if(t>4) 00095 { 00096 t=0; 00097 } 00098 TFC_SetBatteryLED_Level(t); 00099 } 00100 00101 TFC_SetMotorPWM(0,0); //Make sure motors are off 00102 TFC_HBRIDGE_DISABLE; 00103 00104 00105 break; 00106 00107 case 2 : 00108 00109 //Demo Mode 2 will use the Pots to make the motors move 00110 TFC_HBRIDGE_ENABLE; 00111 00112 TFC_SetMotorPWM(TFC_ReadPot(0),TFC_ReadPot(1)); 00113 00114 00115 //Let's put a pattern on the LEDs 00116 if(TFC_Ticker[1] >= 125) 00117 { 00118 TFC_Ticker[1] = 0; 00119 t++; 00120 if(t>4) 00121 { 00122 t=0; 00123 } 00124 TFC_SetBatteryLED_Level(t); 00125 } 00126 break; 00127 00128 case 3 : 00129 00130 00131 //Demo Mode 3 will be in Freescale Garage Mode. It will beam data from the Camera to the 00132 //Labview Application 00133 00134 00135 if(TFC_Ticker[0]>50 && TFC_LineScanImageReady>0) 00136 { 00137 TFC_Ticker[0] = 0; 00138 TFC_LineScanImageReady=0; 00139 TERMINAL_PRINTF("\r\n"); 00140 TERMINAL_PRINTF("L:"); 00141 00142 if(t==0) 00143 t=4; 00144 else 00145 t--; 00146 00147 TFC_SetBatteryLED_Level(t); 00148 00149 // camera 1 00150 for(i=0;i<128;i++) 00151 { 00152 TERMINAL_PRINTF("%X,",TFC_LineScanImage0[i]); 00153 } 00154 00155 // camera 2 00156 for(i=0;i<128;i++) 00157 { 00158 if(i==127) 00159 TERMINAL_PRINTF("%X\r\n",TFC_LineScanImage1[i]); 00160 else 00161 TERMINAL_PRINTF("%X,",TFC_LineScanImage1[i]); 00162 00163 } 00164 } 00165 00166 break; 00167 } 00168 } 00169 00170 00171 } 00172
Generated on Mon Jul 18 2022 22:01:59 by
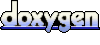