
BlinkButton2Sec done
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 DigitalOut led1(LED1); 00004 InterruptIn button(USER_BUTTON); 00005 EventQueue queue(32 * EVENTS_EVENT_SIZE); 00006 Thread t; 00007 time_t seconds = time(NULL); 00008 00009 void rise_handler_thread_context(void) { 00010 if(seconds - time(NULL) > 2){ 00011 seconds = time(NULL); 00012 } 00013 else { 00014 led1 = !led1; 00015 wait(1); 00016 led1 = !led1; 00017 wait(1); 00018 led1 = !led1; 00019 wait(1); 00020 led1 = !led1; 00021 seconds = time(NULL); 00022 } 00023 } 00024 00025 void rise_handler_iterrupt_context(void) { 00026 queue.call(rise_handler_thread_context); 00027 } 00028 00029 void fall_handler(void) { 00030 printf("hello\n"); 00031 } 00032 00033 int main() { 00034 // Start the event queue 00035 t.start(callback(&queue, &EventQueue::dispatch_forever)); 00036 // The 'rise' handler will execute in IRQ context 00037 button.rise(rise_handler_iterrupt_context); 00038 // The 'fall' handler will execute in the context of thread 't' 00039 button.fall(queue.event(fall_handler)); 00040 }
Generated on Thu Jul 28 2022 03:04:04 by
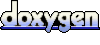