
First Version
Dependencies: EthernetInterface mbed-rtos mbed
dma.c
00001 /* 00002 * dma.c 00003 * 00004 * Created on: 03/07/2011 00005 * Author: francisco 00006 */ 00007 #include <LPC17xx.h> 00008 #include "dma.h" 00009 00010 #define LPC_GPDMACH ((LPC_GPDMACH_TypeDef **) LPC_GPDMACH0_BASE ) 00011 00012 void init_dma() 00013 { 00014 LPC_SC->PCONP |= 1<<29; //Power GPDMA module 00015 00016 LPC_GPDMA->DMACConfig = 1; //Enable GPDMA 00017 00018 //Clear any previous interrupts 00019 LPC_GPDMA->DMACIntTCClear = 0xFF; 00020 LPC_GPDMA->DMACIntErrClr = 0xFF; 00021 00022 NVIC_SetPriority(DMA_IRQn,(1<<__NVIC_PRIO_BITS) - 1); 00023 NVIC_EnableIRQ(DMA_IRQn); 00024 } 00025 00026 void setup_channel(dmaLinkedListNode* pList,int ch,int src,int dst) 00027 { 00028 //Initialize the channel with previously configured LL; 00029 LPC_GPDMACH0->DMACCSrcAddr = pList->sourceAddr; 00030 LPC_GPDMACH0->DMACCDestAddr = pList->destAddr; 00031 LPC_GPDMACH0->DMACCControl = pList->dmaControl; 00032 LPC_GPDMACH0->DMACCLLI = (unsigned long int) pList & 0xFFFFFFFC; //Lower bits must be 0 00033 00034 int transfer_type; 00035 if(src == DMA_MEMORY && dst != DMA_MEMORY) 00036 { 00037 transfer_type = DMA_MEMORY_TO_PERIPHERAL; 00038 src = 0; 00039 } 00040 else if(src != DMA_MEMORY && dst == DMA_MEMORY) 00041 { 00042 transfer_type = DMA_PERIPHERAL_TO_MEMORY; 00043 dst = 0; 00044 } 00045 else if(src == DMA_MEMORY && dst == DMA_MEMORY) 00046 { 00047 transfer_type = DMA_MEMORY_TO_MEMORY; 00048 src=dst = 0; 00049 } 00050 else if(src != DMA_MEMORY && dst != DMA_MEMORY) 00051 transfer_type = DMA_PERIPHERAL_TO_PERIPHERAL; 00052 00053 //Set up all relevant bits 00054 LPC_GPDMACH0->DMACCConfig = (src<<1) | (dst<<5) | (transfer_type<<11) | (1<<15); 00055 00056 //Finally, enable the channel - 00057 LPC_GPDMACH0->DMACCConfig |= 1<<0; 00058 } 00059 00060 void stop_channel() 00061 { 00062 LPC_GPDMACH0->DMACCConfig &= ~(1<<0); 00063 }
Generated on Thu Jul 21 2022 16:56:17 by
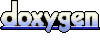