Extended MaximInterface
Dependents: mbed_DS28EC20_GPIO
array.hpp
00001 /******************************************************************************* 00002 * Copyright (C) 2017 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 *******************************************************************************/ 00032 00033 #ifndef MaximInterface_array 00034 #define MaximInterface_array 00035 00036 #include <stddef.h> 00037 #include <stdint.h> 00038 #include <algorithm> 00039 #include <iterator> 00040 00041 namespace MaximInterface { 00042 00043 /// Generic array class similar to std::array. 00044 template <typename T, size_t N> class array { 00045 public: 00046 typedef T value_type; 00047 typedef size_t size_type; 00048 typedef ptrdiff_t difference_type; 00049 typedef value_type & reference; 00050 typedef const value_type & const_reference; 00051 typedef value_type * pointer; 00052 typedef const value_type * const_pointer; 00053 typedef pointer iterator; 00054 typedef const_pointer const_iterator; 00055 typedef std::reverse_iterator<iterator> reverse_iterator; 00056 typedef std::reverse_iterator<const_iterator> const_reverse_iterator; 00057 00058 /// @name Element access 00059 /// @{ 00060 00061 reference operator[](size_type pos) { 00062 return const_cast<reference>( 00063 static_cast<const array &>(*this).operator[](pos)); 00064 } 00065 00066 const_reference operator[](size_type pos) const { return data()[pos]; } 00067 00068 reference front() { 00069 return const_cast<reference>(static_cast<const array &>(*this).front()); 00070 } 00071 00072 const_reference front() const { return operator[](0); } 00073 00074 reference back() { 00075 return const_cast<reference>(static_cast<const array &>(*this).back()); 00076 } 00077 00078 const_reference back() const { return operator[](size() - 1); } 00079 00080 pointer data() { 00081 return const_cast<pointer>(static_cast<const array &>(*this).data()); 00082 } 00083 00084 const_pointer data() const { return _buffer; } 00085 00086 /// @} 00087 00088 /// @name Iterators 00089 /// @{ 00090 00091 iterator begin() { 00092 return const_cast<iterator>(static_cast<const array &>(*this).cbegin()); 00093 } 00094 00095 const_iterator begin() const { return cbegin(); } 00096 00097 const_iterator cbegin() const { return data(); } 00098 00099 iterator end() { 00100 return const_cast<iterator>(static_cast<const array &>(*this).cend()); 00101 } 00102 00103 const_iterator end() const { return cend(); } 00104 00105 const_iterator cend() const { return cbegin() + size(); } 00106 00107 reverse_iterator rbegin() { return reverse_iterator(end()); } 00108 00109 const_reverse_iterator rbegin() const { 00110 return const_reverse_iterator(end()); 00111 } 00112 00113 const_reverse_iterator crbegin() const { return rbegin(); } 00114 00115 reverse_iterator rend() { return reverse_iterator(begin()); } 00116 00117 const_reverse_iterator rend() const { 00118 return const_reverse_iterator(begin()); 00119 } 00120 00121 const_reverse_iterator crend() const { return rend(); } 00122 00123 /// @} 00124 00125 /// @name Capacity 00126 /// @{ 00127 00128 static bool empty() { return size() == 0; } 00129 00130 static size_type size() { return N; } 00131 00132 static size_type max_size() { return size(); } 00133 00134 /// Alternative to size() when a constant expression is required. 00135 static const size_type csize = N; 00136 00137 /// @} 00138 00139 /// @name Operations 00140 /// @{ 00141 00142 void fill(const_reference value) { std::fill(begin(), end(), value); } 00143 00144 void swap(array & other) { std::swap_ranges(begin(), end(), other.begin()); } 00145 00146 /// @} 00147 00148 /// @private 00149 /// @note Implementation detail set public to allow aggregate initialization. 00150 T _buffer[N]; 00151 }; 00152 00153 template <typename T, size_t N> 00154 inline bool operator==(const array<T, N> & lhs, const array<T, N> & rhs) { 00155 return std::equal(lhs.begin(), lhs.end(), rhs.begin()); 00156 } 00157 00158 template <typename T, size_t N> 00159 inline bool operator!=(const array<T, N> & lhs, const array<T, N> & rhs) { 00160 return !operator==(lhs, rhs); 00161 } 00162 00163 template <typename T, size_t N> 00164 inline bool operator<(const array<T, N> & lhs, const array<T, N> & rhs) { 00165 return std::lexicographical_compare(lhs.begin(), lhs.end(), rhs.begin(), 00166 rhs.end()); 00167 } 00168 00169 template <typename T, size_t N> 00170 inline bool operator>(const array<T, N> & lhs, const array<T, N> & rhs) { 00171 return operator<(rhs, lhs); 00172 } 00173 00174 template <typename T, size_t N> 00175 inline bool operator<=(const array<T, N> & lhs, const array<T, N> & rhs) { 00176 return !operator>(lhs, rhs); 00177 } 00178 00179 template <typename T, size_t N> 00180 inline bool operator>=(const array<T, N> & lhs, const array<T, N> & rhs) { 00181 return !operator<(lhs, rhs); 00182 } 00183 00184 template <typename T, size_t N> 00185 inline void swap(array<T, N> & lhs, array<T, N> & rhs) { 00186 lhs.swap(rhs); 00187 } 00188 00189 } // namespace MaximInterface 00190 00191 #endif
Generated on Tue Jul 12 2022 23:29:45 by
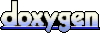