
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
utest_shim.cpp
00001 /**************************************************************************** 00002 * Copyright (c) 2015, ARM Limited, All Rights Reserved 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00006 * not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 **************************************************************************** 00017 */ 00018 00019 #include "utest/utest_shim.h" 00020 #include "utest/utest_stack_trace.h" 00021 00022 #if UTEST_SHIM_SCHEDULER_USE_MINAR 00023 #include "minar/minar.h" 00024 00025 static int32_t utest_minar_init() 00026 { 00027 return 0; 00028 } 00029 static void *utest_minar_post(const utest_v1_harness_callback_t callback, const uint32_t delay_ms) 00030 { 00031 void *handle = minar::Scheduler::postCallback(callback).delay(minar::milliseconds(delay_ms)).getHandle(); 00032 return handle; 00033 } 00034 static int32_t utest_minar_cancel(void *handle) 00035 { 00036 int32_t ret = minar::Scheduler::cancelCallback(handle); 00037 return ret; 00038 } 00039 static int32_t utest_minar_run() 00040 { 00041 return 0; 00042 } 00043 extern "C" { 00044 static const utest_v1_scheduler_t utest_v1_scheduler = 00045 { 00046 utest_minar_init, 00047 utest_minar_post, 00048 utest_minar_cancel, 00049 utest_minar_run 00050 }; 00051 utest_v1_scheduler_t utest_v1_get_scheduler() 00052 { 00053 return utest_v1_scheduler; 00054 } 00055 } 00056 00057 #elif UTEST_SHIM_SCHEDULER_USE_US_TICKER 00058 #ifdef YOTTA_MBED_HAL_VERSION_STRING 00059 # include "mbed-hal/us_ticker_api.h" 00060 #else 00061 # include "mbed.h" 00062 #endif 00063 00064 // only one callback is active at any given time 00065 static volatile utest_v1_harness_callback_t minimal_callback; 00066 static volatile utest_v1_harness_callback_t ticker_callback; 00067 00068 // Timeout object used to control the scheduling of test case callbacks 00069 SingletonPtr<Timeout> utest_timeout_object; 00070 00071 static void ticker_handler() 00072 { 00073 UTEST_LOG_FUNCTION(); 00074 minimal_callback = ticker_callback; 00075 } 00076 00077 static int32_t utest_us_ticker_init() 00078 { 00079 UTEST_LOG_FUNCTION(); 00080 // initialize the Timeout object to makes sure it is not initialized in 00081 // interrupt context. 00082 utest_timeout_object.get(); 00083 return 0; 00084 } 00085 static void *utest_us_ticker_post(const utest_v1_harness_callback_t callback, timestamp_t delay_ms) 00086 { 00087 UTEST_LOG_FUNCTION(); 00088 timestamp_t delay_us = delay_ms *1000; 00089 00090 if (delay_ms) { 00091 ticker_callback = callback; 00092 // fire the interrupt in 1000us * delay_ms 00093 utest_timeout_object->attach_us(ticker_handler, delay_us); 00094 00095 } 00096 else { 00097 minimal_callback = callback; 00098 } 00099 00100 // return a bogus handle 00101 return (void*)1; 00102 } 00103 static int32_t utest_us_ticker_cancel(void *handle) 00104 { 00105 UTEST_LOG_FUNCTION(); 00106 (void) handle; 00107 utest_timeout_object->detach(); 00108 return 0; 00109 } 00110 static int32_t utest_us_ticker_run() 00111 { 00112 UTEST_LOG_FUNCTION(); 00113 while(1) 00114 { 00115 // check if a new callback has been set 00116 if (minimal_callback) 00117 { 00118 // copy the callback 00119 utest_v1_harness_callback_t callback = minimal_callback; 00120 // reset the shared callback 00121 minimal_callback = NULL; 00122 // execute the copied callback 00123 callback(); 00124 } 00125 } 00126 } 00127 00128 00129 extern "C" { 00130 static const utest_v1_scheduler_t utest_v1_scheduler = 00131 { 00132 utest_us_ticker_init, 00133 utest_us_ticker_post, 00134 utest_us_ticker_cancel, 00135 utest_us_ticker_run 00136 }; 00137 utest_v1_scheduler_t utest_v1_get_scheduler() 00138 { 00139 UTEST_LOG_FUNCTION(); 00140 return utest_v1_scheduler; 00141 } 00142 } 00143 #endif 00144 00145 #ifdef YOTTA_CORE_UTIL_VERSION_STRING 00146 // their functionality is implemented using the CriticalSectionLock class 00147 void utest_v1_enter_critical_section(void) {} 00148 void utest_v1_leave_critical_section(void) {} 00149 #endif
Generated on Tue Jul 12 2022 14:49:15 by
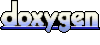