
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
utest_default_handlers.h
00001 /**************************************************************************** 00002 * Copyright (c) 2015, ARM Limited, All Rights Reserved 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00006 * not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 **************************************************************************** 00017 */ 00018 00019 #ifndef UTEST_DEFAULT_HANDLER_H 00020 #define UTEST_DEFAULT_HANDLER_H 00021 00022 #include <stdint.h> 00023 #include <stdbool.h> 00024 #include <stdio.h> 00025 #include "utest/utest_types.h" 00026 00027 00028 namespace utest { 00029 /** \addtogroup frameworks */ 00030 /** @{*/ 00031 namespace v1 { 00032 00033 /** Default handler hint. 00034 * 00035 * Use this handler to indicate the you want the default handler to be called. 00036 * This type automatically casts itself into the appropriate handler type, when possible. 00037 * Use the constants to default a handler unambigously. 00038 */ 00039 static const struct 00040 { 00041 ///@cond IGNORE 00042 // Doxygen can't parse these implicit conversion operators properly, remove from 00043 // doc build 00044 operator test_setup_handler_t() const { return test_setup_handler_t(1); } 00045 operator test_teardown_handler_t() const { return test_teardown_handler_t(1); } 00046 operator test_failure_handler_t() const { return test_failure_handler_t(1); } 00047 00048 operator case_setup_handler_t() const { return case_setup_handler_t(1); } 00049 operator case_teardown_handler_t() const { return case_teardown_handler_t(1); } 00050 operator case_failure_handler_t() const { return case_failure_handler_t(1); } 00051 ///@endcond 00052 } default_handler; 00053 00054 /** Ignore handler hint. 00055 * 00056 * Use this handler to indicate the you want to ignore this handler and it will not be called. 00057 * This type automatically casts itself into the appropriate handler type, when possible. 00058 * Use the constants to ignore a handler unambigously. 00059 */ 00060 static const struct 00061 { 00062 ///@cond IGNORE 00063 // Doxygen can't parse these implicit conversion operators properly, remove from 00064 // doc build 00065 operator case_handler_t() const { return case_handler_t(NULL); } 00066 operator case_control_handler_t() const { return case_control_handler_t(NULL); } 00067 operator case_call_count_handler_t() const { return case_call_count_handler_t(NULL); } 00068 00069 operator test_setup_handler_t() const { return test_setup_handler_t(NULL); } 00070 operator test_teardown_handler_t() const { return test_teardown_handler_t(NULL); } 00071 operator test_failure_handler_t() const { return test_failure_handler_t(NULL); } 00072 00073 operator case_setup_handler_t() const { return case_setup_handler_t(NULL); } 00074 operator case_teardown_handler_t() const { return case_teardown_handler_t(NULL); } 00075 operator case_failure_handler_t() const { return case_failure_handler_t(NULL); } 00076 ///@endcond 00077 } ignore_handler; 00078 00079 /** A table of handlers. 00080 * 00081 * This structure stores all modifyable handlers and provides accessors to 00082 * filter out the default handler. 00083 * So if this structure contains handlers, and you want to use these handlers 00084 * as a default backup, you can use the `get_handler` function to choose the right handler. 00085 * 00086 * Example: 00087 * @code 00088 * const handler_t defaults = { ... }; // your default handlers 00089 * // will return the handler in defaults. 00090 * test_setup_handler_t handler = defaults.get_handler(default_handler); 00091 * // you will still need to manually check the handler before executing it 00092 * if (handler != ignore_handler) handler(...); 00093 * 00094 * extern test_teardown_handler_t custom_handler(...); 00095 * // will return `custom_handler` 00096 * test_teardown_handler_t handler = defaults.get_handler(custom_handler); 00097 * // you will still need to manually check the handler before executing it 00098 * if (handler != ignore_handler) handler(...); 00099 * @endcode 00100 */ 00101 struct handlers_t 00102 { 00103 test_setup_handler_t test_setup; 00104 test_teardown_handler_t test_teardown; 00105 test_failure_handler_t test_failure; 00106 00107 case_setup_handler_t case_setup; 00108 case_teardown_handler_t case_teardown; 00109 case_failure_handler_t case_failure; 00110 00111 inline test_setup_handler_t get_handler(test_setup_handler_t handler) const { 00112 if (handler == default_handler) return test_setup; 00113 return handler; 00114 } 00115 inline test_teardown_handler_t get_handler(test_teardown_handler_t handler) const { 00116 if (handler == default_handler) return test_teardown; 00117 return handler; 00118 } 00119 inline test_failure_handler_t get_handler(test_failure_handler_t handler) const { 00120 if (handler == default_handler) return test_failure; 00121 return handler; 00122 } 00123 00124 inline case_setup_handler_t get_handler(case_setup_handler_t handler) const { 00125 if (handler == default_handler) return case_setup; 00126 return handler; 00127 } 00128 inline case_teardown_handler_t get_handler(case_teardown_handler_t handler) const { 00129 if (handler == default_handler) return case_teardown; 00130 return handler; 00131 } 00132 inline case_failure_handler_t get_handler(case_failure_handler_t handler) const { 00133 if (handler == default_handler) return case_failure; 00134 return handler; 00135 } 00136 }; 00137 00138 /// Prints the number of tests to run and continues. 00139 utest::v1::status_t verbose_test_setup_handler (const size_t number_of_cases); 00140 /// Prints the number of tests that passed and failed with a reason if provided. 00141 void verbose_test_teardown_handler(const size_t passed, const size_t failed, const failure_t failure); 00142 /// Prints the failure for `REASON_TEST_SETUP` and `REASON_TEST_TEARDOWN` and then dies. 00143 void verbose_test_failure_handler (const failure_t failure); 00144 00145 /// Prints the index and description of the case being run and continues. 00146 utest::v1::status_t verbose_case_setup_handler (const Case *const source, const size_t index_of_case); 00147 /// Prints the number of tests that passed and failed with a reason if provided within this case and continues. 00148 utest::v1::status_t verbose_case_teardown_handler(const Case *const source, const size_t passed, const size_t failed, const failure_t failure); 00149 /// Prints the reason of the failure and continues, unless the teardown handler failed, for which it aborts. 00150 utest::v1::status_t verbose_case_failure_handler (const Case *const source, const failure_t reason); 00151 00152 /// Default greentea test case set up handler 00153 #define UTEST_DEFAULT_GREENTEA_TIMEOUT 10 //Seconds 00154 #define UTEST_DEFAULT_HOST_TEST_NAME "default_auto" 00155 00156 utest::v1::status_t default_greentea_test_setup_handler (const size_t number_of_cases); 00157 00158 /// Requests the start test case from greentea and continues. 00159 /// Example usage: 00160 /// utest::v1::status_t greentea_test_setup(const size_t number_of_cases) { 00161 /// GREENTEA_SETUP(5, "default_auto"); 00162 /// return greentea_test_setup_handler(number_of_cases); 00163 /// } 00164 /// 00165 /// Specification specification(greentea_test_setup, cases, greentea_test_teardown_handler); 00166 utest::v1::status_t greentea_test_setup_handler (const size_t number_of_cases); 00167 00168 /// Reports the test results to greentea. 00169 void greentea_test_teardown_handler(const size_t passed, const size_t failed, const failure_t failure); 00170 /// Reports the failure for `REASON_TEST_SETUP` and `REASON_TEST_TEARDOWN` to greentea and then dies. 00171 void greentea_test_failure_handler (const failure_t failure); 00172 00173 /// Registers the test case setup with greentea. 00174 utest::v1::status_t greentea_case_setup_handler (const Case *const source, const size_t index_of_case); 00175 /// Registers the test case teardown with greentea. 00176 utest::v1::status_t greentea_case_teardown_handler(const Case *const source, const size_t passed, const size_t failed, const failure_t failure); 00177 /// Reports the failure to greentea and then aborts. 00178 utest::v1::status_t greentea_case_failure_abort_handler (const Case *const source, const failure_t reason); 00179 /// Reports the failure to greentea and then continues. 00180 utest::v1::status_t greentea_case_failure_continue_handler(const Case *const source, const failure_t reason); 00181 00182 /// Notify greentea of testcase name. 00183 void greentea_testcase_notification_handler(const char *testcase); 00184 00185 /// The verbose default handlers that always continue on failure 00186 extern const handlers_t verbose_continue_handlers; 00187 00188 /// The greentea default handlers that always abort on the first encountered failure 00189 extern const handlers_t greentea_abort_handlers; 00190 00191 /// The greentea default handlers that always continue on failure 00192 extern const handlers_t greentea_continue_handlers; 00193 00194 /// The selftest default handlers that always abort on _any_ assertion failure, otherwise continue 00195 extern const handlers_t selftest_handlers; 00196 00197 /// The greentea aborting handlers are the default 00198 extern const handlers_t& default_handlers; 00199 00200 } // namespace v1 00201 } // namespace utest 00202 00203 #endif // UTEST_DEFAULT_HANDLER_H 00204 00205 /** @}*/
Generated on Tue Jul 12 2022 14:49:14 by
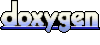