
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
thread_management_api.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2015-2016 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 00015 /** 00016 * \file thread_management_api.h 00017 * \brief Public API for managing Thread network configuration. 00018 * 00019 * This interface enables modification and reading the Thread network parameters. 00020 * 00021 * Thread management can modify the following values in the Thread network: 00022 * * [Network Name] (Read/write) 00023 * * [Security Policy] (Read/write) 00024 * * [Steering Data] (Read/write) 00025 * * [Commissioning Data Timestamp] (Read/write) 00026 * * [Commissioning Credential] PSKc (Write only) 00027 * * [Network Master Key] (Read only when policy allows) 00028 * * [Network Key Sequence] (Read only when policy allows) 00029 * * [Network Mesh-Local ULA] (Read only) 00030 * * [Border Router Locator] (Read only) 00031 * * [Commissioner Session ID] (Read only) 00032 * * [XPANID] (Read only) 00033 * * [PANID] (Read only) 00034 * * [Channel] (Read only) 00035 */ 00036 00037 #ifndef THREAD_MANAGEMENT_API_H_ 00038 #define THREAD_MANAGEMENT_API_H_ 00039 00040 #ifdef __cplusplus 00041 extern "C" { 00042 #endif 00043 00044 #include "ns_types.h" 00045 00046 #define TRACE_GROUP_THREAD_MANAGEMENT_API "TMaA" /**< trace group definition */ 00047 00048 /** Thread management state 00049 */ 00050 typedef enum { 00051 MANAGEMENT_STATE_REJECT, 00052 MANAGEMENT_STATE_PENDING, 00053 MANAGEMENT_STATE_ACCEPT 00054 } management_state_e; 00055 00056 /** \brief Register Thread management interface 00057 * 00058 * \param interface_id Interface ID of the Thread network. 00059 * 00060 * \return A handle for the management interface. 00061 * 00062 */ 00063 int thread_management_register(int8_t interface_id); 00064 00065 /** \brief Unregister Thread management interface 00066 * 00067 * \param instance_id ID of the management session. 00068 * 00069 */ 00070 int thread_management_unregister(int8_t instance_id); 00071 00072 /** \brief Callback to display the result of management set command. 00073 * 00074 * This callback is called when the server responds to the management set command. 00075 * This can fail if the leader rejects the request or the request times out. 00076 * 00077 * \param interface_id The interface ID of the Thread network. 00078 * \param status Result of the request. 00079 * 00080 */ 00081 typedef int (management_set_response_cb)(int8_t interface_id, management_state_e status); 00082 00083 /** \brief Set the Thread security policy 00084 * 00085 * \param instance_id The ID of the management session. 00086 * \param options Security policy options: 00087 * bit 8 Out-of-band commissioning restricted. 00088 * bit 7 Native commissioner restricted. 00089 * \param rotation_time Thread key rotation time in hours. 00090 * \param cb_ptr A callback function indicating the result of the operation. Can be NULL if no result code needed. 00091 * 00092 * \return 0 Success. 00093 * \return <0 Fail. 00094 */ 00095 int thread_management_set_security_policy(int8_t instance_id, uint8_t options, uint16_t rotation_time, management_set_response_cb *cb_ptr); 00096 00097 /** \brief Set the steering data 00098 * 00099 * Steering data can be either: 00100 * - Empty to decline joining. 00101 * - Any length with all bits set to 0 to decline joining. 00102 * - Any length with all bits 1 to allow anyone to join. 00103 * - Bloom filter to guide which device can join. 00104 * 00105 * If a Bloom filter is present it can be any length between 1-16 bytes. The first bit of the Bloom 00106 * filter indicates whether to use 0 == EUI-64 or 1 == bottom 24 bits of EUI-64. 00107 * 00108 * \param instance_id The ID of the management session. 00109 * \param session_id The commissioning session ID that needs to be added. 00110 * \param steering_data_ptr A pointer to new steering data. 00111 * \param steering_data_len The length of the new steering data. 00112 * \param cb_ptr A callback function indicating the result of the operation. Can be NULL if no result code needed. 00113 * 00114 * \return 0 Success. 00115 * \return <0 Fail. 00116 */ 00117 int thread_management_set_steering_data(int8_t instance_id, uint16_t session_id, uint8_t *steering_data_ptr, uint8_t steering_data_len, management_set_response_cb *cb_ptr); 00118 00119 /** \brief Set the Thread commissioning data timestamp 00120 * 00121 * \param instance_id The ID of the management session. 00122 * \param time Upper 48 bits is the timestamp in seconds since the start of unix time, lower 16 bits are fractional portion of time. If the last bit is set to 1, the commissioner has accurate time. 00123 * \param cb_ptr A callback function indicating the result of the operation. Can be NULL if no result code needed. 00124 * 00125 * \return 0 Success. 00126 * \return <0 Fail. 00127 */ 00128 int thread_management_set_commissioning_data_timestamp(int8_t instance_id, uint64_t time, management_set_response_cb *cb_ptr); 00129 00130 /** \brief Callback for reading Thread management information 00131 * 00132 * Result message containing the Thread management TLV message. 00133 * This message can be parsed with thread_meshcop_lib.h. 00134 * 00135 * \param instance_id The ID of the management session. 00136 * \param status Result of the request. 00137 * \param response_message_ptr A meshcop TLV structure pointer containing the requested TLVs. 00138 * \param response_message_len The length of the message. 00139 * 00140 * \return 0 Success. 00141 * \return <0 Fail. 00142 */ 00143 typedef int (management_get_response_cb)(int8_t instance_id, management_state_e status, uint8_t *response_message_ptr, uint16_t response_message_len); 00144 00145 /** \brief Get Thread management fields. 00146 * Fetching Thread management field values from any device in the Thread network. 00147 * 00148 * \param instance_id Instance ID of the management session. 00149 * \param dst_addr Destination address; the address of a remote device from whome it is desirable to fetch management information. If however, the address is not provided, a request is sent to the leader of the network for this purpose. If a native commissioner is used, the request for management information is sent to the border router. 00150 * \param uri_ptr The ASCII string for the URI. This string identifies the CoAP URI for the desired resource. For example, /c/mg identifies the management get information resource. 00151 * \param fields_ptr A pointer that points to an array of desirable MESHCOP TLVs. A list of such TLVs can be found in thread_meshcop_lib.h 00152 * \param fields_count Number of fields in the field pointer array (set of TLVs). 00153 * \param cb_ptr A callback function carrying the result of the operation. 00154 * 00155 * \return 0, Success. 00156 * \return <0 Fail. 00157 */ 00158 int thread_management_get(int8_t instance_id, uint8_t dst_addr[static 16], char *uri_ptr, uint8_t *fields_ptr, uint8_t fields_count, management_get_response_cb *cb_ptr); 00159 00160 /** \brief Set Thread management fields 00161 * 00162 * Set Thread management field values to a device in Thread network. 00163 * 00164 * \param instance_id Instance ID of the management session. 00165 * \param dst_addr Destination address, the address of a remote device where it is desired to setup management information. If however, the address is not provided, a request is sent to leader of the network for this purpose. If a native commissioner is being used, the rquest for setting up management information is sent to the Border router. 00166 * \param uri_ptr The ASCII string for the URI. This string identifies the CoAP URI for the desired resource, for example, /c/ms identifies the the management set information resource. 00167 * \param data_ptr A pointer to the desired set of TLVs. 00168 * \param data_len count of the members (no. of TLVs) in the TLV set. 00169 * \param cb_ptr A callback function carrying the result of the operation. 00170 * 00171 * \return 0, Success 00172 * \return <0 Fail. 00173 * 00174 */ 00175 int thread_management_set(int8_t instance_id, uint8_t dst_addr[static 16], char *uri_ptr, uint8_t *data_ptr, uint8_t data_len, management_set_response_cb *cb_ptr); 00176 00177 #ifdef __cplusplus 00178 } 00179 #endif 00180 #endif /* THREAD_MANAGEMENT_API_H_ */
Generated on Tue Jul 12 2022 14:49:05 by
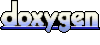